How to work with databases in Ruby?
Working with databases in Ruby is a fundamental skill for many developers, as databases are a core component of most applications for storing and retrieving data efficiently. Ruby provides a variety of libraries and frameworks to interact with databases, and one of the most commonly used options is ActiveRecord, which is part of the Ruby on Rails framework.
Here’s a general overview of how to work with databases in Ruby using ActiveRecord:
- Installation: First, you need to include the ActiveRecord gem in your project. You can do this by adding it to your Gemfile and running `bundle install` or by installing it manually using `gem install activerecord`.
- Configuration: Configure your database connection in a configuration file, typically `config/database.yml`, where you specify the database adapter (e.g., PostgreSQL, MySQL, SQLite), host, username, and password.
- Creating Models: In Ruby on Rails, models are Ruby classes that represent database tables. You create a model using Rails generators or by defining a class manually. Each model corresponds to a table in the database and inherits from `ActiveRecord::Base`.
- Migrations: Migrations are Ruby scripts that define changes to the database schema over time. You can create, modify, or remove database tables and columns using migrations. Running `rails db:migrate` applies these changes to the database.
- CRUD Operations: ActiveRecord provides methods for creating, reading, updating, and deleting records in the database. For example, you can use `Model.create`, `Model.find`, `Model.update`, and `Model.destroy` to perform these operations.
- Querying: You can use ActiveRecord’s query interface to retrieve specific data from the database. Methods like `where`, `order`, `limit`, and `joins` help you construct complex queries easily.
- Associations: ActiveRecord allows you to define associations between models, such as one-to-many or many-to-many relationships. This makes it straightforward to work with related data.
- Validation: You can define validation rules within your models to ensure that data meets specific criteria before it’s saved to the database. Common validations include presence, uniqueness, and format checks.
- Callbacks: Callbacks are hooks that allow you to trigger custom code at certain points in the object’s lifecycle, such as before or after saving to the database.
- Transactions: ActiveRecord supports transactions, which allow you to group multiple database operations into a single atomic unit. This ensures that either all operations succeed or none do.
- Error Handling: Proper error handling is crucial when working with databases. ActiveRecord provides error messages and exceptions to help you handle database-related errors gracefully.
- Testing: Writing tests for database operations is essential to ensure the reliability of your application. Frameworks like RSpec and Minitest are commonly used for testing in Ruby.
Working with databases in Ruby, especially within the Ruby on Rails framework, involves configuring your database connection, defining models, managing schema changes through migrations, and using ActiveRecord’s extensive API for database operations. This combination of tools and practices simplifies database interaction, making it a powerful and efficient process for Ruby developers
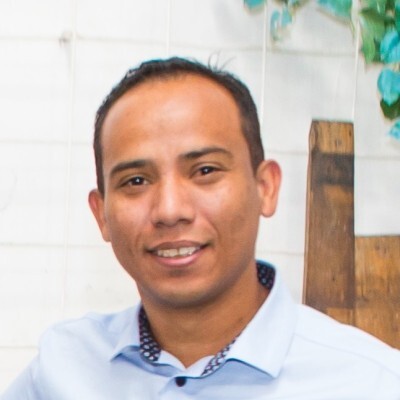
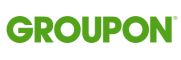