Working with Dates and Times in Ruby: From Simple Calculations to Time Zones
As a Ruby developer, you will inevitably encounter scenarios where handling dates and times becomes crucial. Whether you are building a scheduling application, processing time-sensitive data, or working on any project that requires tracking time, having a solid understanding of date and time manipulation in Ruby is essential.
Table of Contents
In this blog post, we will delve into the fascinating world of working with dates and times in Ruby. We will cover everything from basic calculations and formatting to more advanced topics like handling time zones effectively. By the end of this guide, you’ll be equipped with the knowledge and tools to handle any date and time-related challenges in your Ruby projects.
1. Understanding Ruby’s Time Class
In Ruby, the Time class is at the core of handling dates and times. Let’s start with the basics:
1.1. Creating Time objects
You can create a new Time object using the Time.new method or by directly specifying the year, month, day, hour, minute, and second.
ruby # Using Time.new time1 = Time.new(2023, 7, 17, 12, 30, 0) # Directly specifying components time2 = Time.new(year: 2023, month: 7, day: 17, hour: 12, min: 30, sec: 0)
1.2. Time arithmetic
Ruby allows you to perform arithmetic operations with Time objects, such as addition and subtraction.
ruby # Adding 1 day to a Time object next_day = time1 + (24 * 60 * 60) # Subtracting 2 hours from a Time object two_hours_earlier = time2 - (2 * 60 * 60)
1.3. Extracting components from Time objects
You can extract various components from a Time object, like the year, month, day, hour, minute, and second.
ruby year = time1.year month = time1.month day = time1.day hour = time1.hour minute = time1.min second = time1.sec
2. Formatting Dates and Times
When presenting dates and times to users or saving them in a specific format, formatting is crucial. Ruby’s strftime method comes to the rescue!
2.1. Using strftime method
The strftime method formats a Time object into a string based on directives.
ruby formatted_time = time1.strftime("%Y-%m-%d %H:%M:%S") puts formatted_time #=> "2023-07-17 12:30:00"
2.2. Common formatting directives
Here are some common formatting directives:
- %Y: Year with century as a decimal number.
- %m: Month as a zero-padded decimal number.
- %d: Day of the month as a zero-padded decimal number.
- %H: Hour (24-hour clock) as a zero-padded decimal number.
- %M: Minute as a zero-padded decimal number.
- %S: Second as a zero-padded decimal number.
3. Parsing Dates and Times
Working with user input or external data often requires parsing strings into Time objects. Ruby’s strptime method is perfect for this.
3.1. Using strptime method
The strptime method parses a string according to a specified format and returns a Time object.
ruby require 'time' parsed_time = Time.strptime("2023-07-17 12:30:00", "%Y-%m-%d %H:%M:%S")
3.2. Dealing with different date formats
When parsing dates from different sources, you may encounter various formats. Be sure to adjust the format string accordingly.
ruby date_format_1 = "%m/%d/%Y" date_format_2 = "%d-%m-%Y"
4. Working with Time Zones
Handling time zones correctly is critical when dealing with global applications or events across different regions. Ruby provides the TZInfo and ActiveSupport libraries to manage time zones effectively.
4.1. Time zones and offsets
Time zones are regions with the same standard time. Each time zone has a UTC offset, which represents the time difference from Coordinated Universal Time (UTC). Ruby’s Time objects are automatically represented in UTC, and you can convert them to a specific time zone using TZInfo.
ruby require 'tzinfo' # Get a time zone instance timezone = TZInfo::Timezone.get('America/New_York') # Convert a Time object to the specified time zone time_in_new_york = timezone.utc_to_local(time1)
4.2. Converting between time zones
You can convert a Time object from one time zone to another by first converting it to UTC and then to the desired time zone.
ruby # Convert a Time object from New York time to Sydney time time_in_sydney = timezone.local_to_utc(time_in_new_york) timezone_sydney = TZInfo::Timezone.get('Australia/Sydney') time_in_sydney = timezone_sydney.utc_to_local(time_in_sydney)
4.3. Dealing with Daylight Saving Time
Daylight Saving Time (DST) is a practice of setting the clock forward by one hour during warmer months to extend evening daylight. Ruby’s Time class automatically accounts for DST changes when converting between time zones.
5. Handling Date and Time Differences
Calculating time duration or finding the elapsed time between dates is common in many applications. Ruby provides simple methods to handle these scenarios.
5.1. Calculating time duration
You can calculate the duration between two Time objects using simple subtraction.
ruby start_time = Time.new(2023, 7, 17, 10, 0, 0) end_time = Time.new(2023, 7, 17, 12, 30, 0) duration = end_time - start_time puts duration #=> 9000.0 (seconds)
5.2. Elapsed time between dates
To find the time elapsed between two dates, you can convert the dates to Time objects and perform the same subtraction.
ruby require 'date' start_date = Date.new(2023, 7, 17) end_date = Date.new(2023, 7, 20) duration_days = (end_date - start_date).to_i puts duration_days #=> 3
6. Dealing with Recurring Dates
Some applications require handling recurring dates, like weekly meetings or monthly subscriptions. Ruby’s Date and DateTime classes provide useful functionalities for such scenarios.
6.1. Using the Date and DateTime classes
The Date class allows you to work with dates (year, month, day) without considering time.
ruby require 'date' # Find the next occurrence of a specific day (e.g., Monday) next_monday = Date.today.next_day((1 - Date.today.wday) % 7)
The DateTime class extends Date to include time (hour, minute, second).
ruby require 'date' # Create a DateTime object with specific date and time datetime = DateTime.new(2023, 7, 17, 12, 30, 0)
6.2. Finding recurring patterns
To handle recurring events efficiently, you can use libraries like ice_cube, which provides advanced recurring date and time calculations.
7. Handling Time Intervals
Ruby’s Interval class (part of the date_interval gem) simplifies working with time intervals and performing operations on them.
7.1. Using the Interval class
First, install the date_interval gem.
ruby gem install date_interval
Then, you can create intervals and perform various operations.
ruby require 'date_interval' interval = DateInterval::Interval.new("1 year 6 months") future_date = interval.from(Date.today) puts future_date #=> #<Date: 2024-01-17 ((2459805j,0s,0n),+0s,2299161j)>
8. Working with ActiveSupport (Optional)
ActiveSupport is a powerful gem that extends Ruby’s date and time capabilities, offering additional features and enhancements.
8.1. Overview of ActiveSupport’s date and time support
ActiveSupport provides a wide range of helpful methods for date and time manipulation.
ruby require 'active_support/all' # Add 2 hours to a Time object time = Time.now new_time = time + 2.hours
8.2. Notable features and extensions
Some notable features include duration calculation, time zone support, and various date and time extensions.
Conclusion
Working with dates and times in Ruby is a fundamental skill for any developer. From simple calculations to dealing with time zones, this guide covered the essential aspects of handling date and time-related challenges. Armed with this knowledge, you can confidently build applications that accurately manage and manipulate dates and times, ensuring the success of your projects no matter where they take place.
So, go ahead and apply these techniques in your Ruby projects. Embrace the power of dates and times in your code and unlock a world of possibilities! Happy coding!
Table of Contents
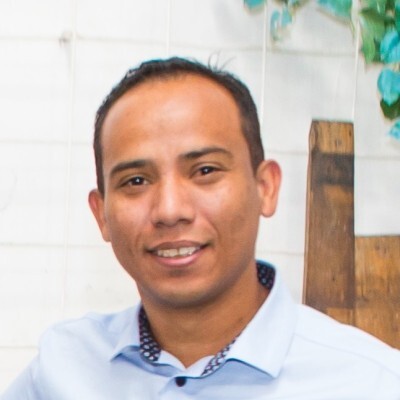
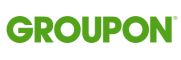