How to use ‘yield’ in Ruby?
In Ruby, the `yield` keyword is a powerful and flexible way to work with blocks of code. It allows you to pass control from a method to a block and back, enabling you to inject custom behavior into a method’s execution.
Here’s how you can use `yield` in Ruby:
- Defining a Method with `yield`:
You can define a method that includes a `yield` statement within its body. When the method is called and encounters the `yield` keyword, it temporarily hands control over to the block of code passed to the method. Any code within the block will be executed.
```ruby def greet puts "Hello," yield puts "Goodbye!" end greet { puts "Ruby" } ```
When you call `greet` with a block, it will yield control to the block, resulting in the following output:
``` Hello, Ruby Goodbye! ```
- Passing Parameters to the Block:
You can also pass parameters to the block when using `yield`. These parameters can be utilized within the block to customize its behavior based on the context in which it is called.
```ruby def greet(name) puts "Hello, #{name}" yield(name) puts "Goodbye!" end greet("Alice") { |name| puts "Nice to meet you, #{name}" } ```
In this example, the block takes the `name` parameter passed by `yield` and incorporates it into its message.
- Handling Block Existence:
You can check if a block was passed to a method using the `block_given?` method. This allows you to conditionally execute code based on the presence of a block.
```ruby def with_block puts "Before block execution" yield if block_given? puts "After block execution" end with_block { puts "Inside the block" } ```
The code within the `yield if block_given?` statement will only execute if a block is passed to the `with_block` method.
Using `yield` in Ruby is a powerful technique for creating methods that can adapt their behavior based on custom code provided by the caller. It’s commonly used in various Ruby libraries and frameworks to create flexible and extensible APIs.
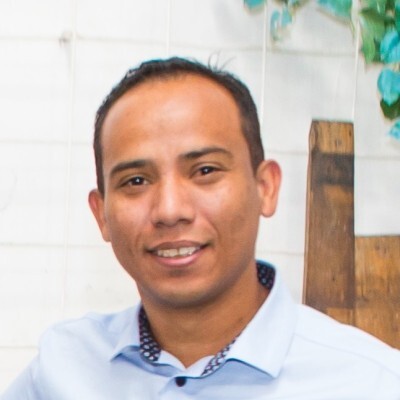
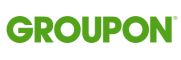