Shopify API Integration: Connecting Your Store with External Services
Shopify is a powerful platform for e-commerce, but its true potential is unlocked when integrated with external services through APIs. Whether you need to connect with payment gateways, inventory management systems, or marketing tools, API integration allows you to extend Shopify’s capabilities to meet your business needs.
This article will guide you through the process of integrating your Shopify store with external services using APIs. We’ll cover the basics of Shopify API, provide practical code examples, and explain how to authenticate, retrieve, and manipulate data to build custom integrations.
Understanding Shopify API
The Shopify API is a set of web services that allow developers to access and manipulate data within a Shopify store. With the API, you can:
– Manage products, orders, and customers
– Handle payments and refunds
– Access analytics and reports
– Integrate with external services
Shopify offers both REST and GraphQL APIs, providing flexibility depending on your needs.
1. Authenticating API Requests
Before you can interact with Shopify’s API, you need to authenticate your requests. Shopify uses OAuth2 for authentication, allowing your app to securely access a store’s data.
Example: Authenticating a Shopify API Request
Here’s a simple example of how to authenticate and make an API call using C.
```csharp using System; using System.Net.Http; using System.Net.Http.Headers; using System.Threading.Tasks; class ShopifyApi { private static readonly string ApiKey = "your_api_key"; private static readonly string Password = "your_api_password"; private static readonly string StoreUrl = "yourstore.myshopify.com"; static async Task Main() { using var client = new HttpClient(); var byteArray = new System.Text.UTF8Encoding().GetBytes($"{ApiKey}:{Password}"); client.DefaultRequestHeaders.Authorization = new AuthenticationHeaderValue("Basic", Convert.ToBase64String(byteArray)); var response = await client.GetStringAsync($"https://{StoreUrl}/admin/api/2023-04/orders.json"); Console.WriteLine(response); } } ```
2. Retrieving Data from Shopify
Once authenticated, you can retrieve data from Shopify. This could include fetching products, orders, customers, or any other resource available through the API.
Example: Fetching Products from Shopify
Here’s an example of how to retrieve a list of products from your Shopify store using C.
```csharp using System; using System.Net.Http; using System.Threading.Tasks; class ShopifyApi { private static readonly string ApiKey = "your_api_key"; private static readonly string Password = "your_api_password"; private static readonly string StoreUrl = "yourstore.myshopify.com"; static async Task Main() { using var client = new HttpClient(); var byteArray = new System.Text.UTF8Encoding().GetBytes($"{ApiKey}:{Password}"); client.DefaultRequestHeaders.Authorization = new System.Net.Http.Headers.AuthenticationHeaderValue("Basic", Convert.ToBase64String(byteArray)); var response = await client.GetStringAsync($"https://{StoreUrl}/admin/api/2023-04/products.json"); Console.WriteLine(response); } } ```
3. Manipulating Data in Shopify
In addition to retrieving data, you may need to create, update, or delete resources in your Shopify store. The Shopify API supports these operations, allowing you to automate tasks or integrate with external services.
Example: Creating a New Product in Shopify
Here’s an example of how to create a new product in your Shopify store using C.
```csharp using System; using System.Net.Http; using System.Text; using System.Threading.Tasks; class ShopifyApi { private static readonly string ApiKey = "your_api_key"; private static readonly string Password = "your_api_password"; private static readonly string StoreUrl = "yourstore.myshopify.com"; static async Task Main() { using var client = new HttpClient(); var byteArray = new System.Text.UTF8Encoding().GetBytes($"{ApiKey}:{Password}"); client.DefaultRequestHeaders.Authorization = new System.Net.Http.Headers.AuthenticationHeaderValue("Basic", Convert.ToBase64String(byteArray)); var newProductJson = @" { ""product"": { ""title"": ""New Product"", ""body_html"": ""<strong>Great new product!</strong>"", ""vendor"": ""Your Vendor"", ""product_type"": ""New Type"", ""variants"": [ { ""price"": ""19.99"", ""sku"": ""new-product-sku"" } ] } }"; var content = new StringContent(newProductJson, Encoding.UTF8, "application/json"); var response = await client.PostAsync($"https://{StoreUrl}/admin/api/2023-04/products.json", content); Console.WriteLine(await response.Content.ReadAsStringAsync()); } } ```
4. Integrating with External Services
Shopify’s API makes it easy to connect your store with external services. Whether you’re integrating with a CRM, payment gateway, or email marketing service, the API allows seamless communication between Shopify and other platforms.
Example: Integrating Shopify with an Email Marketing Service
Imagine you want to add customers who make a purchase to your email marketing list. You can use Shopify’s API to retrieve customer data and an external service’s API to add them to your list.
```csharp using System; using System.Net.Http; using System.Text; using System.Threading.Tasks; class ShopifyEmailIntegration { private static readonly string ApiKey = "your_api_key"; private static readonly string Password = "your_api_password"; private static readonly string StoreUrl = "yourstore.myshopify.com"; private static readonly string EmailServiceApiUrl = "https://api.emailservice.com/add"; static async Task Main() { using var client = new HttpClient(); var byteArray = new System.Text.UTF8Encoding().GetBytes($"{ApiKey}:{Password}"); client.DefaultRequestHeaders.Authorization = new System.Net.Http.Headers.AuthenticationHeaderValue("Basic", Convert.ToBase64String(byteArray)); // Fetch customers var customersJson = await client.GetStringAsync($"https://{StoreUrl}/admin/api/2023-04/customers.json"); // Send to email service var content = new StringContent(customersJson, Encoding.UTF8, "application/json"); var response = await client.PostAsync(EmailServiceApiUrl, content); Console.WriteLine(await response.Content.ReadAsStringAsync()); } } ```
Conclusion
Integrating Shopify with external services through API opens up endless possibilities for customizing and enhancing your e-commerce store. Whether you need to automate workflows, connect with third-party services, or build custom applications, the Shopify API provides the tools you need to achieve your goals.
By following the examples provided in this article, you can begin integrating your Shopify store with the external services that matter most to your business, driving efficiency and growth.
Further Reading:
Table of Contents
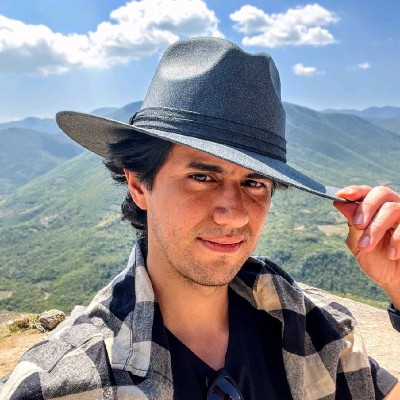
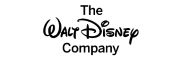