Shopify App Development: Leveraging Shopify POS for Offline Sales
In today’s retail landscape, omnichannel experiences are crucial for success. Shopify’s Point of Sale (POS) system allows merchants to sell in physical stores while maintaining seamless integration with their online Shopify stores. For developers, integrating Shopify POS into apps presents an opportunity to enhance the shopping experience by bridging online and offline sales. This article explores how to leverage Shopify POS for offline sales in app development, with practical examples and best practices.
Understanding Shopify POS
Shopify POS is a powerful tool that enables merchants to conduct sales in physical locations while syncing with their Shopify online store. It allows for inventory management, customer tracking, and seamless transactions, all within the Shopify ecosystem. Integrating Shopify POS into your app can help merchants manage their business more efficiently by unifying their online and offline sales channels.
1. Getting Started with Shopify POS Integration
To start building apps that leverage Shopify POS, you’ll need to familiarize yourself with Shopify’s API and SDKs, which provide the necessary tools to interact with the POS system.
Example: Setting Up the Shopify POS SDK
To integrate Shopify POS into your app, you’ll need to install and configure the Shopify POS SDK. This SDK allows your app to communicate with the POS hardware and process transactions.
```javascript import ShopifyPOS from '@shopify/shopify-pos-js'; const shopifyPOS = new ShopifyPOS({ shopDomain: 'your-shop-name.myshopify.com', apiKey: 'your-api-key', accessToken: 'your-access-token' }); // Example function to start a transaction function startTransaction() { shopifyPOS.startTransaction() .then(response => console.log('Transaction started:', response)) .catch(error => console.error('Error starting transaction:', error)); } ```
2. Managing Inventory Across Channels
A key feature of Shopify POS is its ability to sync inventory between online and offline stores. This ensures that inventory levels are accurate, whether a product is sold in-store or online.
Example: Synchronizing Inventory with Shopify POS
Using Shopify’s Inventory API, you can synchronize inventory levels between online and offline channels. Here’s a sample code snippet:
```javascript import axios from 'axios'; async function syncInventory(productId, locationId, quantity) { const response = await axios.post(`https://your-shop-name.myshopify.com/admin/api/2023-07/inventory_levels/adjust.json`, { inventory_item_id: productId, location_id: locationId, available_adjustment: quantity }); console.log('Inventory synchronized:', response.data); } ```
3. Processing Offline Transactions
Offline transactions are essential for physical stores. With Shopify POS, you can process payments, issue receipts, and manage refunds, all while syncing data with the Shopify backend.
Example: Handling Payment Processing with Shopify POS
Here’s an example of how you can process a payment using Shopify POS:
```javascript import ShopifyPOS from '@shopify/shopify-pos-js'; function processPayment() { const paymentData = { amount: 1000, // Amount in cents currency: 'USD', paymentType: 'credit_card' }; shopifyPOS.processPayment(paymentData) .then(receipt => console.log('Payment processed, receipt:', receipt)) .catch(error => console.error('Error processing payment:', error)); } ```
4. Enhancing Customer Experience
Shopify POS allows for customer data collection and loyalty programs, which can be integrated into your app to enhance the customer experience. By leveraging customer data, merchants can provide personalized service and build stronger relationships with their clients.
Example: Integrating Customer Data
You can use the Shopify API to access and manage customer data, enabling personalized experiences in-store.
```javascript import axios from 'axios'; async function fetchCustomerData(customerId) { const response = await axios.get(`https://your-shop-name.myshopify.com/admin/api/2023-07/customers/${customerId}.json`); const customer = response.data.customer; console.log('Customer data:', customer); } ```
Conclusion
Leveraging Shopify POS for offline sales can significantly enhance a merchant’s ability to operate seamlessly across online and physical channels. By integrating Shopify POS into your app development, you can provide merchants with a powerful tool to manage inventory, process transactions, and improve customer experience. With the examples and best practices outlined in this article, you’ll be well on your way to building Shopify apps that empower merchants to succeed in an omnichannel retail environment.
Further Reading:
Table of Contents
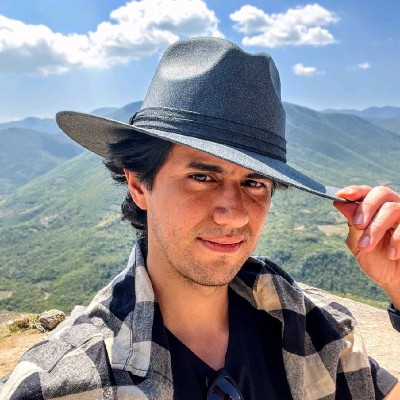
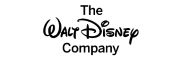