Shopify Script Editor: Implementing Dynamic Discounts and Promotions
Introduction to Shopify Script Editor
Shopify Script Editor is a powerful tool that allows store owners to create custom scripts that run on their Shopify store. These scripts can be used to create personalized shopping experiences, including dynamic discounts, custom promotions, and other advanced pricing strategies. By leveraging Shopify’s Ruby-based Script Editor, you can significantly enhance the flexibility and effectiveness of your store’s promotional activities.
Understanding Shopify Scripts
Shopify Scripts are small pieces of code that run in your store’s backend, allowing you to apply custom logic to checkout, shipping, and payment processes. Scripts are written in Ruby, and the Script Editor provides an environment to write, test, and implement these scripts directly in your Shopify store.
Getting Started with Shopify Script Editor
To begin using Shopify Script Editor, you’ll need to ensure your store is on the Shopify Plus plan, as the Script Editor is exclusive to this tier. Once enabled, you can start creating scripts to implement dynamic discounts and promotions.
1. Creating a Percentage-Based Discount Script
One of the most common promotions is offering percentage-based discounts. With the Script Editor, you can create a script that applies a percentage discount based on specific conditions, such as cart value or the number of items in the cart.
Example: Applying a 10% Discount on Orders Over $100
```ruby class PercentageDiscount < Script def initialize @discount_threshold = Money.new(cents: 10000) $100 threshold @discount_percentage = 10 10% discount end def run(cart) return unless cart.subtotal_price >= @discount_threshold discount = cart.subtotal_price @discount_percentage / 100 cart.discount_code("PERCENTAGE10") do |discount_code| discount_code.description = "10% off on orders over $100" discount_code.value = discount end end end PercentageDiscount.new.run(Input.cart) ```
2. Implementing a Buy-One-Get-One (BOGO) Promotion
BOGO promotions are a great way to incentivize customers to purchase more products. Using Shopify Scripts, you can create a BOGO promotion where customers receive a free or discounted item when they purchase a specific product.
Example: Buy One, Get One 50% Off
```ruby class BogoDiscount < Script def run(cart) eligible_items = cart.line_items.select do |line_item| line_item.product.id == 1234567890 Replace with your product ID end eligible_items.each do |item| next if item.quantity < 2 discount_item = item.split(quantity: item.quantity / 2) discount_item.change_line_price(discount_item.line_price 0.5, message: "BOGO 50% Off") cart.line_items << discount_item end end end BogoDiscount.new.run(Input.cart) ```
3. Creating Tiered Pricing Discounts
Tiered pricing allows you to offer discounts based on the quantity of items purchased. This strategy can encourage customers to buy more by offering greater discounts at higher quantities.
Example: Tiered Discounts for Bulk Purchases
```ruby class TieredDiscount < Script def run(cart) cart.line_items.each do |item| if item.quantity >= 10 item.change_line_price(item.line_price 0.8, message: "20% off for 10+ items") elsif item.quantity >= 5 item.change_line_price(item.line_price 0.9, message: "10% off for 5+ items") end end end end TieredDiscount.new.run(Input.cart) ```
4. Combining Multiple Discounts
Sometimes, you may want to combine multiple discounts into a single promotion. With Shopify Scripts, you can easily implement logic that applies different discounts based on various conditions.
Example: Combining Percentage Discount with Free Shipping
```ruby class CombinedDiscount < Script def run(cart) apply_percentage_discount(cart) apply_free_shipping(cart) end def apply_percentage_discount(cart) discount_threshold = Money.new(cents: 5000) $50 threshold discount_percentage = 15 15% discount return unless cart.subtotal_price >= discount_threshold cart.discount_code("15PERCENT") do |discount_code| discount_code.description = "15% off on orders over $50" discount_code.value = cart.subtotal_price discount_percentage / 100 end end def apply_free_shipping(cart) free_shipping_threshold = Money.new(cents: 10000) $100 threshold return unless cart.subtotal_price >= free_shipping_threshold cart.shipping_methods.each do |shipping_method| shipping_method.change_final_price(0, message: "Free Shipping") end end end CombinedDiscount.new.run(Input.cart) ```
Conclusion
The Shopify Script Editor is a powerful tool that allows you to implement advanced pricing strategies tailored to your store’s unique needs. By using the Script Editor, you can create dynamic discounts and promotions that enhance customer engagement and increase sales. Whether it’s simple percentage discounts or complex multi-tiered promotions, Shopify Scripts give you the flexibility to create a shopping experience that resonates with your audience.
Further Reading:
Table of Contents
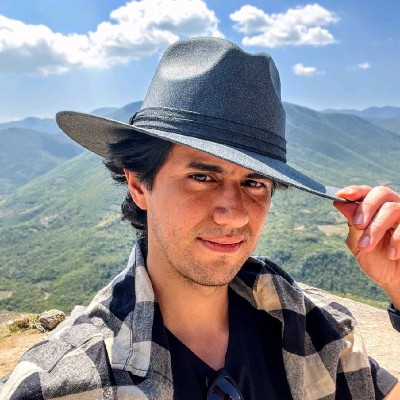
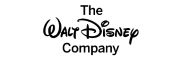