Mastering Shopify’s Webhooks: Building Real-time Integrations
Webhooks are a powerful feature in Shopify that allow you to automate workflows and build real-time integrations between your Shopify store and other services. By triggering HTTP callbacks when specific events occur in your store, Webhooks enable you to respond instantly to changes, enhancing the efficiency and automation of your e-commerce operations. This article will guide you through mastering Shopify’s Webhooks, from setting them up to integrating them with external systems.
Understanding Shopify Webhooks
Shopify Webhooks are HTTP POST requests sent by Shopify to a specified URL when certain events happen in your store. These events can include order creation, product updates, customer account changes, and more. Webhooks are essential for creating real-time integrations, ensuring that external systems are immediately notified of any changes in your store.
Setting Up Shopify Webhooks
To start using Webhooks in Shopify, you need to configure them in the Shopify Admin interface or via the Shopify API. Below, we’ll cover both methods.
1. Creating Webhooks via Shopify Admin
Creating Webhooks through the Shopify Admin interface is straightforward. Follow these steps:
- Navigate to the Notifications Settings: Go to your Shopify Admin, click on Settings > Notifications.
- Create a New Webhook: Scroll down to the Webhooks section and click Create a webhook.
- Select the Event: Choose the event you want the Webhook to listen to, such as `Order creation` or `Product update`.
- Specify the URL: Enter the URL where Shopify will send the Webhook data.
- Save: Click Save webhook.
2. Creating Webhooks via Shopify API
Alternatively, you can create Webhooks programmatically using the Shopify API. This method is more flexible and allows for dynamic Webhook management.
Example: Creating a Webhook using Shopify API with Python
```python import requests SHOPIFY_API_KEY = 'your_api_key' SHOPIFY_PASSWORD = 'your_password' SHOPIFY_STORE_NAME = 'your_store_name' WEBHOOK_URL = 'https://your-server.com/webhook-handler' data = { "webhook": { "topic": "orders/create", "address": WEBHOOK_URL, "format": "json" } } response = requests.post( f'https://{SHOPIFY_API_KEY}:{SHOPIFY_PASSWORD}@{SHOPIFY_STORE_NAME}.myshopify.com/admin/api/2023-07/webhooks.json', json=data ) if response.status_code == 201: print("Webhook created successfully") else: print("Failed to create webhook", response.content) ```
Handling Webhook Data
Once a Webhook is triggered, Shopify sends a POST request with the event data to the specified URL. To process this data, you need to set up a server-side endpoint that can handle the incoming request.
Example: Handling a Webhook in Node.js
```javascript const express = require('express'); const bodyParser = require('body-parser'); const app = express(); app.use(bodyParser.json()); app.post('/webhook-handler', (req, res) => { const webhookData = req.body; console.log('Received webhook:', webhookData); // Process the webhook data (e.g., store in database, trigger other actions) res.status(200).send('Webhook received successfully'); }); app.listen(3000, () => { console.log('Server is running on port 3000'); }); ```
Securing Webhooks
Security is crucial when working with Webhooks to prevent unauthorized access and ensure data integrity.
1. Verifying Webhook Integrity
Shopify sends an HMAC-SHA256 hash of the Webhook data in the `X-Shopify-Hmac-Sha256` header. You can use this to verify that the Webhook data has not been tampered with.
Example: Verifying Webhook Integrity in Node.js
```javascript const crypto = require('crypto'); function verifyWebhook(req, secret) { const hmac = req.headers['x-shopify-hmac-sha256']; const generatedHmac = crypto.createHmac('sha256', secret) .update(req.rawBody, 'utf8') .digest('base64'); return crypto.timingSafeEqual(Buffer.from(hmac), Buffer.from(generatedHmac)); } ```
2. Using HTTPS
Always use HTTPS for your Webhook URLs to encrypt the data being sent, ensuring that sensitive information is protected during transmission.
Practical Use Cases of Shopify Webhooks
Shopify Webhooks can be utilized in various ways to enhance your store’s functionality. Here are some practical examples:
1. Inventory Management
Automatically sync inventory levels between your Shopify store and your warehouse management system by triggering Webhooks on product updates.
2. Order Processing
Trigger Webhooks on order creation to automatically send order details to your fulfillment service or CRM system.
3. Customer Notifications
Use Webhooks to notify customers or your support team when specific events occur, such as when an order is fulfilled or a payment fails.
Conclusion
Shopify Webhooks are a powerful tool for creating real-time integrations that can significantly enhance the automation and efficiency of your e-commerce operations. By mastering the setup, handling, and security of Webhooks, you can unlock new possibilities for your Shopify store, enabling seamless communication between your store and external systems. Leveraging Webhooks effectively can lead to a more responsive, dynamic, and efficient online store.
Further Reading:
Table of Contents
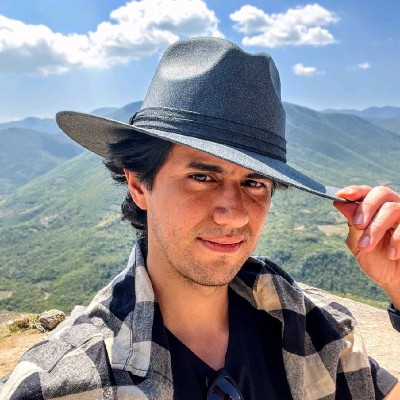
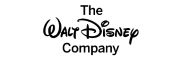