Swift and Core Graphics: Advanced Drawing and Animation in iOS
In iOS development, Core Graphics provides powerful tools for 2D drawing and animations. Swift, Apple’s modern programming language, enhances these capabilities, allowing developers to create rich and interactive user interfaces. This blog explores how Swift and Core Graphics can be used to craft advanced drawing and animation effects in iOS applications, providing practical examples and insights.
Understanding Core Graphics
Core Graphics, also known as Quartz 2D, is a framework that allows developers to draw 2D graphics and handle image processing tasks. It provides a rich set of functions for rendering shapes, text, and images, making it a valuable tool for creating custom graphics and animations.
Using Swift for Core Graphics
Swift’s strong type system and performance-oriented features make it an ideal language for working with Core Graphics. Below are some key aspects and code examples demonstrating how to use Swift with Core Graphics for advanced drawing and animation.
1. Drawing Custom Shapes
Custom shapes can be drawn using Core Graphics’ drawing functions. Swift’s syntax and Core Graphics’ APIs make it easy to create complex shapes.
Example: Drawing a Custom Shape
Here’s how to draw a custom shape (a star) using Core Graphics in Swift.
```swift import UIKit class StarView: UIView { override func draw(_ rect: CGRect) { guard let context = UIGraphicsGetCurrentContext() else { return } context.setFillColor(UIColor.yellow.cgColor) let starPath = UIBezierPath() let center = CGPoint(x: bounds.width / 2, y: bounds.height / 2) let radius: CGFloat = min(bounds.width, bounds.height) / 2 for i in 0..<5 { let angle = CGFloat(Double(i) * (2.0 * .pi / 5.0)) let x = center.x + radius * cos(angle) let y = center.y + radius * sin(angle) let point = CGPoint(x: x, y: y) if i == 0 { starPath.move(to: point) } else { starPath.addLine(to: point) } } starPath.close() context.addPath(starPath.cgPath) context.drawPath(using: .fill) } } ```
2. Animating Graphics
Animating graphics can enhance the user experience by making interfaces more dynamic and engaging. Core Animation works alongside Core Graphics to provide smooth animations.
Example: Basic Animation
This example demonstrates a simple animation that moves a view across the screen.
```swift import UIKit class AnimatedCircleView: UIView { override func draw(_ rect: CGRect) { guard let context = UIGraphicsGetCurrentContext() else { return } context.setFillColor(UIColor.blue.cgColor) context.addEllipse(in: bounds) context.drawPath(using: .fill) } func animateMovement() { let animation = CABasicAnimation(keyPath: "position") animation.fromValue = NSValue(cgPoint: center) animation.toValue = NSValue(cgPoint: CGPoint(x: center.x + 100, y: center.y)) animation.duration = 2.0 layer.add(animation, forKey: "positionAnimation") center.x += 100 } } ```
3. Creating Interactive Animations
Interactive animations respond to user gestures, enhancing the app’s responsiveness and engagement.
Example: Bounce Animation on Tap
Here’s how to create a bounce effect when a view is tapped.
```swift import UIKit class BounceView: UIView { override func draw(_ rect: CGRect) { guard let context = UIGraphicsGetCurrentContext() else { return } context.setFillColor(UIColor.red.cgColor) context.addEllipse(in: bounds) context.drawPath(using: .fill) } @objc func handleTap() { let bounceAnimation = CAKeyframeAnimation(keyPath: "transform.scale") bounceAnimation.values = [1.0, 1.2, 0.8, 1.0] bounceAnimation.duration = 0.5 layer.add(bounceAnimation, forKey: "bounceAnimation") } override init(frame: CGRect) { super.init(frame: frame) let tapGesture = UITapGestureRecognizer(target: self, action: #selector(handleTap)) addGestureRecognizer(tapGesture) } required init?(coder: NSCoder) { fatalError("init(coder:) has not been implemented") } } ```
4. Combining Core Graphics with Core Animation
Combining Core Graphics and Core Animation allows for complex visual effects that are both performant and engaging.
Example: Animated Chart
Here’s a basic example of animating a chart using Core Graphics and Core Animation.
```swift import UIKit class AnimatedChartView: UIView { override func draw(_ rect: CGRect) { guard let context = UIGraphicsGetCurrentContext() else { return } context.setFillColor(UIColor.green.cgColor) let chartPath = UIBezierPath() // Sample data points let dataPoints = [10, 20, 15, 30, 25] let width = bounds.width / CGFloat(dataPoints.count) for (index, value) in dataPoints.enumerated() { let x = CGFloat(index) * width let y = bounds.height - CGFloat(value) let rect = CGRect(x: x, y: y, width: width, height: CGFloat(value)) chartPath.addRect(rect) } context.addPath(chartPath.cgPath) context.drawPath(using: .fill) } func animateChart() { let animation = CABasicAnimation(keyPath: "bounds.size.height") animation.fromValue = NSNumber(value: 0) animation.toValue = NSNumber(value: bounds.height) animation.duration = 1.0 layer.add(animation, forKey: "chartAnimation") } } ```
Conclusion
Swift, combined with Core Graphics, provides powerful tools for creating advanced drawing and animation effects in iOS applications. Whether you’re drawing custom shapes, animating views, or integrating interactive animations, leveraging these capabilities will enhance the visual appeal and functionality of your apps. By mastering these techniques, you can build more engaging and dynamic user interfaces.
Further Reading:
Table of Contents
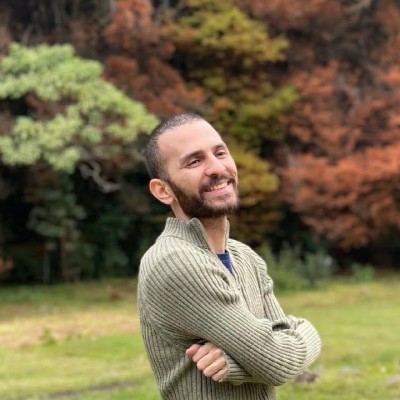
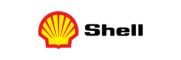