Xamarin.Android Development: Best Practices
Xamarin.Android is a powerful framework that allows developers to build native Android apps using C# and the .NET framework. It offers a rich set of tools and libraries, enabling developers to create high-quality and performant applications. However, like any development platform, there are certain best practices that can help streamline the development process and ensure the creation of robust and maintainable apps. In this blog post, we will delve into some essential best practices for Xamarin.Android development, providing insights, tips, and code samples to help you build exceptional apps.
Understand the Android Platform
To build successful Xamarin.Android apps, it’s crucial to have a solid understanding of the Android platform. Familiarize yourself with the Android lifecycle, UI components, and various device configurations. This knowledge will allow you to leverage platform-specific features effectively and deliver a seamless user experience.
Utilize Xamarin.Forms for UI Design
Xamarin.Forms is a UI toolkit that allows developers to create a single, shared UI codebase across multiple platforms, including Android. By leveraging Xamarin.Forms, you can write UI code once and have it adapt to different screen sizes and resolutions. This approach simplifies maintenance and speeds up development while providing a consistent user experience across platforms.
Optimize App Performance
Android devices come in various configurations, and it’s crucial to optimize your Xamarin.Android app for performance across a wide range of devices. Consider the following practices to ensure optimal performance:
- Use asynchronous programming techniques: Utilize async/await to perform time-consuming tasks in the background, keeping the UI responsive.
- Implement lazy loading: Load data and resources on-demand to reduce startup time and memory usage.
- Employ memory management techniques: Dispose of unused resources, use object pooling, and avoid excessive memory allocations.
- Optimize network requests: Use caching, compress data where appropriate, and minimize the number of round-trips to the server.
Handle Device Orientation and Configuration Changes
Android devices can undergo configuration changes, such as screen orientation or language changes, during the lifecycle of an app. Ensure that your Xamarin.Android app handles these changes gracefully by preserving important data and maintaining a smooth user experience. Utilize the onSaveInstanceState and onRestoreInstanceState methods to save and restore the app’s state during configuration changes.
csharp protected override void OnSaveInstanceState(Bundle outState) { outState.PutString("key", value); // Save important data base.OnSaveInstanceState(outState); } protected override void OnRestoreInstanceState(Bundle savedInstanceState) { var value = savedInstanceState.GetString("key"); // Restore important data base.OnRestoreInstanceState(savedInstanceState); }
Implement App Permissions Properly
Android apps often require specific permissions to access device resources or perform certain operations. Follow best practices for handling permissions by requesting them at runtime when needed and explaining to the user why the permission is required. Be mindful of handling permission request results and provide graceful fallbacks if a permission is denied.
Test on Real Devices and Emulators
To ensure your Xamarin.Android app functions well across different devices, thoroughly test it on real devices and emulators. Emulators are useful for testing different screen sizes, orientations, and device configurations, while real devices help you identify performance issues and platform-specific bugs. Utilize automated testing frameworks like Xamarin.UITest to create robust test suites that can be run on a variety of devices.
Utilize the Android Support Libraries
The Android Support Libraries provide backward compatibility and additional functionality to older versions of Android. By utilizing these libraries, you can ensure your app runs smoothly on a broader range of devices. The support libraries offer features like material design components, AppCompat themes, and backward-compatible APIs.
Conclusion
Building high-quality Xamarin.Android apps requires a combination of platform knowledge, efficient coding practices, and attention to detail. By following the best practices outlined in this blog post, you can develop robust, performant, and user-friendly applications. Remember to understand the Android platform, optimize performance, handle device orientation changes, implement permissions properly, and thoroughly test your app. By leveraging these best practices, you’ll be well-equipped to create outstanding Xamarin.Android applications that delight users and stand out in the competitive app market.
Remember, Xamarin.Android is a versatile framework, and these best practices should be adapted to fit the specific needs and requirements of your app. Stay up-to-date with the latest developments in Xamarin.Android and the Android platform to ensure your apps remain at the forefront of innovation and deliver a superior user experience. Happy coding!
(Note: The code samples provided are for illustrative purposes only. Make sure to adapt them to your specific implementation and follow the official Xamarin.Android documentation for the most up-to-date code examples and guidelines.)
Table of Contents
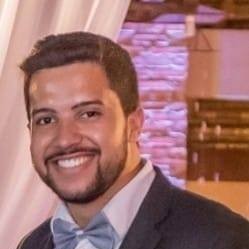
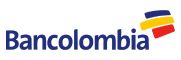