Building Cross-Platform Apps with Xamarin.Forms
In today’s digital age, the demand for cross-platform app development has skyrocketed. Businesses and developers are seeking efficient ways to build applications that can run seamlessly across multiple platforms, saving time and resources. Xamarin.Forms, a powerful framework developed by Microsoft, provides the perfect solution. In this comprehensive guide, we will explore the world of Xamarin.Forms and learn how to leverage its capabilities to develop cross-platform apps.
What is Xamarin.Forms?
Xamarin.Forms is an open-source framework that allows developers to build native user interfaces (UI) for iOS, Android, and Windows using a single codebase. It provides a rich set of UI controls and layouts that can be shared across platforms, resulting in significant time and effort savings. Xamarin.Forms follows the Model-View-ViewModel (MVVM) architectural pattern, making it easy to separate UI logic from business logic.
Advantages of Xamarin.Forms
Xamarin.Forms offers several advantages for cross-platform app development. Firstly, it allows developers to write shared code in C#, reducing the need for platform-specific languages. This increases code reuse and speeds up development time. Secondly, Xamarin.Forms provides a unified UI toolkit, enabling developers to create consistent user experiences across different platforms. Additionally, Xamarin.Forms allows direct access to native APIs, making it possible to leverage platform-specific capabilities seamlessly.
Getting Started with Xamarin.Forms
To begin building Xamarin.Forms apps, you need to set up your development environment. Install Visual Studio, Xamarin, and the necessary SDKs for Android and iOS. Once the setup is complete, create a new Xamarin.Forms project and choose the desired project structure (Portable Class Library or .NET Standard). Visual Studio will generate a basic project structure with platform-specific projects for Android, iOS, and Windows.
Code Sample:
csharp using Xamarin.Forms; namespace MyApp { public class App : Application { public App() { MainPage = new MainPage(); } } public class MainPage : ContentPage { public MainPage() { Content = new Label { Text = "Welcome to Xamarin.Forms!" }; } } }
In this code sample, we define the entry point of the application (App class) and set the MainPage to an instance of MainPage class. The MainPage class extends ContentPage and contains a Label element.
Building User Interfaces with XAML
Xamarin.Forms supports building user interfaces using XAML (eXtensible Application Markup Language), a markup language similar to HTML. XAML allows developers to define UI elements and their properties in a declarative manner. It provides a powerful way to create complex layouts and customize the appearance of controls. By separating UI definition from code, XAML promotes better code organization and collaboration between designers and developers.
Code Sample:
xaml <ContentPage xmlns="http://xamarin.com/schemas/2014/forms" xmlns:x="http://schemas.microsoft.com/winfx/2009/xaml" x:Class="MyApp.MainPage"> <StackLayout VerticalOptions="Center"> <Label Text="Welcome to Xamarin.Forms!" HorizontalOptions="CenterAndExpand" FontSize="24" /> <Button Text="Click Me!" HorizontalOptions="Center" /> </StackLayout> </ContentPage>
In this XAML code, we define a StackLayout with a Label and a Button. The VerticalOptions property sets the alignment of the StackLayout in the vertical direction, while HorizontalOptions property aligns the controls within the StackLayout horizontally. The FontSize property specifies the font size of the Label.
Implementing App Logic with C#
Once the UI is set up, it’s time to implement the app logic using C#. Xamarin.Forms allows you to write platform-agnostic code that can be shared across different platforms. You can handle user interactions, data manipulation, and business logic in a centralized manner.
Code Sample:
csharp public class MainPage : ContentPage { private Button _clickButton; private int _clickCount; public MainPage() { _clickButton = new Button { Text = "Click Me!" }; _clickButton.Clicked += OnClickButtonClicked; Content = new StackLayout { Children = { _clickButton } }; } private void OnClickButtonClicked(object sender, EventArgs e) { _clickCount++; _clickButton.Text = $"Clicked {_clickCount} times!"; } }
In this code sample, we enhance the previous MainPage by adding a button and a click event handler. The _clickCount variable keeps track of the number of clicks, and the label of the button is updated to display the click count.
Accessing Native APIs with Dependency Service
Xamarin.Forms provides a Dependency Service mechanism that enables you to access platform-specific APIs and functionality from shared code. By defining interfaces and implementing them separately for each platform, you can leverage native capabilities seamlessly.
Code Sample:
csharp public interface IToastService { void ShowToast(string message); } public class ToastService : IToastService { public void ShowToast(string message) { // Platform-specific code to display a toast notification } }
In this code sample, we define an interface called IToastService, which represents a toast notification. Then, we implement the ToastService class for each platform, providing the platform-specific code to display the toast notification.
Navigation and Page Navigation
In Xamarin.Forms, navigation is a fundamental aspect of building apps with multiple screens. You can navigate between pages and pass data between them using navigation techniques provided by Xamarin.Forms.
Code Sample:
csharp // Navigating to a new page await Navigation.PushAsync(new DetailsPage()); // Passing data to the new page var detailsPage = new DetailsPage(); detailsPage.BindingContext = selectedItem; await Navigation.PushAsync(detailsPage);
In this code sample, we demonstrate how to navigate to a new page using PushAsync. We can also pass data to the new page by setting the BindingContext property.
Data Binding and MVVM Pattern
Xamarin.Forms supports data binding, a powerful technique that allows you to establish relationships between UI elements and data sources. The Model-View-ViewModel (MVVM) architectural pattern is commonly used with Xamarin.Forms to promote separation of concerns and maintainability.
Code Sample:
xaml <Label Text="{Binding UserName}" /> <Entry Text="{Binding Email}" /> <Button Text="Save" Command="{Binding SaveCommand}" />
In this code sample, we bind the Text property of a Label and an Entry to properties (UserName and Email) of the underlying data source. The Button is bound to a SaveCommand property, which represents a command in the view model.
Working with Platform-Specific Code
While Xamarin.Forms allows for code sharing across platforms, there may be cases where you need to implement platform-specific functionality. Xamarin.Forms provides mechanisms to work with platform-specific code seamlessly.
Code Sample (iOS):
csharp [assembly: Dependency(typeof(PlatformSpecificService))] namespace MyApp.iOS { public class PlatformSpecificService : IPlatformSpecificService { public string GetDeviceInfo() { // iOS-specific implementation to retrieve device information } } }
In this code sample, we define a platform-specific service for iOS by implementing the IPlatformSpecificService interface. The [assembly: Dependency] attribute registers the implementation with the dependency service.
Testing and Debugging Xamarin.Forms Apps
Xamarin.Forms apps can be tested and debugged using various tools and techniques. Xamarin.Forms supports unit testing, UI testing, and integration with popular testing frameworks. Additionally, Xamarin.Forms provides debugging capabilities for inspecting and troubleshooting app behavior.
Publishing and Distributing Apps
Once your Xamarin.Forms app is developed and tested, it’s time to prepare it for publishing and distribution. Xamarin.Forms allows you to generate platform-specific app packages for iOS, Android, and Windows. You can then submit your app to respective app stores or distribute it internally within your organization.
Tips and Best Practices for Xamarin.Forms Development
To make the most out of Xamarin.Forms development, consider the following tips and best practices:
- Follow the MVVM architectural pattern to separate UI and business logic.
- Utilize XAML for declarative UI design and code readability.
- Leverage data binding to establish relationships between UI and data.
- Use the dependency service to access platform-specific APIs and functionality.
- Employ platform-specific styling and custom renderers for fine-grained control over the UI.
- Utilize Xamarin.Forms community packages and plugins for extending functionality.
- Regularly update Xamarin.Forms and related tools to benefit from the latest features and bug fixes.
- Test your app thoroughly on different platforms and screen sizes to ensure consistent behavior.
- Optimize performance by considering platform-specific optimizations and utilizing asynchronous programming.
- Engage with the Xamarin.Forms community for support, guidance, and inspiration.
Conclusion
Building cross-platform apps has never been easier with Xamarin.Forms. This complete guide has provided you with a comprehensive understanding of Xamarin.Forms and its capabilities. From setting up your development environment to implementing app logic, accessing native APIs, and publishing your app, you’re equipped to create powerful and efficient cross-platform applications. By leveraging the strengths of Xamarin.Forms, you can save time, reduce development effort, and reach a wider audience with your apps. Happy coding and exploring the world of cross-platform app development
Table of Contents
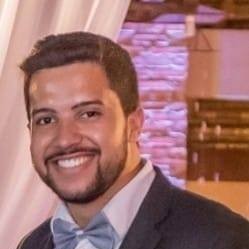
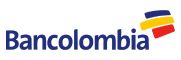