Xamarin and Firebase: Integrating Real-Time Features in Your App
In today’s fast-paced digital world, users expect real-time updates and seamless communication within their mobile apps. Xamarin, a popular cross-platform app development framework, allows developers to build native mobile apps for iOS and Android using C# and .NET. When combined with Firebase, Google’s powerful suite of cloud-based tools, Xamarin becomes even more powerful, enabling developers to integrate real-time features into their apps effortlessly.
In this blog post, we will explore how to leverage Xamarin and Firebase to add real-time capabilities to your app. We will focus on two key Firebase services: the Firebase Real-time Database for data synchronization and the Firebase Cloud Messaging for push notifications. By the end of this guide, you’ll have the knowledge and code samples to create a more engaging and interactive user experience in your Xamarin app.
Setting up Firebase for Xamarin
Before we dive into the integration process, we need to set up Firebase for our Xamarin app. Follow these steps to get started:
- Create a Firebase project in the Firebase console.
- Add your Xamarin app to the Firebase project.
- Download the google-services.json configuration file for your Xamarin app.
Integrating Firebase Real-time Database
The Firebase Real-time Database allows developers to store and sync data in real-time. Follow these steps to integrate it into your Xamarin app:
- Configure the Firebase Real-time Database rules to secure your data.
- Establish a connection between your Xamarin app and the Firebase Real-time Database.
- Use Firebase’s SDK to read and write data in real-time.
csharp // Code sample: Reading data from the Firebase Real-time Database FirebaseDatabase database = FirebaseDatabase.Instance; DatabaseReference dataRef = database.GetReference("messages"); dataRef.AddValueEventListener(new ValueEventListener() { public void OnDataChange(DataSnapshot snapshot) { foreach (DataSnapshot messageSnapshot in snapshot.Children) { string message = messageSnapshot.GetValue(true).ToString(); Console.WriteLine(message); } } public void OnCancelled(DatabaseError error) { Console.WriteLine("Error: " + error.Message); } }); // Code sample: Writing data to the Firebase Real-time Database DatabaseReference newMessageRef = dataRef.Push(); newMessageRef.SetValue("Hello, Firebase!");
Implementing Firebase Cloud Messaging
Firebase Cloud Messaging (FCM) enables you to send push notifications to your app users. Here’s how you can integrate FCM into your Xamarin app:
- Enable Firebase Cloud Messaging in the Firebase console.
- Register your app for push notifications.
- Send push notifications using the Firebase console or programmatically.
csharp // Code sample: Handling push notifications in Xamarin public class MyFirebaseMessagingService : FirebaseMessagingService { public override void OnMessageReceived(RemoteMessage message) { base.OnMessageReceived(message); if (message.Notification != null) { // Handle notification message string title = message.Notification.Title; string body = message.Notification.Body; // Display the notification to the user // ... } if (message.Data.Count > 0) { // Handle data message string dataValue = message.Data["key"]; // Process the data // ... } } }
Enhancing User Experience with Real-Time Features
Real-time features can greatly enhance the user experience in your Xamarin app. Consider implementing the following functionalities:
- Real-time chat: Enable users to communicate in real-time by implementing a chat feature using Firebase Real-time Database.
- Live updates for collaborative apps: Allow multiple users to collaborate and see real-time updates as they work on shared content.
- Real-time data synchronization between devices: Keep user data synchronized across multiple devices seamlessly using Firebase Real-time Data
Conclusion
By integrating Xamarin with Firebase, you can easily add real-time features to your app, enhancing the user experience and keeping users engaged. In this blog post, we explored how to integrate Firebase Real-time Database for data synchronization and Firebase Cloud Messaging for push notifications. We also discussed various ways to leverage real-time features to create more interactive and collaborative apps. Now it’s time for you to dive in, experiment with Firebase and Xamarin, and create amazing real-time experiences in your own apps!
Remember, with Xamarin and Firebase, the possibilities are endless. Happy coding!
Table of Contents
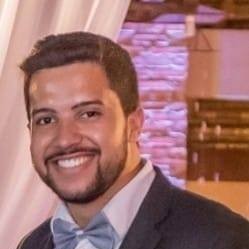
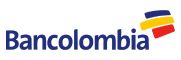