Xamarin and IoT: Building Connected Applications
In today’s interconnected world, the Internet of Things (IoT) has gained tremendous popularity. It has revolutionized the way we interact with devices, enabling seamless communication and automation. Mobile applications play a vital role in this ecosystem, providing a user-friendly interface to control and monitor IoT devices. Xamarin, a popular cross-platform development framework, offers a robust platform for building such connected applications. In this blog, we will explore the exciting possibilities of Xamarin and IoT, and provide code samples to help you kick-start your own connected application development journey.
Understanding Xamarin
Xamarin is a powerful cross-platform development framework that allows developers to build native applications for iOS, Android, and Windows using a single codebase. Leveraging the C# programming language and the .NET framework, Xamarin enables developers to write shared business logic and UI code, providing a consistent experience across multiple platforms. With its extensive libraries and tools, Xamarin offers a seamless development experience, making it a popular choice among developers.
The Internet of Things (IoT)
The Internet of Things refers to a network of interconnected devices, embedded with sensors, software, and connectivity, enabling them to collect and exchange data. These devices can be anything from smart thermostats and wearables to industrial machinery and autonomous vehicles. IoT devices generate vast amounts of data that can be leveraged for analytics, automation, and intelligent decision-making. Mobile applications provide a user-friendly interface to interact with these devices, making them an integral part of the IoT ecosystem.
Xamarin and IoT Integration
Xamarin provides excellent support for IoT integration, enabling developers to build connected applications with ease. Here are some key aspects to consider when developing Xamarin-based IoT applications:
- Device Communication: Xamarin allows seamless integration with various IoT protocols such as MQTT, HTTP, and CoAP, enabling bidirectional communication between the mobile app and IoT devices. This ensures real-time data synchronization and control.
Code Sample: Establishing a connection using MQTT in Xamarin:
csharp // Install the MQTTnet NuGet package using MQTTnet; using MQTTnet.Client; public async Task ConnectToMQTTBroker() { var factory = new MqttFactory(); var mqttClient = factory.CreateMqttClient(); var options = new MqttClientOptionsBuilder() .WithTcpServer("mqtt.example.com", 1883) .Build(); await mqttClient.ConnectAsync(options); }
2. Data Visualization: Xamarin.Forms, the UI framework in Xamarin, provides a rich set of controls and layouts for designing intuitive user interfaces. You can leverage these capabilities to create visually appealing dashboards and visualizations to display real-time data from IoT devices.
Code Sample: Displaying sensor data in Xamarin.Forms:
csharp using Xamarin.Forms; public class SensorDataPage : ContentPage { public SensorDataPage() { var sensorDataLabel = new Label(); // Update the label with real-time sensor data sensorDataLabel.Text = GetSensorDataFromDevice(); Content = new StackLayout { Children = { sensorDataLabel } }; } private string GetSensorDataFromDevice() { // Retrieve and format sensor data from the IoT device return "Temperature: 25°C, Humidity: 45%"; } }
3. Device Control: Xamarin allows developers to send commands and control IoT devices directly from the mobile application. You can create custom UI controls and actions to enable users to interact with IoT devices seamlessly.
Code Sample: Sending control commands in Xamarin:
csharp // Assuming a button named "ControlButton" in the UI var controlButton = FindViewById<Button>(Resource.Id.ControlButton); controlButton.Click += (sender, e) => { // Send control command to the IoT device SendControlCommandToDevice(); };
Security Considerations
When building IoT applications, security is of paramount importance. Xamarin provides robust security features and libraries to ensure secure communication between the mobile app and IoT devices. Implementing secure communication protocols, encrypting sensitive data, and adhering to best practices for authentication and authorization are crucial to protect user data and ensure the integrity of IoT systems.
Conclusion
Xamarin, with its cross-platform capabilities and seamless integration with IoT protocols, offers an ideal platform for developing connected applications. By combining Xamarin and IoT, developers can create powerful, user-friendly interfaces to control and monitor IoT devices from mobile devices. This blog provided an overview of Xamarin and IoT integration, along with code samples to get you started on your journey of building connected applications. Embrace the power of Xamarin and IoT, and unlock the vast potential of the interconnected world.
Incorporate the code samples and concepts shared in this blog to develop your own Xamarin-based IoT applications and embark on a fascinating development adventure. Happy coding!
Table of Contents
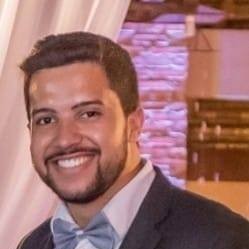
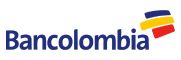