Xamarin and Machine Learning: Creating Intelligent Apps
In today’s era of digital transformation, mobile applications have become an integral part of our lives. Users now expect apps to be smart, intuitive, and personalized to their needs. This is where machine learning comes into play. By integrating machine learning capabilities into Xamarin, a popular cross-platform development framework, developers can create intelligent apps that can analyze data, make predictions, and adapt to users’ preferences. In this blog, we will explore how Xamarin and machine learning can be combined to build intelligent mobile applications.
Understanding Xamarin
Xamarin is a powerful cross-platform development framework that allows developers to build native mobile apps for iOS and Android using a single codebase. It leverages the C# programming language and the .NET framework, providing a familiar and robust development environment. Xamarin enables code sharing, rapid development, and seamless integration with device-specific features, making it an ideal choice for building mobile applications.
Integrating Machine Learning into Xamarin
To incorporate machine learning capabilities into Xamarin apps, we can leverage popular machine learning libraries and frameworks such as TensorFlow, ML.NET, or PyTorch. These libraries provide pre-trained models and algorithms that can be used for various tasks like image recognition, natural language processing, sentiment analysis, and more.
Let’s take a look at a code sample that demonstrates how to use Xamarin with TensorFlow to create an image recognition app:
csharp // Load the pre-trained TensorFlow model var model = await TensorFlowModel.LoadAsync("model.pb"); // Capture an image using the device's camera var image = await TakePhotoAsync(); // Preprocess the image (resize, normalize, etc.) var preprocessedImage = PreprocessImage(image); // Perform inference using the TensorFlow model var predictions = model.Predict(preprocessedImage); // Display the predictions to the user ShowPredictions(predictions);
In this example, we load a pre-trained TensorFlow model, capture an image using the device’s camera, preprocess the image, perform inference using the model, and finally display the predictions to the user.
Leveraging ML.NET in Xamarin
ML.NET is another powerful machine learning framework that integrates seamlessly with Xamarin. It provides a high-level API for training and deploying machine learning models. Let’s see how ML.NET can be used to create a sentiment analysis app:
csharp // Define the data model public class SentimentData { [LoadColumn(0)] public string SentimentText; [LoadColumn(1)] [ColumnName("Label")] public bool Sentiment; } // Load the trained ML.NET model var model = mlContext.Model.Load("sentimentModel.zip", out var schema); // Create a prediction engine var engine = mlContext.Model.CreatePredictionEngine<SentimentData, SentimentPrediction>(model); // Perform sentiment analysis on a text input var prediction = engine.Predict(new SentimentData { SentimentText = "This app is amazing!" }); // Display the sentiment prediction ShowSentimentPrediction(prediction);
In this code snippet, we define a data model for sentiment analysis, load a pre-trained ML.NET model, create a prediction engine, perform sentiment analysis on a text input, and display the sentiment prediction to the user.
Benefits of Xamarin and Machine Learning Integration
- Cross-platform compatibility: Xamarin allows developers to build apps that run on both iOS and Android platforms, while machine learning libraries and frameworks provide cross-platform support, ensuring consistent behavior across devices.
- Reduced development time: Xamarin’s code-sharing capabilities, combined with machine learning frameworks’ pre-trained models, accelerate the development process by eliminating the need to build models from scratch.
- Enhanced user experiences: By incorporating machine learning into Xamarin apps, developers can provide personalized and intelligent experiences to users. Whether it’s voice recognition, image recognition, or sentiment analysis, the possibilities are endless.
- Improved app performance: Machine learning models can be optimized and fine-tuned to run efficiently on mobile devices, ensuring optimal performance and minimal resource consumption.
Conclusion
The combination of Xamarin and machine learning opens up a world of possibilities for creating intelligent mobile applications. By leveraging the power of machine learning libraries and frameworks, developers can build apps that analyze data, make predictions, and adapt to users’ preferences. Whether you’re working on image recognition, natural language processing, or sentiment analysis, Xamarin provides a solid foundation for integrating machine learning capabilities into your mobile apps. So, start exploring the possibilities and create intelligent, user-centric apps with Xamarin and machine learning.
Table of Contents
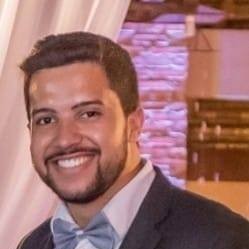
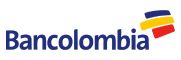