CameraX Uncovered: Expert Insights into Simplifying Camera Integration on Android
The Android CameraX API is a part of Android Jetpack, a suite of libraries that simplifies complex tasks in Android development. CameraX stands out for its user-friendly approach to camera functionality in Android applications. Unlike the traditional camera APIs that Android offered, CameraX provides a much more straightforward, concise, and less error-prone interface. You can hire Android developers for your projects to ensure greater success.This is particularly beneficial for developers looking to integrate camera capabilities without delving deep into the complex specifics of camera hardware.
Table of Contents
1. Benefits of Using CameraX Over Traditional Camera API
CameraX abstracts the underlying camera complexities, offering consistent behavior across a wide range of devices. This means that developers can focus on the implementation specifics of their application without worrying about device compatibility. Key benefits include:
- Simplified Codebase: CameraX requires significantly less code compared to the older Camera and Camera2 APIs.
- Consistent Behavior: It offers consistent behavior across different devices, which reduces the need for device-specific code.
- Extensibility: CameraX is designed to be extensible for various use-cases, including image analysis, preview, and image capture.
- Integration with Android ecosystem: CameraX works seamlessly with other Jetpack libraries and LiveData, providing a more robust and reactive approach to camera functionality.
2. Getting Started with CameraX
2.1 Setting Up the Environment
To start using CameraX, ensure you have the latest version of Android Studio and that your `build.gradle` file includes the necessary dependencies. You’ll need to add the CameraX libraries along with any additional extensions you might need.
```kotlin dependencies { // CameraX core library implementation "androidx.camera:camera-camera2:1.0.0-beta07" implementation "androidx.camera:camera-lifecycle:1.0.0-beta07" implementation "androidx.camera:camera-view:1.0.0-alpha20" // Additional extensions (optional) implementation "androidx.camera:camera-extensions:1.0.0-alpha20" } ```
For a detailed setup guide, refer to the official Android CameraX documentation.
2.2 Basic Implementation Steps
Implementing CameraX involves a few key steps:
- Permission Handling: Ensure your app has the necessary permissions for camera use.
- Creating a CameraView: Define a `CameraView` in your app’s layout to display the camera feed.
- Configuring CameraX: Set up the camera using CameraX configurations to handle different aspects like preview, image capture, and analysis.
Here’s a simple example to set up a camera preview:
```kotlin val cameraProviderFuture = ProcessCameraProvider.getInstance(this) cameraProviderFuture.addListener(Runnable { val cameraProvider: ProcessCameraProvider = cameraProviderFuture.get() val preview = Preview.Builder() .build() .also { it.setSurfaceProvider(viewFinder.surfaceProvider) } cameraProvider.bindToLifecycle(this, cameraSelector, preview) }, ContextCompat.getMainExecutor(this)) ```
For more in-depth tutorials and examples, visit CameraX CodeLab
3. Core Features of CameraX
The CameraX API provides several key features that simplify camera operations:
3.1 Image Capture
Image Capture is a fundamental feature of CameraX, allowing for high-quality photo taking. The API provides various options to customize image capture, such as setting the resolution, flash mode, and capture mode (single or burst).
3.2 Image Analysis
For applications requiring real-time image processing, CameraX’s Image Analysis feature is incredibly useful. It provides access to a buffer of the latest image captured by the camera, which can be used for face detection, barcode scanning, or custom image processing tasks.
3.3 Video Capture
Although video capture is not natively supported in the initial versions of CameraX, it is expected to be included in future releases. Developers currently rely on integrating CameraX with other APIs for video recording functionality.
4. Advanced CameraX Functionalities
4.1 Camera Controls
CameraX API offers extensive control over camera features such as zoom, flash, focus, and exposure. This allows for a more refined photography experience, suitable for applications that require more than just basic camera functionality.
For instance, implementing tap-to-focus is simplified with CameraX, as the API abstracts the complex calculations and interactions with the camera hardware:
```kotlin val meteringPoint = cameraView.meteringPointFactory .createPoint(event.x, event.y) val action = FocusMeteringAction.Builder(meteringPoint).build() cameraControl.startFocusAndMetering(action) ```
4.2 Integration with ML Kit
For advanced image processing and machine learning applications, CameraX can be seamlessly integrated with Google’s ML Kit. This combination allows developers to implement features like real-time text recognition, barcode scanning, and face detection with minimal effort.
5. Real-World Examples and Use Cases
Example 1: Building a Simple Camera App
The most straightforward use-case for CameraX is building a basic camera app. CameraX’s simplicity means that with just a few lines of code, you can have a fully functioning camera app with features like zoom, flash control, and image capture.
Example 2: Implementing Barcode Scanning
By integrating CameraX with ML Kit’s barcode scanning feature, developers can easily create an app that can scan and interpret barcodes in real-time. This can be used in various applications, from retail to logistics.
Example 3: Creating a Video Recording Application
While native video recording support in CameraX is still under development, developers can combine Camera2 API with CameraX for video recording functionality. This hybrid approach enables the creation of applications that require both photo and video capturing capabilities.
6. Best Practices and Performance Optimization
When working with CameraX, it’s essential to follow best practices to ensure optimal performance and a smooth user experience:
- Lifecycle Awareness: Make sure your camera use is tied to the appropriate lifecycle events of your Activity or Fragment.
- Handling Configuration Changes: Properly handle orientation and other configuration changes to maintain a consistent camera experience.
- Resource Management: Always release the camera and other resources when not in use to prevent memory leaks and other performance issues.
- Testing Across Devices: Test your application on various devices to ensure consistent performance, given the fragmentation in the Android ecosystem.
For more detailed guidelines on optimizing CameraX performance, check out the CameraX performance documentation.
Conclusion
The Android CameraX API revolutionizes how developers integrate camera functionalities into their applications. Its simplicity, coupled with robust features, makes it an indispensable tool for modern Android development. Whether you’re building a simple camera app or a complex application requiring advanced camera controls and machine learning integration, CameraX is a reliable and efficient choice.
You can check out our other blog posts to learn more about Android. We bring you a complete guide titled Elevating Your Skills: Essential Tips and Tricks for Android Development Mastery along with the Demystifying the Android App Lifecycle: An In-depth Exploration of Activity Execution Flow and Android Security: Best Practices to Protect User Data which will help you understand and gain more insight into the Android applications.
Table of Contents
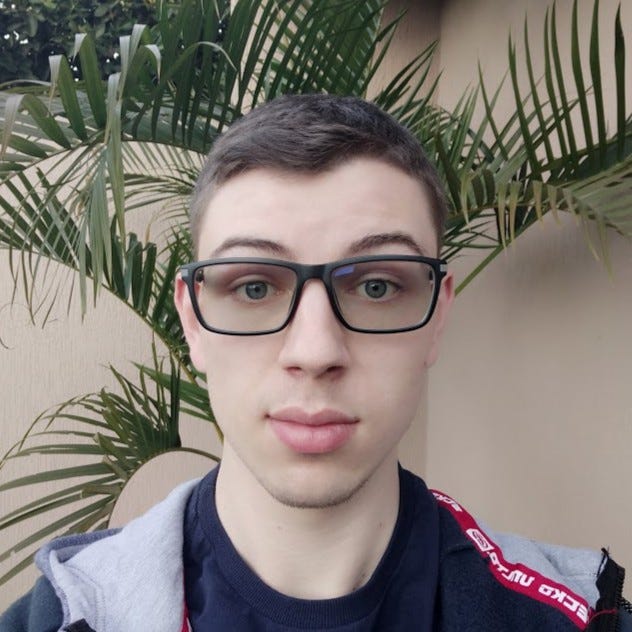
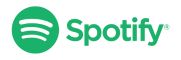