Android Security: Best Practices to Protect User Data
In today’s digital age, protecting user data is of paramount importance. With the widespread use of smartphones and the Android operating system, it’s crucial to implement robust security measures to safeguard sensitive information. This blog post highlights key best practices for Android security, providing developers with actionable steps to enhance data protection. From encryption to secure coding practices, we’ll delve into various techniques that can help strengthen the security of Android applications.
1. Encryption
Encryption is a fundamental aspect of securing user data. By encrypting sensitive information, even if it gets intercepted or accessed unauthorizedly, it remains indecipherable. Two critical areas of focus for encryption in Android are secure storage and transport layer security (TLS).
1.1 Secure Storage
When it comes to storing sensitive data on the device, it’s crucial to use encryption. Android provides the Android Keystore System to securely store cryptographic keys, passwords, and other sensitive data. This system ensures that the data remains protected even if the device is compromised. Additionally, the KeyChain API can be utilized for storing user credentials securely.
1.2 Transport Layer Security (TLS)
Securing data during transmission is equally important. Implementing Transport Layer Security (TLS) ensures that the communication between the Android application and the server is encrypted and protected from eavesdropping or tampering. Always use the latest TLS versions and strong cipher suites to minimize security vulnerabilities.
2. Permissions
Managing permissions is essential to control access to sensitive user data. Android provides a permission system that allows developers to specify the required permissions and obtain user consent before accessing sensitive resources. Here are some best practices to follow:
2.1 Restrictive Permissions
Request only the permissions necessary for the functionality of your application. Avoid requesting excessive permissions that are unrelated to your app’s core features. This reduces the risk of exposing unnecessary user data and helps build user trust.
2.2 Runtime Permissions
Starting from Android 6.0 (Marshmallow), runtime permissions were introduced, giving users more control over granting access to sensitive data. Instead of requesting all permissions at installation, apps now request permissions as needed during runtime. Ensure that your app handles permission requests gracefully and provides clear explanations to users regarding why the permission is required.
2.3 Avoid Unnecessary Permissions
Periodically review the permissions required by your app and remove any unnecessary permissions. Be cautious when integrating third-party libraries, as they may request excessive permissions. Always consider the potential privacy implications and limit permissions to the bare minimum.
3. Input Validation and Sanitization
Validating and sanitizing user input is crucial to prevent various security vulnerabilities such as code injection, SQL injection, and cross-site scripting (XSS). Here are some recommended practices for input validation and sanitization:
3.1 Validation of User Input
Validate all user input on the client-side and server-side. Implement robust input validation mechanisms to ensure that only expected input is processed by the application. Regular expression patterns, input length restrictions, and data type checks can be used to validate user input effectively.
3.2 Data Sanitization
Sanitize user input to prevent malicious content from being executed or stored. Use appropriate sanitization techniques such as escaping special characters, HTML entity encoding, and parameterized queries to protect against attacks like SQL injection and XSS.
3.3 Secure Data Transmission
When transmitting user data over the network, ensure that it is encrypted and transmitted over secure channels. Use HTTPS for web communication and establish secure connections when interacting with APIs or backend services. Avoid sending sensitive data over unsecured protocols like HTTP.
4. Secure Coding Practices
Adhering to secure coding practices is crucial to minimize security vulnerabilities and protect user data. Consider the following practices when developing Android applications:
4.1 Use Cryptographic Libraries
Utilize established cryptographic libraries and algorithms provided by Android to ensure secure data handling. Avoid creating custom encryption algorithms, as they may have security flaws. Follow recommended practices for key generation, encryption, and decryption.
4.2 Implement Secure Authentication
Implement strong authentication mechanisms, such as biometric authentication or multi-factor authentication, to protect user accounts. Avoid storing passwords or sensitive authentication data in plain text and use secure storage mechanisms like hashed passwords with salt.
4.3 Avoid Hardcoding Sensitive Data
Avoid hardcoding sensitive data, such as API keys, passwords, or cryptographic keys, directly in the code. Instead, store them securely using Android’s built-in security features like the Android Keystore System or KeyChain API. This prevents accidental exposure of sensitive information if the code is compromised.
5. Code Sample: Encryption using Android Keystore API
java import android.security.keystore.KeyGenParameterSpec; import android.security.keystore.KeyProperties; import javax.crypto.Cipher; import javax.crypto.KeyGenerator; import javax.crypto.SecretKey; // Generate a secret key KeyGenerator keyGenerator = KeyGenerator.getInstance(KeyProperties.KEY_ALGORITHM_AES, "AndroidKeyStore"); keyGenerator.init(new KeyGenParameterSpec.Builder("my_key_alias", KeyProperties.PURPOSE_ENCRYPT | KeyProperties.PURPOSE_DECRYPT) .setBlockModes(KeyProperties.BLOCK_MODE_CBC) .setEncryptionPaddings(KeyProperties.ENCRYPTION_PADDING_PKCS7) .build()); SecretKey secretKey = keyGenerator.generateKey(); // Encrypt data using the secret key Cipher cipher = Cipher.getInstance(KeyProperties.KEY_ALGORITHM_AES + "/" + KeyProperties.BLOCK_MODE_CBC + "/" + KeyProperties.ENCRYPTION_PADDING_PKCS7); cipher.init(Cipher.ENCRYPT_MODE, secretKey); byte[] encryptedData = cipher.doFinal(data);
Conclusion
Protecting user data is a responsibility that every Android developer should take seriously. By implementing the best practices discussed in this blog post, you can enhance the security of your Android applications and ensure the privacy of user information. Remember to encrypt sensitive data, manage permissions diligently, validate and sanitize user input, follow secure coding practices, and leverage the built-in security features provided by the Android platform. By prioritizing security, you contribute to building trust and confidence among your users and help create a safer digital ecosystem for all.
Table of Contents
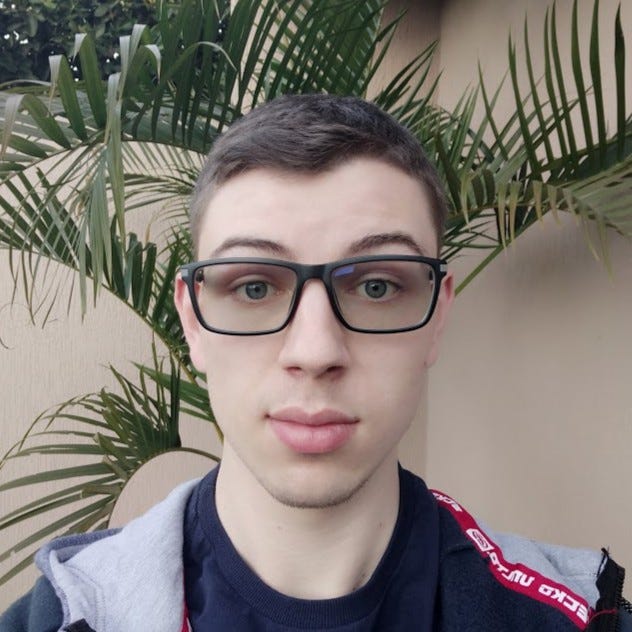
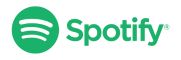