Demystifying the Android App Lifecycle: An In-depth Exploration of Activity Execution Flow
When developing an Android application, understanding the lifecycle of an app is crucial. This lifecycle defines the series of states an app goes through from the moment it’s launched to the moment it’s closed. Grasping these elements ensures your app behaves as expected, preserves user data, and conserves system resources. This understanding is part of the expertise that skilled Android developers bring to the table.
This article offers an in-depth look into the execution flow of an Android app lifecycle, which can serve as a practical guide for those looking to hire Android developers. The article is punctuated with concrete examples for better understanding.
Understanding the Basics
Every Android application is initiated from a single point, the `main()` function, like most programming languages. However, Android apps differ because their entry points are diverse, depending on the app component being executed. These components could be Activities, Services, Broadcast Receivers, and Content Providers. Here, we’ll focus more on the Activity lifecycle.
The lifecycle of an Activity in Android is a complex and intricate process. It’s broken down into several callback methods that the system calls when a state change occurs.
The Core Lifecycle Callbacks
There are seven methods in the lifecycle of an Activity:
- `onCreate()`
- `onStart()`
- `onResume()`
- `onPause()`
- `onStop()`
- `onRestart()`
- `onDestroy()`
These methods are triggered by the user navigating between apps, returning to the app, or even rotating the device. Let’s dive into each of these methods and see them in action.
onCreate()
`onCreate()` is the first method to be called when an Activity is created. It’s here where you’d initialize the essential components of your Activity. This includes setting the layout with `setContentView()`, initializing UI components, setting up event listeners, and more.
```java @Override protected void onCreate(Bundle savedInstanceState) { super.onCreate(savedInstanceState); setContentView(R.layout.activity_main); // Initialize your UI components here }
onStart()
The `onStart()` method is called immediately after `onCreate()` or when the Activity becomes visible again after being hidden. If a user navigates away and then returns to the Activity, `onStart()` will be triggered.
```java @Override protected void onStart() { super.onStart(); // The activity is about to become visible. }
onResume()
After `onStart()`, the `onResume()` method is called. This is where you would start animations or other visual-oriented operations you halted in `onPause()`.
```java @Override protected void onResume() { super.onResume(); // The activity has become visible (it is now "resumed"). }
onPause()
`onPause()` is called when the system is about to resume a previous activity or when the current activity is partially visible but not in focus. Here, it’s crucial to pause ongoing tasks that should not continue while paused, like a playing video.
```java @Override protected void onPause() { super.onPause(); // Another activity is taking focus (this activity is about to be "paused"). }
onStop()
The `onStop()` method is invoked when the Activity is no longer visible. This could occur when a new Activity is starting, an existing one is resuming, or the Activity is being destroyed.
```java @Override protected void onStop() { super.onStop(); // The activity is no longer visible (it is now "stopped"). }
onRestart()
`onRestart()` is called when the Activity is about to be restarted after being stopped. This can happen if the user navigates back to the Activity.
java @Override protected void onRestart() { super.onRestart(); // The activity is about to be restarted. }
onDestroy()
Finally, `onDestroy()` is called before the Activity is destroyed. This is the final call that the Activity receives and can happen either because the Activity is finishing (due to the user completely closing the app or `finish()` being called), or because the system is temporarily destroying it to save space.
```java @Override protected void onDestroy() { super.onDestroy(); // The activity is about to be destroyed. }
Importance of Understanding the Android App Lifecycle
Grasping the lifecycle of an Android app is a key skill for building more robust and efficient apps, a skill that professional Android developers possess. For instance, knowing when to pause heavy tasks or knowing when your app is no longer in the foreground can prevent unnecessary processing and battery drain. This technical insight is one of the reasons why businesses choose to hire Android developers, as they bring this essential knowledge to the app development process, ensuring a more refined and efficient end product.
Consider an app like Spotify. When a user navigates away from the app, the music should continue to play (`onPause()`), but the album art animation should stop. When the user closes the app, the music should stop (`onStop()`), unless it’s being played in the background.
To illustrate, let’s have a look at a pseudo-implementation of such a scenario:
```java @Override protected void onPause() { super.onPause(); // Pause animations, keep the music playing albumArtAnimation.pause(); } @Override protected void onStop() { super.onStop(); // If the music isn't supposed to keep playing, stop it. if (!isPlayingInBackground) { mediaPlayer.stop(); } }
Conclusion
Understanding and handling the Android app lifecycle effectively is critical for building high-quality apps. This expertise is part of what you gain when you hire Android developers. This understanding helps create smooth user experiences, efficient battery use, and overall, a more responsive application. By gaining insights into what happens ‘under the hood,’ developers can take control of these system-level processes, leading to apps that are more robust, efficient, and user-friendly. So, when you’re looking to hire Android developers, ensure they have a deep comprehension of the Android app lifecycle, as it plays a pivotal role in the creation of superior applications.
Table of Contents
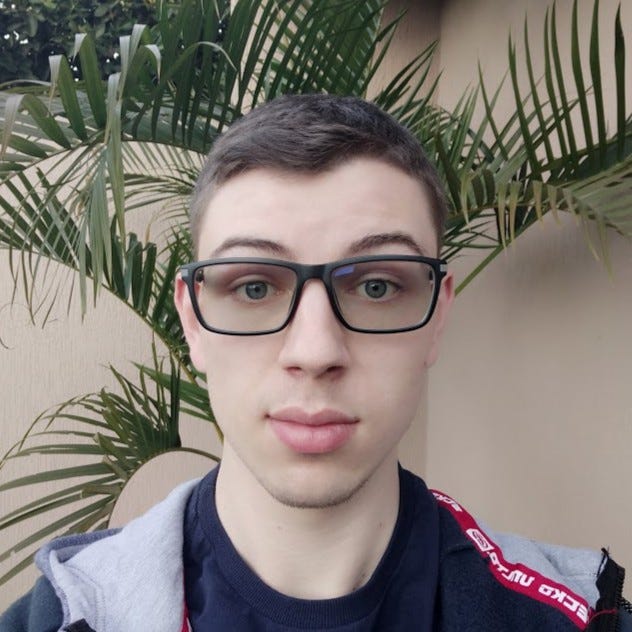
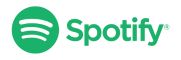