Enhance, Engage, Enthrall: Ultimate RecyclerView Animation Tips for Android
In the world of Android development, RecyclerView is a fundamental component used in a plethora of applications. It stands out due to its flexibility and efficiency in displaying dynamic data sets. However, the aesthetic appeal of an app can significantly increase by integrating animations into RecyclerView. This blog post aims to delve into the realm of RecyclerView animations, guiding you through various techniques to enhance list transitions, thereby enriching the user interface of your Android application. You can hire Android developers for your projects to ensure greater success.
Table of Contents
1. Understanding RecyclerView
1.1. What is RecyclerView?
RecyclerView is an advanced version of ListView and GridView. It’s designed to display large data sets that can be scrolled efficiently, maintaining a limited number of views.
1.2. The Importance of RecyclerView
RecyclerView’s adaptability and optimized performance make it the go-to choice for displaying dynamic and large data sets in Android applications. It’s not only about displaying data; RecyclerView also offers the potential for adding animations that can make your app feel more dynamic and engaging.
2. Types of Animations in RecyclerView
2.1. Basic Item Animations
Android’s RecyclerView comes with a set of default item animations for actions like addition, removal, and updates. These animations are subtle yet effective for basic needs.
2.2. Custom Item Animations
For more specific requirements, custom animations allow greater flexibility. Developers can create unique animations for item additions, deletions, and updates.
2.3. Layout Animations
Animating the layout process adds an extra layer of fluidity to the app. This can include animations when the layout changes or when items are rearranged within the RecyclerView.
3. Implementing Basic Item Animations
3.1. Step-by-Step Guide
To implement basic item animations in RecyclerView:
- Setup RecyclerView: Start by setting up your RecyclerView with an Adapter and a LayoutManager.
- Enable Default Animations: Use `DefaultItemAnimator` to enable basic animations for add, remove, and move events.
- Customize Animations: Modify the duration, add interpolators, or change the default animations using the `RecyclerView.ItemAnimator` class.
3.2. Code Samples
Here’s a snippet to set up a basic animation:
```java RecyclerView recyclerView = findViewById(R.id.recycler_view); recyclerView.setLayoutManager(new LinearLayoutManager(this)); recyclerView.setItemAnimator(new DefaultItemAnimator()); ```
4. Best Practices
– Keep animations subtle to enhance user experience without overwhelming them.
– Test for performance issues, especially with large data sets.
Learn More About RecyclerView Basics
5. Creating Custom Item Animations
5.1. Understanding the Animator API
Android’s Animator API allows for more complex animations. `ValueAnimator` and `ObjectAnimator` are particularly useful for creating custom RecyclerView animations.
5.2. Custom Animation Examples
Imagine an animation where items slide in from the side or fade in when added. These can be achieved using the Animator API and custom item decorators.
5.3. Integrating Custom Animations
To integrate custom animations:
- Extend RecyclerView.ItemAnimator: Create a custom class that extends `RecyclerView.ItemAnimator`.
- Override Animation Methods: Implement the methods for the add, remove, update, and move animations.
- Apply to RecyclerView: Set your custom animator to the RecyclerView.
```java public class CustomAnimator extends RecyclerView.ItemAnimator { // Implement custom animation logic here } // Setting the custom animator recyclerView.setItemAnimator(new CustomAnimator()); ```
Explore Advanced Animation Techniques
6. Advanced Techniques: Layout Animations
6.1. Conceptualizing Layout Animations
Layout animations occur when the layout of RecyclerView changes. This can be a powerful way to animate items based on layout changes like grid to list transitions.
6.2. Implementing Layout Animations
To implement layout animations:
- Define the Animation: Create an animation resource file in XML.
- Apply to LayoutManager: Use `layoutAnimation` on your RecyclerView’s LayoutManager.
```xml <layoutAnimation xmlns:android="http://schemas.android.com/apk/res/android" android:animation="@anim/item_animation_fall_down" android:animationOrder="normal" android:delay="15%" /> ```
6.3. Challenges and Solutions
– Performance: Ensure your animations are optimized for performance.
– Complexity: Start with simple animations and progressively enhance them.
Discover Layout Animation Resources
Conclusion
Incorporating animations into RecyclerView can dramatically improve the user experience of your Android app. From basic item animations to custom and layout animations, the possibilities are vast. Remember, the key is to enhance the user interface without compromising on performance. Experiment, test, and find the right balance that fits your app’s design and functionality.
You can check out our other blog posts to learn more about Android. We bring you a complete guide titled Elevating Your Skills: Essential Tips and Tricks for Android Development Mastery along with the Demystifying the Android App Lifecycle: An In-depth Exploration of Activity Execution Flow and Android Security: Best Practices to Protect User Data which will help you understand and gain more insight into the Android applications.
Table of Contents
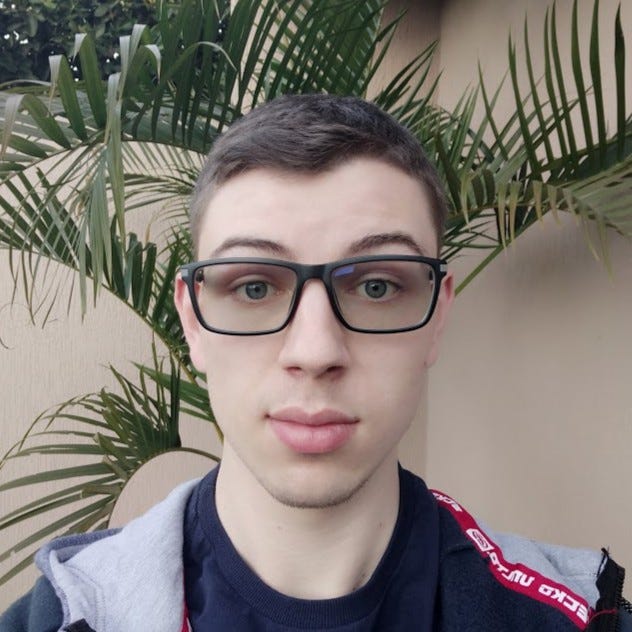
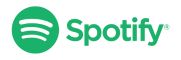