Using CakePHP’s Validation Component: Data Validation Made Easy
In the world of web development, ensuring the security and reliability of your applications is paramount. One critical aspect of achieving this is proper data validation. The CakePHP framework, known for its rapid development capabilities, provides a powerful Validation Component that streamlines the process of validating data input. In this article, we will explore how CakePHP’s Validation Component can make data validation easy, secure, and efficient for your web applications.
Table of Contents
1. Why Data Validation Matters
Data validation is the process of ensuring that the data input by users or generated within the application meets the required criteria before it’s processed further. This step is essential to prevent security vulnerabilities, data corruption, and other unwanted issues that can arise from faulty or malicious input.
Consider a scenario where a user registers on a website, providing an email address. Without proper validation, the user might enter an invalid email address, leading to communication errors and frustration. Moreover, malicious users can exploit weak validation to inject harmful data, potentially compromising the application’s security.
2. Introducing CakePHP’s Validation Component
CakePHP, a popular PHP framework, takes data validation seriously. Its Validation Component offers a comprehensive and user-friendly solution to validate data efficiently. The component integrates seamlessly into your application, allowing you to define validation rules directly in your models.
2.1. Setting Up the Validation Component
Before diving into the specifics of using the Validation Component, let’s see how to set it up within a CakePHP application:
- Include the Component: The Validation Component is included by default in CakePHP, so there’s no need to install or configure it separately.
- Create a Model: To leverage the power of the Validation Component, you need to create a model for the data you want to validate. Models serve as a bridge between your application’s data and the database, and they are the perfect place to define validation rules.
2.2. Defining Validation Rules
Defining validation rules with CakePHP’s Validation Component is intuitive and flexible. Let’s take a look at how to set up validation rules for a hypothetical “User” model:
php // File: src/Model/Table/UsersTable.php use Cake\ORM\Table; class UsersTable extends Table { public function validationDefault(Validator $validator): Validator { $validator ->notEmptyString('username', 'A username is required') ->notEmptyString('email', 'An email is required') ->email('email', 'Invalid email format') ->notEmptyString('password', 'A password is required') ->lengthBetween('password', [8, 20], 'Password must be between 8 and 20 characters'); return $validator; } }
In the example above, the validationDefault method is where you define your validation rules. In this case, we are ensuring that the username, email, and password fields are not empty, the email follows a valid format, and the password is of an acceptable length.
3. Using Validation Rules in the Controller
Once you’ve set up your validation rules, applying them to incoming data is seamless. In your controller, you can use the Validation Component to validate data before processing it:
php // File: src/Controller/UsersController.php use App\Model\Table\UsersTable; use Cake\Validation\Validation; class UsersController extends AppController { public function register() { $user = $this->Users->newEmptyEntity(); if ($this->request->is('post')) { $user = $this->Users->patchEntity($user, $this->request->getData()); if ($this->Users->save($user)) { // User registration successful } else { // Display validation errors $errors = $user->getErrors(); $this->Flash->error(__('There was an error with your registration.')); } } $this->set(compact('user')); } }
In the example above, the patchEntity method is used to apply the validation rules to the incoming data. If the data is valid, the user entity is saved. If validation fails, you can retrieve the validation errors using the getErrors method and handle them appropriately.
4. Custom Validation Rules
While CakePHP’s Validation Component offers a wide range of built-in validation rules, you might encounter situations where you need to define custom rules specific to your application. CakePHP makes it easy to create custom validation rules:
php // File: src/Model/Table/UsersTable.php use Cake\Validation\Validator; class UsersTable extends Table { public function validationDefault(Validator $validator): Validator { $validator // Existing rules... ->add('phone', 'custom', [ 'rule' => function ($value, $context) { // Implement custom validation logic // Return true if valid, false if not }, 'message' => 'Invalid phone number' ]); return $validator; } }
Conclusion
Data validation is an essential part of building secure and reliable web applications. CakePHP’s Validation Component simplifies this process by providing an intuitive way to define and apply validation rules to your data. With its built-in rules and the flexibility to create custom rules, you can ensure that your application’s data is accurate and safe.
By using CakePHP’s Validation Component, you save development time and reduce the risk of data-related issues, ultimately delivering a more polished and trustworthy user experience. So, whether you’re a seasoned CakePHP developer or just starting, integrating the Validation Component into your workflow will undoubtedly make data validation a breeze.
Table of Contents
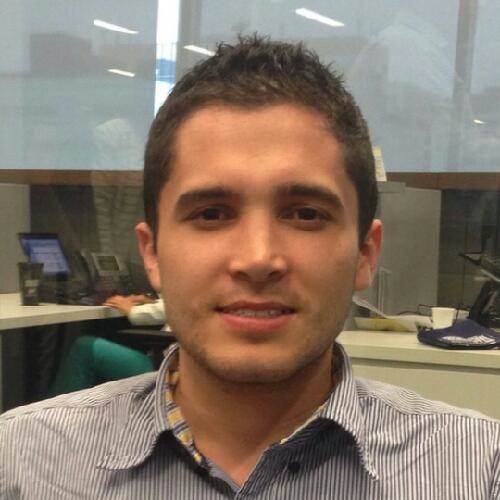
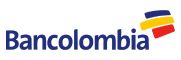