Mastering Flask: A Comprehensive Guide to Building Web Applications
Are you looking to build web applications using Python? Flask, a lightweight and powerful web framework, is an excellent choice. With its simplicity and flexibility, Flask has gained popularity among developers for creating robust and scalable web applications. In this comprehensive guide, we will explore Flask’s key features and walk you through the process of mastering Flask development.
Introduction to Flask
Flask is a micro web framework that follows the “Keep It Simple” principle. Its minimalist design allows developers to quickly build web applications without unnecessary overhead. Flask provides essential functionality for handling HTTP requests and responses, URL routing, template rendering, and session management. Its modular architecture also makes it easy to extend and customize according to specific project requirements.
Setting Up Flask Environment
Let’s begin by setting up a Flask development environment. You’ll need Python and pip installed on your system. After creating a virtual environment, install Flask using the following command:
bash $ pip install flask
Routing and URL Handling
Routing is a fundamental aspect of web applications, and Flask offers an elegant solution for handling URLs and mapping them to view functions. Take a look at the code snippet below to understand how Flask handles routing:
python from flask import Flask app = Flask(__name__) @app.route('/') def index(): return 'Hello, Flask!' if __name__ == '__main__': app.run()
Templates and Views
Flask allows you to separate the presentation layer from the business logic using templates and views. Templates help create dynamic HTML pages by embedding placeholders for data. Here’s an example of rendering a template in Flask:
python from flask import Flask, render_template app = Flask(__name__) @app.route('/') def index(): name = 'John Doe' return render_template('index.html', name=name) if __name__ == '__main__': app.run()
Forms and User Input
Building interactive web applications often requires handling user input through forms. Flask simplifies form processing and validation with the help of Flask-WTF extension. Take a look at the code snippet below to see how to create and handle forms using Flask-WTF:
python from flask import Flask, render_template from flask_wtf import FlaskForm from wtforms import StringField, SubmitField app = Flask(__name__) app.config['SECRET_KEY'] = 'your_secret_key' class MyForm(FlaskForm): name = StringField('Name') submit = SubmitField('Submit') @app.route('/', methods=['GET', 'POST']) def index(): form = MyForm() if form.validate_on_submit(): # Handle form submission logic return 'Form submitted successfully!' return render_template('form.html', form=form) if __name__ == '__main__': app.run()
Database Integration with Flask
Flask integrates seamlessly with various databases, allowing you to store and retrieve data efficiently. SQLAlchemy, a popular Object-Relational Mapping (ORM) library, provides a powerful and flexible database abstraction layer for Flask applications. Here’s an example of connecting Flask with a SQLite database:
python from flask import Flask from flask_sqlalchemy import SQLAlchemy app = Flask(__name__) app.config['SQLALCHEMY_DATABASE_URI'] = 'sqlite:///mydatabase.db' db = SQLAlchemy(app) class User(db.Model): id = db.Column(db.Integer, primary_key=True) name = db.Column(db.String(50)) @app.route('/') def index(): users = User.query.all() return render_template('index.html', users=users) if __name__ == '__main__': app.run()
User Authentication and Authorization
Securing web applications is crucial, and Flask provides various mechanisms for user authentication and authorization. Flask-Login and Flask-Principal are popular extensions that simplify the implementation of user management features. Here’s a simplified example of implementing user authentication:
python from flask import Flask from flask_login import LoginManager, UserMixin, login_user, logout_user, login_required app = Flask(__name__) app.config['SECRET_KEY'] = 'your_secret_key' login_manager = LoginManager(app) class User(UserMixin): pass @login_manager.user_loader def load_user(user_id): # Load user from the database return User() @app.route('/login') def login(): user = User() login_user(user) return 'Logged in successfully!' @app.route('/logout') @login_required def logout(): logout_user() return 'Logged out successfully!' @app.route('/') @login_required def index(): return 'Welcome to the protected area!' if __name__ == '__main__': app.run()
RESTful APIs with Flask
Flask provides excellent support for building RESTful APIs. You can easily create endpoints for handling HTTP methods like GET, POST, PUT, and DELETE. Here’s an example of creating a simple API endpoint in Flask:
python from flask import Flask, jsonify app = Flask(__name__) @app.route('/api/data', methods=['GET']) def get_data(): data = {'message': 'Hello, Flask API!'} return jsonify(data) if __name__ == '__main__': app.run()
Testing and Debugging
Flask encourages the use of testing to ensure the reliability of your web applications. The Flask-Testing extension provides a testing framework specifically designed for Flask. You can easily write test cases to validate the behavior of your application. Here’s an example of a basic test case using Flask-Testing:
python import unittest from flask import Flask from flask_testing import TestCase app = Flask(__name__) class MyTestCase(TestCase): def create_app(self): return app def test_homepage(self): response = self.client.get('/') self.assert200(response) self.assertEqual(response.data.decode(), 'Hello, Flask!') if __name__ == '__main__': unittest.main()
Deployment and Productionization
Once you have developed your Flask application, it’s time to deploy it to a production environment. Options like Docker, Heroku, or cloud platforms such as AWS or GCP can be used for deployment. Be sure to consider factors like scalability, performance, security, and monitoring when deploying your application.
Conclusion
Congratulations! You have completed this comprehensive guide to mastering Flask development. We covered Flask’s essential features, including routing, templates, forms, database integration, user authentication, RESTful APIs, testing, and deployment. With this knowledge, you are well-equipped to build powerful web applications using Flask. Start experimenting and unleash the full potential of Flask!
Table of Contents
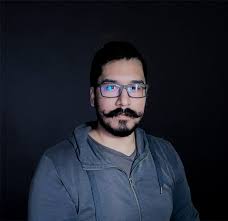