Securing Flask Applications: Best Practices and Techniques
Flask is a powerful and lightweight web framework for Python that allows developers to quickly build web applications. However, like any web application, Flask applications are susceptible to security vulnerabilities if not properly secured. In this blog post, we will explore some best practices and techniques for securing Flask applications, ensuring that your application remains robust and protected against potential attacks.
Implement Proper Authentication
Authentication is a critical aspect of securing any web application. By implementing a strong authentication system, you can ensure that only authorized users have access to sensitive functionality or data. Flask provides various authentication libraries, such as Flask-Login and Flask-JWT, that can simplify the authentication process.
Here’s an example of implementing authentication using Flask-Login:
python from flask import Flask, render_template, redirect from flask_login import LoginManager, login_user, logout_user, login_required app = Flask(__name__) app.secret_key = 'your_secret_key' login_manager = LoginManager(app) @login_manager.user_loader def load_user(user_id): # Load and return the user object based on the user_id pass @app.route('/login', methods=['GET', 'POST']) def login(): # Authenticate user credentials and login the user pass @app.route('/logout') @login_required def logout(): # Logout the current user pass @app.route('/protected') @login_required def protected(): # Protected route accessible only to authenticated users pass
Validate and Sanitize User Input
User input is a common source of security vulnerabilities, such as SQL injection and cross-site scripting (XSS). It is essential to validate and sanitize user input before using it in your application. Flask-WTF provides an excellent solution for handling forms and performing input validation.
Consider the following example:
python from flask import Flask, render_template from flask_wtf import FlaskForm from wtforms import StringField, SubmitField from wtforms.validators import DataRequired app = Flask(__name__) app.secret_key = 'your_secret_key' class MyForm(FlaskForm): username = StringField('Username', validators=[DataRequired()]) submit = SubmitField('Submit') @app.route('/', methods=['GET', 'POST']) def index(): form = MyForm() if form.validate_on_submit(): # Process the form data pass return render_template('index.html', form=form)
Protect Against Cross-Site Request Forgery (CSRF) Attacks
CSRF attacks occur when a malicious website tricks a user’s browser into making unintended requests to your application on their behalf. To prevent CSRF attacks, Flask provides built-in support for generating and validating CSRF tokens. By including the CSRF token in your forms, you can ensure that only legitimate requests are accepted.
Here’s an example of using Flask’s CSRF protection:
python from flask import Flask, render_template, request, redirect from flask_wtf.csrf import CSRFProtect app = Flask(__name__) app.secret_key = 'your_secret_key' csrf = CSRFProtect(app) @app.route('/', methods=['GET', 'POST']) def index(): if request.method == 'POST': # Process the form data pass return render_template('index.html')
Set Secure HTTP Headers
HTTP headers can provide an additional layer of security to your Flask application. By setting appropriate security headers, you can mitigate various attacks, such as cross-site scripting (XSS), clickjacking, and content sniffing. The Flask framework allows you to set custom headers easily.
Consider the following example of setting secure HTTP headers:
python from flask import Flask app = Flask(__name__) @app.after_request def set_secure_headers(response): response.headers['X-Content-Type-Options'] = 'nosniff' response.headers['X-Frame-Options'] = 'SAMEORIGIN' response.headers['X-XSS-Protection'] = '1; mode=block' return response
Secure Sensitive Configuration and Secrets
Sensitive configuration values and secrets, such as database credentials and API keys, should never be hardcoded in your Flask application’s source code. Instead, store them in environment variables or use a dedicated secrets management tool like Flask-Secrets. This approach ensures that your secrets remain protected and can be easily rotated if needed.
Here’s an example of using Flask-Secrets to manage secrets:
python from flask import Flask from flask_secrets import SecretsManager app = Flask(__name__) secrets = SecretsManager(app) @app.route('/') def index(): db_password = secrets.get('DATABASE_PASSWORD') # Use the secret value pass
Conclusion
By following these best practices and techniques, you can significantly enhance the security of your Flask applications. Remember that security is an ongoing process, so stay updated with the latest security practices and regularly review and audit your application for potential vulnerabilities.
Securing your Flask applications not only protects your data and users but also establishes trust and credibility in your application, leading to a better overall user experience.
Now it’s time to implement these security measures and build secure Flask applications!
Table of Contents
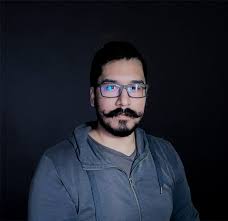