Flask-WTF: Simplifying Form Handling in Flask
Building web applications often involves handling forms, which can be a complex and error-prone task. Flask-WTF is a powerful extension for Flask that simplifies form handling, providing a seamless and intuitive way to create, validate, and process forms. In this blog post, we will explore the features and benefits of Flask-WTF and demonstrate how it can streamline your form handling workflow in Flask.
Installing Flask-WTF
To get started with Flask-WTF, ensure you have Flask installed in your virtual environment. You can install Flask-WTF using pip:
shell pip install Flask-WTF
Creating a Form
Flask-WTF allows you to define forms as classes, making form creation straightforward. Here’s an example of a simple form with two fields: “username” and “password”:
python from flask_wtf import FlaskForm from wtforms import StringField, PasswordField, SubmitField from wtforms.validators import DataRequired class LoginForm(FlaskForm): username = StringField('Username', validators=[DataRequired()]) password = PasswordField('Password', validators=[DataRequired()]) submit = SubmitField('Sign In')
Rendering the Form
To display the form in your Flask application, you need to render it within a template. Here’s an example using Jinja2 templating:
html+jinja2 <form method="POST" action="{{ url_for('login') }}"> {{ form.hidden_tag() }} {{ form.username.label }} {{ form.username() }} {{ form.password.label }} {{ form.password() }} {{ form.submit() }} </form>
Handling Form Submission
To handle form submission and validate the data, you can use the following code in your Flask view function:
python from flask import render_template, request @app.route('/login', methods=['GET', 'POST']) def login(): form = LoginForm() if form.validate_on_submit(): # Perform login logic username = form.username.data password = form.password.data # ... return render_template('login.html', form=form)
Form Validation
Flask-WTF simplifies form validation by providing various built-in validators. In the example above, we used the DataRequired validator to ensure that the fields are not submitted empty. You can explore more validators in the Flask-WTF documentation.
CSRF Protection
Flask-WTF also automatically adds CSRF (Cross-Site Request Forgery) protection to your forms. The {{ form.hidden_tag() }} in the template code generates a hidden field containing a CSRF token, which is validated during form submission.
Conclusion
Flask-WTF is an essential extension for Flask developers, simplifying form handling and validation in Flask applications. It provides a clean and intuitive interface for creating forms, validating user input, and protecting against CSRF attacks. By leveraging Flask-WTF, you can streamline your form handling workflow and focus more on building great web applications.
Start using Flask-WTF today and experience the simplicity it brings to your Flask projects!
Remember to add the necessary imports and configure your Flask application before using Flask-WTF. Check out the Flask-WTF documentation for more details and advanced features.
Happy coding with Flask-WTF!
Table of Contents
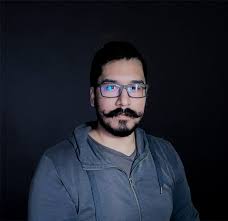