Creating Scrollable Content with jQuery Scrollbar
In today’s digital age, where user experience plays a pivotal role in the success of a website, creating a seamless and user-friendly interface has become paramount. One crucial aspect of this is managing scrollable content. Long gone are the days of default browser scrollbars that often disrupt the visual appeal of a webpage. Enter jQuery Scrollbar, a powerful tool that enables you to craft custom scrollbars, enhancing both aesthetics and functionality. In this article, we’ll delve into the world of creating scrollable content using jQuery Scrollbar, complete with step-by-step guidance and illustrative code samples.
Table of Contents
1. Understanding the Need for Custom Scrollbars
Scrollbars are an essential component of web design, especially when dealing with lengthy content that might not fit within the viewport. While browser default scrollbars serve the purpose, they might clash with your website’s design and branding. Moreover, they offer minimal customization options, limiting your creative freedom.
Custom scrollbars, on the other hand, provide a personalized touch to your website, seamlessly blending with your overall design language. They enable you to maintain a consistent look and feel while allowing you to fine-tune various aspects such as colors, sizes, and behavior.
2. Introducing jQuery Scrollbar
jQuery Scrollbar is a lightweight and easy-to-use JavaScript library that empowers developers to create custom scrollbars for their websites. By integrating this library, you can replace the standard browser scrollbars with ones that match your site’s aesthetic and offer enhanced functionality.
3. Getting Started
Before we dive into the implementation details, let’s cover the initial steps to set up jQuery Scrollbar in your project. First, make sure you have jQuery included in your project. You can either download jQuery and host it locally or include it using a content delivery network (CDN). Here’s an example of including jQuery using a CDN:
html <script src="https://code.jquery.com/jquery-3.6.0.min.js"></script>
Next, download the jQuery Scrollbar library from its official website or include it via a CDN:
html <link rel="stylesheet" href="https://cdnjs.cloudflare.com/ajax/libs/jScrollPane/2.4.3/style/jquery.jscrollpane.min.css"> <script src="https://cdnjs.cloudflare.com/ajax/libs/jScrollPane/2.4.3/script/jquery.jscrollpane.min.js"></script>
4. Implementing jQuery Scrollbar
Let’s now explore how to implement jQuery Scrollbar on a scrollable content element, such as a div with overflow. For this example, consider a section on your website that contains lengthy text. We want to apply a custom scrollbar to enhance the user experience.
4.1. HTML Structure
html <div class="custom-scroll-container"> <div class="scroll-content"> <!-- Your lengthy content goes here --> </div> </div>
4.2. CSS Styling
Before initializing the custom scrollbar, add some basic styling to ensure the container’s dimensions are appropriately set:
css .custom-scroll-container { width: 300px; /* Adjust width as needed */ height: 400px; /* Adjust height as needed */ overflow: auto; } .scroll-content { padding: 15px; }
4.3. JavaScript Initialization
Now comes the exciting part – initializing the custom scrollbar using jQuery Scrollbar. Add the following code snippet within a <script> tag at the end of your HTML file, just before the closing </body> tag:
html <script> $(document).ready(function () { $('.custom-scroll-container').jScrollPane(); }); </script>
By executing the above code, you’ve successfully integrated jQuery Scrollbar into your project. The library will automatically detect the specified container and apply the custom scrollbar to it.
5. Fine-tuning the Custom Scrollbar
While the basic implementation provides you with a functional custom scrollbar, jQuery Scrollbar offers an array of customization options to align the scrollbar with your website’s design language. Here are some ways you can fine-tune the scrollbar’s appearance and behavior:
5.1. Styling the Scrollbar
You can modify the visual aspects of the scrollbar, such as its track, thumb, and buttons. For example, to change the color of the thumb:
css .jspDrag { background: #3498db; /* Change to your desired color */ }
5.2. Adjusting Scroll Speed
By default, the scrolling speed of the custom scrollbar is determined by the user’s input. However, you can set a fixed scroll speed using the mouseWheelSpeed option:
javascript $('.custom-scroll-container').jScrollPane({ mouseWheelSpeed: 50 // Adjust the value as needed });
5.3. Disabling Scrollbar Auto-Reinitialization
jQuery Scrollbar automatically reinitializes when the content within the scrollable element changes. If you wish to disable this behavior, you can do so by setting the autoReinitialise option to false:
javascript $('.custom-scroll-container').jScrollPane({ autoReinitialise: false });
Conclusion
Incorporating custom scrollbars using jQuery Scrollbar can significantly enhance the user experience on your website. By seamlessly integrating them with your design language and providing smoother scrolling, you create a more engaging and visually appealing environment for your visitors. Remember to explore the documentation of jQuery Scrollbar to uncover more advanced features and customization options.
In today’s competitive online landscape, attention to detail sets exceptional websites apart. Through the power of jQuery Scrollbar, you’ve gained the ability to create a polished and intuitive scrollable content experience, ultimately elevating your website’s usability and aesthetics. So, why settle for the ordinary when you can craft the extraordinary? Take your web design skills to the next level by mastering jQuery Scrollbar and offering your users a browsing experience like no other.
Table of Contents
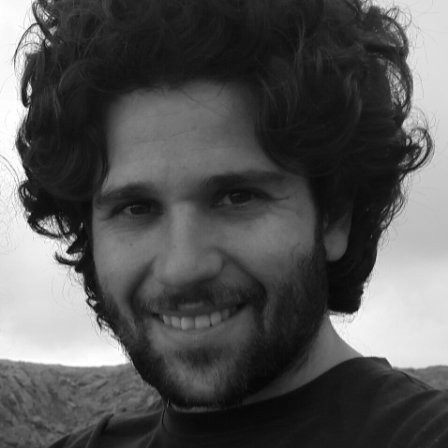
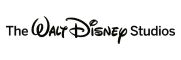