jQuery and Highcharts: Creating Interactive Charts and Graphs
In today’s data-driven world, conveying information effectively is essential. Visual representations, like charts and graphs, can make complex data more understandable and engaging. In this blog post, we’ll explore how to create interactive charts and graphs using jQuery and Highcharts. Whether you’re a seasoned developer or a novice, this comprehensive guide will walk you through the process step by step, with plenty of code samples and examples along the way.
Table of Contents
1. Introduction to Highcharts
Highcharts is a JavaScript library that allows you to add interactive and visually appealing charts to your web applications. It provides a wide range of chart types, including line charts, bar charts, pie charts, and more. Highcharts is highly customizable and can handle large datasets with ease. To get started, you’ll need to include the Highcharts library in your project. You can download it from the Highcharts website or use a content delivery network (CDN) link.
2. Setting Up Your Environment
Before we dive into creating interactive charts, let’s set up our development environment. You’ll need a basic HTML structure and include the necessary jQuery and Highcharts libraries. Here’s a simple HTML template:
html <!DOCTYPE html> <html lang="en"> <head> <meta charset="UTF-8"> <meta name="viewport" content="width=device-width, initial-scale=1.0"> <title>Interactive Charts with jQuery and Highcharts</title> <!-- Include jQuery library --> <script src="https://code.jquery.com/jquery-3.6.0.min.js"></script> <!-- Include Highcharts library --> <script src="https://code.highcharts.com/highcharts.js"></script> </head> <body> <!-- Your chart container will go here --> <div id="chart-container"></div> <!-- Your JavaScript code will go here --> </body> </html>
With this basic setup, you’re ready to start creating your interactive charts.
3. Creating Your First Chart
Let’s begin by creating a simple line chart using Highcharts. We’ll use jQuery to populate the chart with data.
Step 1: Define Your Data
First, define the data you want to visualize. For this example, let’s create a basic dataset of monthly sales data:
javascript const salesData = [ { month: 'January', sales: 1000 }, { month: 'February', sales: 1200 }, { month: 'March', sales: 800 }, { month: 'April', sales: 1500 }, // Add more data here ];
Step 2: Create the Chart
Next, you can create the chart using Highcharts. In this case, we’ll create a simple line chart. Add the following code within a <script> tag in your HTML file:
javascript $(document).ready(function () { Highcharts.chart('chart-container', { title: { text: 'Monthly Sales Data' }, xAxis: { categories: salesData.map(item => item.month) }, yAxis: { title: { text: 'Sales' } }, series: [{ name: 'Sales', data: salesData.map(item => item.sales) }] }); });
In this code, we’re telling Highcharts to render a line chart in the ‘chart-container’ <div> element. We specify the chart’s title, X-axis categories (months), Y-axis title, and the data series (monthly sales).
Step 3: Display the Chart
That’s it! When you open your HTML file in a web browser, you’ll see your first interactive chart displaying the monthly sales data.
4. Making Your Chart Interactive
Now that you have your basic chart, let’s make it more interactive. Highcharts provides various interactive features out of the box, such as tooltips, zooming, and exporting. Let’s explore some of these features.
4.1. Adding Tooltips
Tooltips provide additional information when you hover over data points on the chart. Highcharts makes it easy to enable tooltips:
javascript tooltip: { formatter: function () { return `<b>${this.x}</b><br/>Sales: ${this.y}`; } }
Add the tooltip configuration to your chart options, and now, when you hover over data points, you’ll see tooltips displaying the month and sales value.
4.2. Zooming and Panning
Highcharts allows users to zoom in on specific sections of the chart for a closer look. It also supports panning, which lets users move around the chart. To enable these features, add the following code:
javascript chart: { zoomType: 'x' // Enable X-axis zooming },
With this configuration, users can click and drag to select an area for zooming and use the mouse wheel to zoom in and out on the X-axis.
4.3. Exporting the Chart
Highcharts provides an easy way to export your charts as images or PDFs. To add exporting options, include the following code:
javascript exporting: { enabled: true, buttons: { contextButton: { menuItems: ['downloadPNG', 'downloadJPEG', 'downloadPDF', 'downloadSVG'], }, }, },
This code enables an export button on your chart, allowing users to save it in various formats.
4.4. Customizing Your Chart
Highcharts offers extensive customization options to make your charts match your project’s design and requirements. Here are some common customizations you can apply:
4.5. Changing Chart Type
Highcharts supports multiple chart types. To change your line chart to a different type, such as a bar chart or a pie chart, simply modify the ‘chart’ configuration in your chart options.
javascript chart: { type: 'bar' // Change chart type to bar },
4.6. Styling the Chart
You can customize the appearance of your chart by changing colors, fonts, and other visual elements. Highcharts provides a wide range of styling options to achieve the desired look and feel.
javascript plotOptions: { series: { color: '#0070C0', // Change line color marker: { fillColor: 'white', // Change marker color lineWidth: 2 // Adjust marker line width } } }
4.7. Adding Labels and Legends
To make your chart more informative, consider adding labels and legends:
javascript legend: { enabled: true, // Show legend },
You can also add data labels to individual data points:
javascript plotOptions: { series: { dataLabels: { enabled: true, format: '{point.y}', // Display the data value } } }
Customize the labels and legends to best convey the information you want to present.
5. Handling Dynamic Data
In real-world applications, data is often dynamic and may change over time. Highcharts makes it easy to update your chart with new data without reloading the entire page. Here’s how to handle dynamic data updates:
Step 1: Prepare Your Data
First, ensure you have a mechanism to fetch updated data from your server or another data source. For this example, we’ll simulate dynamic data by generating random sales values for each month.
javascript function generateRandomSalesData() { const newData = []; for (let i = 0; i < salesData.length; i++) { newData.push({ month: salesData[i].month, sales: Math.floor(Math.random() * 2000) }); } return newData; }
Step 2: Update the Chart
Next, create a function to update the chart with new data. You can use the update method provided by Highcharts to achieve this:
javascript function updateChart() { const newSalesData = generateRandomSalesData(); // Update X-axis categories and data series chart.xAxis[0].setCategories(newSalesData.map(item => item.month)); chart.series[0].setData(newSalesData.map(item => item.sales)); }
Step 3: Trigger Updates
Now, you can trigger data updates at regular intervals or in response to user actions. For example, you can update the chart every few seconds:
javascript setInterval(updateChart, 5000); // Update every 5 seconds
This code calls the updateChart function every 5 seconds, causing the chart to display new data.
Conclusion
In this comprehensive guide, we’ve explored how to create interactive charts and graphs using jQuery and Highcharts. You’ve learned how to set up your environment, create basic charts, make them interactive, customize their appearance, and handle dynamic data updates. With these skills, you can effectively convey complex information to your audience in a visually engaging way.
Remember that Highcharts offers even more features and customization options, so feel free to explore the official documentation to unlock the full potential of this powerful charting library. Whether you’re building a data dashboard, a financial report, or a data-driven website, Highcharts and jQuery provide a robust solution for your charting needs. Happy charting!
Table of Contents
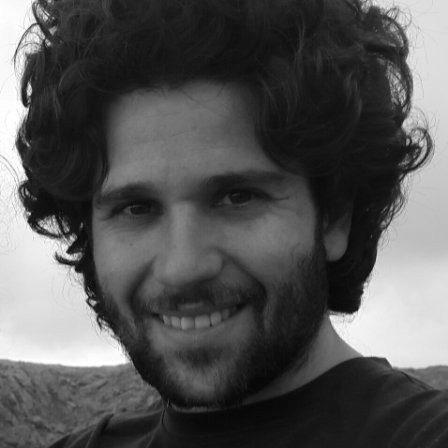
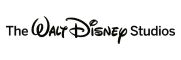