jQuery and Slick: Creating Responsive Carousels
In the dynamic world of web design, creating responsive and visually appealing carousels is essential to engage your audience. Whether you want to showcase your products, share your portfolio, or simply add an interactive element to your website, carousels are an excellent choice. In this comprehensive guide, we’ll explore how to achieve this using jQuery and the Slick carousel library. We’ll walk you through the process step by step, complete with code samples and explanations.
Table of Contents
1. Understanding the Basics
Before diving into the technical aspects, let’s clarify what jQuery and Slick are and why they are crucial for building responsive carousels.
1.1. jQuery: The Swiss Army Knife of Web Development
jQuery is a fast, small, and feature-rich JavaScript library. It simplifies various tasks, making it easier to manipulate HTML documents, handle events, create animations, and interact with server-side data. Its broad range of functions and extensive support within the web development community makes it a powerful choice for enhancing the interactivity and functionality of your website.
1.2. Slick Carousel: A Versatile Slider Library
Slick is a popular jQuery carousel library that allows you to create highly customizable and responsive sliders effortlessly. With Slick, you can transform a static set of images or content into dynamic, engaging carousels that adapt to various screen sizes and orientations. Its extensive options and settings enable you to design carousels that match your unique requirements.
2. Setting Up Your Project
Before you can start creating responsive carousels, you need to set up your project. Here’s a step-by-step guide to get you started:
Step 1: Project Structure
Create a project directory and organize your files neatly. Here’s a typical structure for a simple carousel project:
arduino project/ ? index.html ? style.css ? script.js ? slick/ ? ??? slick.min.js ? ??? slick.css ? ??? slick-theme.css ? images/ ? ??? image1.jpg ? ??? image2.jpg ? ??? …
In this structure, index.html is your main HTML file, style.css is the stylesheet, script.js contains your JavaScript code, and the slick/ directory houses the Slick carousel library and its styles. Place your carousel images in the images/ directory.
Step 2: HTML Markup
In your index.html file, create the HTML structure for your carousel. Here’s a minimal example:
html <!DOCTYPE html> <html> <head> <title>Responsive Carousel</title> <link rel="stylesheet" type="text/css" href="style.css"> </head> <body> <div class="carousel"> <div><img src="images/image1.jpg" alt="Image 1"></div> <div><img src="images/image2.jpg" alt="Image 2"></div> <!-- Add more slides as needed --> </div> <script src="https://code.jquery.com/jquery-3.6.0.min.js"></script> <script src="slick/slick.min.js"></script> <script src="script.js"></script> </body> </html>
This code sets up a basic HTML structure with two images inside a div with the class “carousel.” We’ve included the necessary CSS and JavaScript files as well.
Step 3: Styling Your Carousel
In style.css, you can define the appearance of your carousel. Customize the width, height, colors, and other visual aspects to match your website’s design.
css /* Add your styles here */ .carousel { width: 80%; margin: 0 auto; } .carousel img { width: 100%; height: auto; }
These CSS rules set the width of the carousel to 80% of the parent container and ensure that the images adapt to the container’s width while maintaining their aspect ratio.
Step 4: Initializing Slick
Now, it’s time to add the magic of Slick to your carousel. In script.js, you’ll initialize the Slick carousel with the desired options.
javascript $(document).ready(function(){ $('.carousel').slick({ autoplay: true, autoplaySpeed: 2000, arrows: true, dots: true // Add more options as needed }); });
In this example, the carousel is set to autoplay, display arrows for navigation, and show dots for slide indicators. You can customize these options to suit your preferences.
3. Making Your Carousel Responsive
One of the significant advantages of using Slick is its built-in responsiveness. Your carousel will automatically adjust to different screen sizes, but you can fine-tune this behavior further.
3.1. Customizing Responsive Settings
Slick allows you to define different settings for various screen sizes using the responsive option. This is especially useful when you want to change the number of visible slides or other carousel settings on different devices.
Here’s an example of how to customize the carousel for smaller screens:
javascript $(document).ready(function(){ $('.carousel').slick({ autoplay: true, autoplaySpeed: 2000, arrows: true, dots: true, responsive: [ { breakpoint: 768, // Adjust this value as needed settings: { slidesToShow: 2, slidesToScroll: 2 } }, { breakpoint: 480, // Adjust this value as needed settings: { slidesToShow: 1, slidesToScroll: 1 } } ] }); });
In this example, when the screen width drops below 768 pixels, the carousel will display 2 slides at a time, and when it’s below 480 pixels, it will display 1 slide at a time. You can customize the breakpoint values and settings according to your design requirements.
4. Enhancing Your Carousel
Now that you have a basic responsive carousel set up, let’s explore some additional features and enhancements you can apply.
4.1. Adding Navigation Arrows
You can enhance user navigation by adding custom navigation arrows. Here’s how to do it:
javascript $(document).ready(function(){ $('.carousel').slick({ autoplay: true, autoplaySpeed: 2000, prevArrow: '<button class="slick-prev">Previous</button>', nextArrow: '<button class="slick-next">Next</button>', // other settings... }); });
In this code, we’ve added custom HTML for the previous and next buttons. You can style these buttons to match your website’s design.
4.2. Customizing Dots
To further customize the slide indicators (dots), you can modify their appearance using CSS. Here’s an example:
css /* Customize slick-dots */ .slick-dots { display: flex; justify-content: center; align-items: center; list-style: none; padding: 0; } .slick-dots li { margin: 0 6px; } .slick-dots li button { width: 10px; height: 10px; border-radius: 50%; background-color: #333; /* Change to your desired color */ }
This CSS code centers the dots and customizes their appearance. Adjust the values as needed to match your design.
4.3. Adding Captions
If you want to include captions for each slide, you can modify your HTML structure and add the captions in the div elements inside your carousel:
html <div class="carousel"> <div> <img src="images/image1.jpg" alt="Image 1"> <div class="caption">Caption 1</div> </div> <div> <img src="images/image2.jpg" alt="Image 2"> <div class="caption">Caption 2</div> </div> <!-- Add more slides with captions --> </div>
Then, use CSS to style the captions:
css /* Style captions */ .caption { background-color: rgba(0, 0, 0, 0.5); color: #fff; position: absolute; bottom: 0; left: 0; width: 100%; padding: 10px; }
This CSS code creates a semi-transparent black background for the captions and positions them at the bottom of each slide.
Conclusion
Creating responsive carousels using jQuery and Slick is a fantastic way to add interactivity and engagement to your website. With Slick’s flexibility and customization options, you can tailor your carousel to suit your design needs, whether you’re building an online store, showcasing your portfolio, or simply enhancing your site’s visual appeal.
Remember that these examples provide a solid foundation for building responsive carousels, but the possibilities are endless. Experiment with different settings, styles, and animations to create a carousel that perfectly complements your website’s unique identity. Your users will appreciate the dynamic and interactive experience you’ve created.
Incorporate these techniques into your web design toolbox, and elevate your websites with captivating and responsive carousels. Happy coding!
Table of Contents
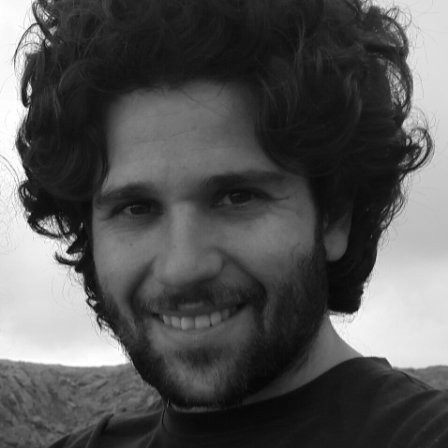
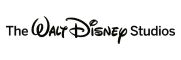