Simplifying AJAX File Uploads with jQuery File Upload
In today’s digital age, file uploads are an integral part of web applications. Whether you’re building a social media platform, an e-commerce website, or a project management tool, allowing users to upload files is a common requirement. However, handling file uploads can be a daunting task, especially if you’re new to web development.
Table of Contents
Fortunately, there are powerful tools and libraries available to make this process much more straightforward. One such tool is jQuery File Upload. In this comprehensive guide, we will walk you through the process of simplifying AJAX file uploads with jQuery File Upload, providing you with code samples and step-by-step instructions.
1. What is jQuery File Upload?
jQuery File Upload is a powerful and customizable jQuery plugin that simplifies the process of adding file upload functionality to your web application. It provides a user-friendly interface for selecting and uploading files, as well as robust server-side integration options for handling the uploaded files.
Here’s why jQuery File Upload is a fantastic choice for your file upload needs:
1.1. User-Friendly Interface
jQuery File Upload offers an intuitive and visually appealing interface for users to select and upload files. This means a better user experience and increased user engagement on your website.
1.2. Cross-Browser Compatibility
One of the most significant advantages of using jQuery File Upload is its cross-browser compatibility. It works seamlessly across various browsers, ensuring that your users can upload files regardless of their chosen browser.
1.3. Customizable
jQuery File Upload is highly customizable, allowing you to adapt it to your application’s specific needs. You can change the appearance, behavior, and functionality to match your design and requirements.
1.4. AJAX File Uploads
This plugin uses AJAX to perform file uploads, which means there’s no need for page reloads during the upload process. This results in a smoother and more efficient user experience.
1.5. Server-Side Integration
jQuery File Upload can be easily integrated with popular server-side technologies like PHP, Node.js, and Ruby on Rails, making it a versatile choice for a wide range of web development projects.
Now that you understand why jQuery File Upload is an excellent choice let’s dive into the steps to simplify AJAX file uploads using this powerful tool.
2. Getting Started with jQuery File Upload
Before we proceed, make sure you have the following prerequisites in place:
- A basic understanding of HTML, JavaScript, and jQuery.
- jQuery library included in your project.
- A server-side script (e.g., PHP, Node.js) to handle file uploads on the server.
Step 1: Download jQuery File Upload
To get started, you need to download jQuery File Upload. You can either download it from the official website or include it using a package manager like npm or yarn. For this guide, we will use the download method.
Once you’ve downloaded the plugin, extract it to your project directory.
Step 2: Include Dependencies
Next, you’ll need to include the necessary CSS and JavaScript files in your HTML document. These files are part of the jQuery File Upload package.
html <link rel="stylesheet" href="path/to/jquery.fileupload.css"> <script src="path/to/jquery.min.js"></script> <script src="path/to/jquery.fileupload.js"></script>
Make sure to replace “path/to/” with the actual path to these files within your project directory.
Step 3: Create an HTML File Input Element
To allow users to select files for upload, create an HTML file input element in your form.
html <input type="file" name="files[]" multiple>
The multiple attribute allows users to select multiple files simultaneously.
Step 4: Initialize jQuery File Upload
Now, it’s time to initialize jQuery File Upload on your file input element. You can do this by adding a simple JavaScript snippet to your HTML document.
html <script> $(function () { $('input[name="files[]"]').fileupload(); }); </script>
This code snippet tells jQuery to enable the file upload functionality on the specified input element.
Step 5: Configure Server-Side Handling
While jQuery File Upload simplifies the client-side file selection and upload process, you still need to handle file uploads on the server. The plugin expects a specific JSON response format from your server, so you’ll need to set up your server-side script accordingly.
Here’s a basic example of how you might handle file uploads in PHP:
php <?php $upload_dir = 'uploads/'; $allowed_types = array('jpg', 'jpeg', 'png', 'gif'); if ($_SERVER['REQUEST_METHOD'] === 'POST') { $uploaded_file = $_FILES['files']['tmp_name'][0]; $file_extension = pathinfo($_FILES['files']['name'][0], PATHINFO_EXTENSION); if (in_array($file_extension, $allowed_types)) { $new_filename = uniqid() . '.' . $file_extension; $destination = $upload_dir . $new_filename; if (move_uploaded_file($uploaded_file, $destination)) { echo json_encode(array( 'status' => 'success', 'message' => 'File uploaded successfully' )); } else { echo json_encode(array( 'status' => 'error', 'message' => 'File upload failed' )); } } else { echo json_encode(array( 'status' => 'error', 'message' => 'Invalid file type' )); } } ?>
This PHP script checks if the uploaded file has a valid file extension, generates a unique filename, and moves the file to an “uploads” directory. The script then returns a JSON response indicating the upload status.
You will need to adapt this server-side code to your specific requirements and programming language, but the key is to ensure that the response format matches what jQuery File Upload expects.
Step 6: Handle Upload Events
jQuery File Upload provides various events that you can hook into to customize the behavior of your file upload process. Here are a few essential events:
fileuploadadd
This event is triggered when a file is added to the upload queue. You can use it to perform tasks like displaying file information to the user.
javascript $('input[name="files[]"]').on('fileuploadadd', function (e, data) { var fileInfo = data.files[0]; console.log('File added:', fileInfo.name); });
fileuploadprogress
This event allows you to track the progress of file uploads. You can use it to update a progress bar or display a percentage complete.
javascript $('input[name="files[]"]').on('fileuploadprogress', function (e, data) { var progress = parseInt(data.loaded / data.total * 100, 10); console.log('Upload progress:', progress + '%'); });
fileuploaddone and fileuploadfail
These events are triggered when file uploads are either successful or fail. You can use them to update the user interface with the result.
javascript $('input[name="files[]"]').on('fileuploaddone', function (e, data) { console.log('Upload complete:', data.result.message); }); $('input[name="files[]"]').on('fileuploadfail', function (e, data) { console.log('Upload failed:', data.result.message); });
These are just a few examples of the events you can utilize to enhance the user experience of your file upload feature.
3. Customizing jQuery File Upload
One of the standout features of jQuery File Upload is its customizability. You can tailor the plugin to match the look and feel of your website and add additional functionality as needed.
3.1. Styling
You can easily style the file upload interface to match your website’s design by modifying the CSS provided by the plugin. Change colors, fonts, and layouts to create a seamless integration.
3.2. Adding File Validation
To ensure users only upload valid files, you can implement client-side and server-side validation. Use JavaScript to check file types, sizes, and other attributes before initiating the upload. Server-side validation is essential to protect your server from malicious uploads.
3.3. Implementing Drag-and-Drop
jQuery File Upload supports drag-and-drop functionality out of the box. By enabling this feature, you can enhance the user experience by allowing users to drag files directly into the upload area.
3.4. Integrating with Cloud Storage
If you’re dealing with a large number of uploads or require robust storage options, you can integrate jQuery File Upload with cloud storage services like Amazon S3 or Google Cloud Storage. This allows you to store and manage uploaded files more efficiently.
4. Security Considerations
When implementing file uploads, security is paramount. Here are some key security considerations to keep in mind:
4.1. File Type Validation
Ensure that users can only upload files with allowed file extensions. Reject files that could potentially harm your server or compromise the security of your application.
4.2. File Size Limit
Set a reasonable file size limit to prevent users from uploading excessively large files that could overwhelm your server.
4.3. Sanitize File Names
Sanitize file names to remove any potentially dangerous characters or sequences that could lead to security vulnerabilities.
4.4. Protect Against Malware
Implement server-side malware scanning to detect and remove malicious files before they can harm your server or other users’ data.
4.5. Authentication and Authorization
Implement proper authentication and authorization to ensure that only authorized users can upload files. This prevents unauthorized access to sensitive areas of your application.
Conclusion
In this guide, we’ve explored how to simplify AJAX file uploads using jQuery File Upload. This powerful jQuery plugin provides an excellent solution for handling file uploads in your web applications, with its user-friendly interface, cross-browser compatibility, and extensive customization options.
By following the steps outlined in this guide, you can quickly integrate jQuery File Upload into your project and provide a seamless file upload experience for your users. Remember to consider security best practices and customize the plugin to meet your specific requirements.
So go ahead, streamline your file uploading process, and enhance your web application with the power of jQuery File Upload. Your users will thank you for it!
Start simplifying your AJAX file uploads today, and take your web development skills to the next level with jQuery File Upload. Happy coding!
Table of Contents
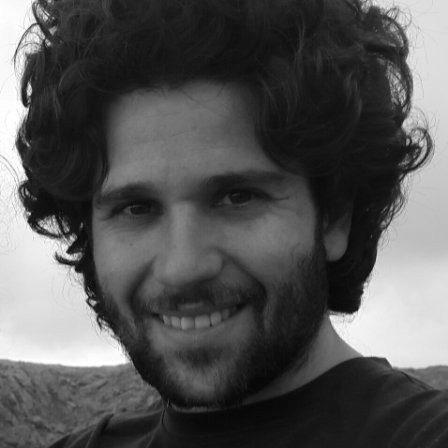
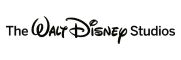