Building Autocomplete Features with jQuery UI Autocomplete
In the ever-evolving landscape of web development, user experience is paramount. One crucial element that can significantly enhance user experience is an autocomplete feature in search bars or input fields. jQuery UI Autocomplete is a robust tool that makes implementing this feature a breeze. In this comprehensive guide, we will delve into the world of jQuery UI Autocomplete, exploring its features and demonstrating how to integrate it into your web applications effectively.
Table of Contents
1. Introduction to Autocomplete
1.1. What is Autocomplete?
Autocomplete is a feature that aids users in finding relevant options or suggestions while typing in an input field. It can drastically improve the user experience by reducing the effort required to input data, especially when dealing with long lists of items or complex data structures.
1.2. Why Use jQuery UI Autocomplete?
jQuery UI Autocomplete is a widely used library that simplifies the implementation of autocomplete features in web applications. It offers a range of powerful features, including:
- Customization: Tailor the autocomplete behavior to fit your specific needs.
- Compatibility: Works seamlessly with various browsers and devices.
- Accessibility: Provides accessibility features for a better user experience.
- Remote Data Sources: Easily fetch data from remote servers.
- Theming: Customize the look and feel of the autocomplete widget.
Now, let’s roll up our sleeves and start building autocomplete features with jQuery UI Autocomplete.
2. Getting Started
2.1. Setting Up Your Environment
Before diving into jQuery UI Autocomplete, make sure you have a development environment set up. You’ll need a code editor, a web browser for testing, and a basic understanding of HTML, CSS, and JavaScript.
2.2. Downloading jQuery UI Autocomplete
To begin, download the jQuery UI library, which includes the Autocomplete module. You can obtain it from the official jQuery UI website. Once downloaded, include the necessary CSS and JavaScript files in your project.
html <!-- Include jQuery library --> <script src="https://code.jquery.com/jquery-3.6.0.min.js"></script> <!-- Include jQuery UI library with Autocomplete --> <link rel="stylesheet" href="https://code.jquery.com/ui/1.12.1/themes/base/jquery-ui.css"> <script src="https://code.jquery.com/ui/1.12.1/jquery-ui.js"></script>
2.3. Basic HTML Structure
Create a simple HTML structure for your autocomplete input field:
html <label for="search">Search: </label> <input type="text" id="search">
Now, let’s move on to implementing jQuery UI Autocomplete.
3. Implementing jQuery UI Autocomplete
3.1. Initializing Autocomplete
To enable autocomplete on the input field, add the following JavaScript code within a <script> tag in your HTML file:
javascript $(document).ready(function () { // Initialize Autocomplete $("#search").autocomplete({ source: ["Apple", "Banana", "Cherry", "Date", "Fig", "Grape", "Kiwi"] }); });
In this example, we initialize the autocomplete on the input field with the ID “search” and provide a static data source in the form of an array. As you type in the input field, Autocomplete will display suggestions based on the provided data.
3.2. Providing a Data Source
The source option in the initialization code specifies where Autocomplete should fetch its suggestions. You can provide various data sources, including:
- An Array: As shown above, provide an array of string values.
- A Function: Create a function to fetch data dynamically based on user input.
- A URL: Retrieve data from a remote server using an API.
3.3. Customizing Autocomplete Behavior
jQuery UI Autocomplete provides several options to customize its behavior according to your application’s needs. Here are a few essential options:
- minLength: Set the minimum number of characters the user must type before Autocomplete triggers suggestions.
- delay: Define a delay (in milliseconds) before Autocomplete starts searching for suggestions after the user stops typing.
- select: Specify a callback function to execute when a suggestion is selected.
javascript $("#search").autocomplete({ source: ["Apple", "Banana", "Cherry", "Date", "Fig", "Grape", "Kiwi"], minLength: 2, delay: 300, select: function (event, ui) { // Handle the selected item here console.log("Selected item: " + ui.item.value); } });
By adjusting these options, you can fine-tune the autocomplete behavior to create a seamless user experience.
4. Advanced Features
4.1. Remote Data Sources
In real-world applications, you often need to fetch suggestions from a remote server. jQuery UI Autocomplete makes this process straightforward by allowing you to specify a URL as the data source.
javascript $("#search").autocomplete({ source: function (request, response) { // Send a request to your server to fetch data $.ajax({ url: "https://example.com/api/search", data: { query: request.term }, success: function (data) { // Process the data and pass it to Autocomplete response(data); } }); } });
This code sends an AJAX request to a server endpoint (https://example.com/api/search) with the user’s input, retrieves the matching data, and displays it as Autocomplete suggestions.
4.2. Handling Selections
To perform actions when a user selects an item from the autocomplete suggestions, use the select option, as mentioned earlier. You can, for instance, populate another input field or navigate to a new page based on the selection.
javascript $("#search").autocomplete({ source: ["Apple", "Banana", "Cherry", "Date", "Fig", "Grape", "Kiwi"], select: function (event, ui) { // Populate another input field with the selected value $("#selectedItem").val(ui.item.value); } });
4.3. Theming Autocomplete
jQuery UI Autocomplete is highly customizable, allowing you to match its appearance with your application’s design. You can easily change the theme by including different CSS classes or by creating custom CSS rules.
css /* Custom Autocomplete CSS */ .ui-autocomplete { max-height: 200px; overflow-y: auto; } .ui-menu-item { padding: 10px; cursor: pointer; } .ui-menu-item:hover { background-color: #f0f0f0; }
By modifying the CSS classes associated with Autocomplete, you can achieve the desired look and feel to seamlessly integrate it into your web application’s design.
5. Tips and Best Practices
5.1. Optimizing Performance
To ensure optimal performance when using jQuery UI Autocomplete, consider the following:
- Limit Data: Avoid loading large datasets; only fetch and display relevant data.
- Debounce Input: Implement input debouncing to reduce the number of requests to the server.
- Cache Data: Cache frequently used data to minimize server requests.
5.2. Accessibility Considerations
Accessibility is a crucial aspect of web development. To make your autocomplete feature accessible, ensure that it works with screen readers and keyboard navigation. You can use ARIA attributes to enhance accessibility.
5.3. Handling Edge Cases
Think about edge cases in your application. What happens when the server doesn’t respond, or the user’s internet connection is slow? Implement error handling and provide a fallback solution.
Conclusion
jQuery UI Autocomplete is a powerful tool for enhancing the user experience in web applications. It simplifies the implementation of autocomplete features, making it easy to provide users with relevant suggestions as they type.
In this guide, we’ve covered the basics of setting up and using jQuery UI Autocomplete, including customizing its behavior and appearance. We’ve also explored advanced features like remote data sources and provided tips for optimizing performance and ensuring accessibility.
By integrating jQuery UI Autocomplete into your projects, you can create efficient and user-friendly search interfaces that improve user satisfaction and engagement. So go ahead, experiment with Autocomplete, and take your web applications to the next level!
Table of Contents
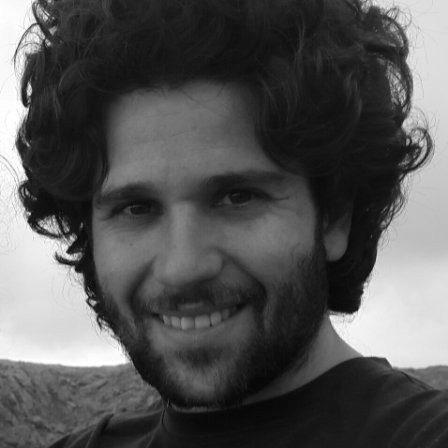
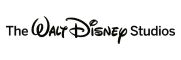