Supercharging Your WordPress Site with Advanced Programming Techniques
In the world of website development, WordPress is a popular choice due to its flexibility, ease of use, and extensive plugin ecosystem. However, as your WordPress site grows, you may encounter performance and functionality challenges. In this blog, we will explore advanced programming techniques to supercharge your WordPress site, enabling you to optimize performance, enhance user experience, and unlock new capabilities. Whether you’re a seasoned developer or a WordPress enthusiast, these expert tips and code samples will empower you to take your site to the next level.
Caching for Lightning-Fast Performance
To achieve blazing-fast page load times and reduce server load, implement caching techniques. The following code snippet demonstrates how to leverage the Transients API to cache expensive database queries and function calls:
php // Check if cached data exists $cached_data = get_transient('my_cached_data'); if (false === $cached_data) { // Data not found in cache, perform expensive operation $data = expensive_operation(); // Cache the result for 1 hour set_transient('my_cached_data', $data, 1 * HOUR_IN_SECONDS); } else { // Use cached data $data = $cached_data; }
Optimize Database Queries
Efficient database queries are crucial for WordPress performance. Use the $wpdb class to interact with the database directly and leverage indexing to speed up queries. Consider the following example:
php global $wpdb; $results = $wpdb->get_results( "SELECT * FROM {$wpdb->prefix}posts WHERE post_status = 'publish' AND post_type = 'post' ORDER BY post_date DESC LIMIT 5" );
Lazy Loading Images
Loading all images at once can significantly slow down your site. Implement lazy loading to defer image loading until they are visible on the user’s screen. The popular Lazy Load by WP Rocket plugin can handle this efficiently. Alternatively, you can achieve the same result using JavaScript. Here’s an example:
html <img src="placeholder.jpg" data-src="image.jpg" class="lazyload" alt="Image"> <script src="lazyload.js"></script>
Minify and Concatenate CSS and JavaScript
Reducing the number of HTTP requests is crucial for optimal performance. Minifying and concatenating your CSS and JavaScript files can help achieve this. Consider using tools like Gulp or Grunt, or employ plugins such as Autoptimize to automate this process.
Implementing Asynchronous Loading
By loading certain JavaScript files asynchronously, you prevent them from blocking the rendering of the page. Use the async or defer attributes in your script tags to achieve this. Here’s an example:
html <script src="script.js" async></script>
Utilize Content Delivery Networks (CDNs)
Leveraging CDNs can dramatically speed up your WordPress site by serving static assets from geographically distributed servers. Popular CDNs like Cloudflare or Amazon CloudFront offer easy integration options. Additionally, you can use plugins like W3 Total Cache to implement CDN support seamlessly.
Implement Object Caching
WordPress includes an object caching system that can significantly reduce database queries. Utilize object caching plugins like Redis Object Cache or Memcached for optimal performance. Here’s a sample configuration:
php define('WP_CACHE', true); define('WP_CACHE_KEY_SALT', 'your_unique_salt'); $redis_server = array( 'host' => '127.0.0.1', 'port' => 6379, 'database' => 0, ); define('WP_REDIS_CLIENT', 'pecl'); define('WP_REDIS_BACKEND', 'predis'); define('WP_REDIS_SENTINEL', false); define('WP_REDIS_SERVERS', serialize([$redis_server]));
Conclusion
By applying these advanced programming techniques, you can supercharge your WordPress site and unlock its full potential. From optimizing performance through caching, database query optimizations, and lazy loading to implementing asynchronous loading and utilizing CDNs, these techniques will enhance your site’s speed, user experience, and functionality. Stay ahead of the curve by implementing these expert tips and taking your WordPress site to new heights.
Table of Contents
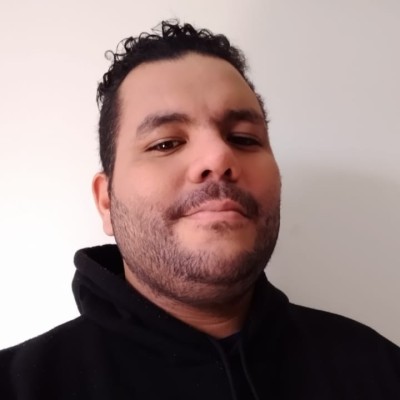
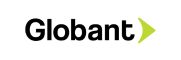