WordPress Backup and Restore: Programming Solutions
In the ever-evolving digital landscape, websites are the lifeblood of businesses, bloggers, and organizations. WordPress, one of the most popular content management systems (CMS) globally, powers over 40% of websites. While it provides a user-friendly environment for creating and managing content, it’s crucial to have a robust backup and restore strategy in place. In this blog, we’ll explore the importance of WordPress backup and restore and delve into programming solutions to ensure your website’s data and functionality are always protected.
Table of Contents
1. Why Backup and Restore is Crucial for WordPress
1.1. Protecting Your Hard Work
Your website is a culmination of hard work, creativity, and resources. Whether it’s a blog, e-commerce site, or an informational platform, losing your website’s data can be catastrophic. It’s not just about the content; it’s also the design, functionality, and user experience. Without proper backup and restore mechanisms, you risk losing all of this in a single unfortunate event.
1.2. Guarding Against Data Loss
Data loss can occur for various reasons, such as server crashes, hacking attempts, human errors, or even plugin conflicts. Having reliable backups ensures that you have a fail-safe mechanism to recover your website and its data in any of these scenarios.
1.3. Ensuring Business Continuity
For businesses, websites often serve as the primary revenue generator. Downtime due to data loss can lead to financial losses, damage to reputation, and loss of customers. Regular backups and quick restoration can minimize these risks, ensuring business continuity.
2. Manual vs. Automated Backup and Restore
Before diving into programming solutions, let’s differentiate between manual and automated backup and restore methods.
2.1. Manual Backup and Restore
Pros:
Complete control over the process.
No reliance on third-party tools.
Ideal for small websites with infrequent updates.
Cons:
Time-consuming and prone to human error.
Not suitable for large, frequently updated sites.
Lack of automation can lead to negligence.
2.2. Automated Backup and Restore
Pros:
Time-efficient and consistent.
Suitable for websites of all sizes.
Reduced risk of human error.
Cons:
May require third-party plugins or services.
Cost associated with premium solutions.
Considering the advantages of automation, let’s explore programming solutions for automated WordPress backup and restore.
3. Programming Solutions for WordPress Backup and Restore
3.1. WordPress Plugins
WordPress has a rich ecosystem of plugins that simplify backup and restore processes. Here are a few popular options:
3.1.1. UpdraftPlus
UpdraftPlus is a user-friendly plugin that offers automated backups with scheduling options. It allows you to store backups on various remote locations like Dropbox, Google Drive, and more.
Code Sample:
php // Install UpdraftPlus programmatically if (!class_exists('UpdraftPlus_Backup')) { require_once ABSPATH . 'wp-admin/includes/plugin-install.php'; $plugin = 'updraftplus/updraftplus.php'; install_plugin_install_status($plugin); $api = plugins_api('plugin_information', array('slug' => 'updraftplus')); $result = null; if (!is_wp_error($api)) { $result = install_plugin_install_status($plugin); } if (isset($result['status']) && $result['status'] !== 'installed') { $installer = install_plugin_install_status($plugin); if (!is_wp_error($installer)) { $api->download_link = $installer['download_link']; $api->plugin_name = $installer['name']; $api->version = $installer['version']; include_once ABSPATH . 'wp-admin/includes/plugin-install.php'; include_once ABSPATH . 'wp-admin/includes/class-wp-upgrader.php'; $upgrader = new Plugin_Upgrader(new WP_Upgrader_Skin()); $upgrader->install($api); } } }
3.1.2. BackWPup
BackWPup is another robust plugin that allows you to schedule automated backups and store them on various cloud services. It offers options for optimizing your database and even checking for malware.
Code Sample:
php // Install BackWPup programmatically if (!class_exists('BackWPup_Admin')) { require_once ABSPATH . 'wp-admin/includes/plugin-install.php'; $plugin = 'backwpup/backwpup.php'; install_plugin_install_status($plugin); $api = plugins_api('plugin_information', array('slug' => 'backwpup')); $result = null; if (!is_wp_error($api)) { $result = install_plugin_install_status($plugin); } if (isset($result['status']) && $result['status'] !== 'installed') { $installer = install_plugin_install_status($plugin); if (!is_wp_error($installer)) { $api->download_link = $installer['download_link']; $api->plugin_name = $installer['name']; $api->version = $installer['version']; include_once ABSPATH . 'wp-admin/includes/plugin-install.php'; include_once ABSPATH . 'wp-admin/includes/class-wp-upgrader.php'; $upgrader = new Plugin_Upgrader(new WP_Upgrader_Skin()); $upgrader->install($api); } } }
Both of these plugins offer a simple user interface for configuring backups and restoring your WordPress site.
3.2. Custom PHP Scripts
If you prefer a more hands-on approach or have specific requirements, you can create custom PHP scripts to perform WordPress backup and restore tasks.
3.2.1. Backup Script
Code Sample:
php <?php // Define backup directory $backup_dir = '/path/to/backup/directory/'; // Create a backup of the WordPress database exec("mysqldump -u username -p'password' database_name > $backup_dir/database_backup.sql"); // Create a backup of the WordPress files exec("tar -czf $backup_dir/wordpress_backup.tar.gz /path/to/wordpress"); // Compress the backup directory exec("tar -czf $backup_dir/backup_$(date +'%Y%m%d%H%M%S').tar.gz $backup_dir"); // Clean up old backups (retain the last 7 days) exec("find $backup_dir -type f -name '*.tar.gz' -mtime +7 -exec rm {} \;"); ?>
This script performs a database dump and backs up WordPress files, compressing them into a single archive. It also cleans up older backups to save storage space.
3.2.2. Restore Script
Code Sample:
php <?php // Define backup directory $backup_dir = '/path/to/backup/directory/'; // Identify the backup file to restore $restore_file = 'backup_20230828120000.tar.gz'; // Extract the backup exec("tar -xzf $backup_dir/$restore_file -C /"); // Restore the database exec("mysql -u username -p'password' database_name < $backup_dir/database_backup.sql"); ?>
This script restores your WordPress site from a specific backup file. Make sure to replace placeholders with your actual database credentials and paths.
3.3. Version Control System (VCS)
If you’re a developer and your WordPress site is under version control using tools like Git, you can leverage your VCS for backup and restore.
3.3.1. Backup Using Git
You can commit your WordPress files and database backup to a Git repository. This way, you can track changes over time and easily restore to a previous state.
Code Sample:
shell # Initialize a Git repository git init # Add WordPress files git add . # Commit changes with a meaningful message git commit -m "Backup before updating plugins" # Create a database dump mysqldump -u username -p'password' database_name > database_backup.sql # Add the database backup to the repository git add database_backup.sql # Commit the database backup git commit -m "Database backup before updating plugins"
3.3.2. Restore Using Git
To restore your WordPress site to a previous state, you can simply checkout the desired commit or branch.
Code Sample:
shell # Rollback to a specific commit git checkout <commit_hash>
Using Git for backups offers version control benefits, but keep in mind that it doesn’t replace a dedicated backup solution for complete site recovery.
4. Best Practices for WordPress Backup and Restore
While implementing backup and restore solutions, follow these best practices:
4.1. Regular Backups
Schedule backups at regular intervals, especially before performing updates or changes to your website. Daily or weekly backups are recommended, depending on your site’s update frequency.
4.2. Offsite Storage
Store backups in multiple offsite locations, such as cloud storage or remote servers. This safeguards your data in case of server failures or disasters.
4.3. Test Restorations
Periodically test the restoration process to ensure it works as expected. A backup is only valuable if you can restore your site successfully.
4.4. Security Measures
Protect your backups with strong passwords and encryption. Ensure that only authorized personnel can access and restore backups.
4.5. Documentation
Maintain clear documentation of your backup and restore procedures. This helps streamline recovery in emergencies.
Conclusion
WordPress backup and restore are essential components of website management. Whether you opt for plugins, custom scripts, or version control systems, having a reliable backup and restore strategy is non-negotiable. Protect your hard work, guard against data loss, and ensure business continuity by implementing robust backup and restore solutions for your WordPress site. Remember, it’s not a matter of if you’ll need a backup, but when.
By following the programming solutions and best practices outlined in this blog, you can rest assured that your WordPress site is well-prepared for any unforeseen challenges that may come its way. Your website’s data and functionality are too valuable not to safeguard with a solid backup and restore strategy.
Table of Contents
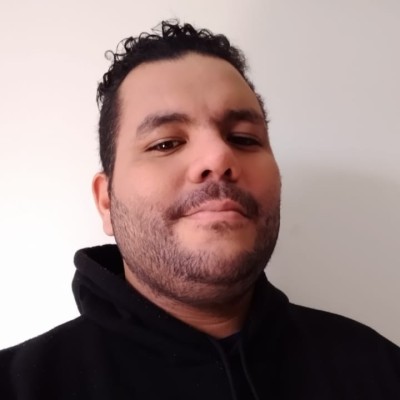
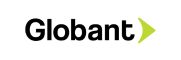