Building Secure WordPress Themes with Programming
In today’s digital landscape, security is a paramount concern for website owners. With the increasing popularity of WordPress as a content management system, it’s crucial to develop themes that prioritize security. By implementing programming techniques and following best practices, you can build secure WordPress themes that protect your website from potential vulnerabilities. In this blog, we will explore essential steps and code samples to help you create robust and secure WordPress themes.
Sanitizing and Validating User Input
One of the most common security vulnerabilities is allowing untrusted user input into your theme. To prevent cross-site scripting (XSS) attacks, it’s vital to sanitize and validate all user input. Use functions like ‘sanitize_text_field()’ and ‘esc_html()’ to sanitize input and prevent malicious code execution. Here’s an example of sanitizing user input in WordPress:
php $user_input = sanitize_text_field( $_POST['user_input'] );
Protecting Against SQL Injection
SQL injection is another significant security threat. By using prepared statements or parameterized queries, you can prevent attackers from manipulating SQL queries. WordPress provides the $wpdb global object for interacting with the database safely. Here’s an example of using prepared statements in WordPress:
php $prepared_statement = $wpdb->prepare( "SELECT * FROM wp_users WHERE user_login = %s", $user_login );
Implementing Secure Authentication
User authentication is a critical aspect of any website. When building a WordPress theme, it’s essential to implement secure authentication mechanisms. Avoid storing plain-text passwords and instead use functions like wp_hash_password() and wp_check_password() for secure password handling. Here’s an example of securely hashing and verifying passwords:
php $hashed_password = wp_hash_password( $user_password ); $is_valid = wp_check_password( $user_password, $hashed_password );
Escaping Output
To prevent cross-site scripting (XSS) vulnerabilities, it’s crucial to escape all output that comes from user input or any untrusted sources. Utilize functions like esc_html(), esc_attr(), and esc_url() to escape output appropriately. Here’s an example of escaping output in WordPress:
php echo '<div>' . esc_html( $user_input ) . '</div>';
Enforcing Secure File Handling
File handling vulnerabilities can expose your website to attacks. When handling file uploads, ensure that you validate file types, set appropriate file permissions, and sanitize file names. Utilize WordPress functions like wp_check_filetype() and sanitize_file_name() for secure file handling. Here’s an example of secure file handling in WordPress:
php $file_info = wp_check_filetype( $file_name ); if ( in_array( $file_info['ext'], array( 'jpg', 'jpeg', 'png' ) ) ) { // Handle the file upload } else { // Invalid file type }
Keeping Themes and Plugins Updated
Regularly updating your WordPress themes and plugins is crucial for maintaining security. Outdated themes or plugins may have vulnerabilities that attackers can exploit. Stay updated with the latest versions and security patches provided by theme and plugin developers.
Conclusion
Building secure WordPress themes requires a proactive approach towards implementing programming techniques and best practices. By following the steps outlined in this blog and consistently staying updated with the latest security practices, you can enhance the security of your WordPress themes and protect your website from potential vulnerabilities. Prioritize security from the start, and your WordPress themes will not only provide an engaging user experience but also offer robust protection against potential attacks.
Table of Contents
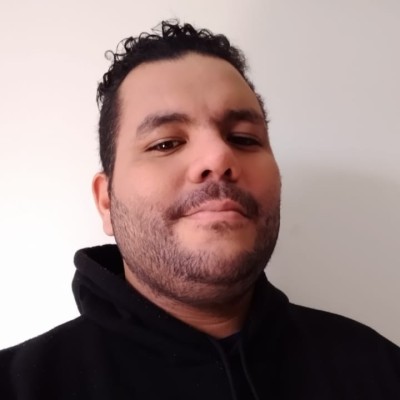
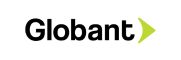