Creating a Job Board Plugin for WordPress with Programming
Are you looking to enhance your WordPress website with a job board feature? Perhaps you want to build a niche job portal or simply offer job listings as an additional service to your users. Whatever your motivation, creating a job board plugin for WordPress can be an excellent way to add value to your site. In this comprehensive guide, we will walk you through the process, step by step, using programming techniques to ensure you have full control over your job board’s functionality and appearance.
Table of Contents
1. Why Create a Custom Job Board Plugin?
Before diving into the technical details, let’s explore why you might want to create a custom job board plugin for your WordPress site rather than using an existing one.
1.1. Tailored to Your Needs
A custom job board plugin allows you to design and implement features that precisely fit your requirements. You can avoid unnecessary bloat and ensure a seamless user experience.
1.2. Full Control
You have complete control over the look and feel of your job board. This means you can integrate it seamlessly with your site’s design and branding.
1.3. No Subscription Fees
Many third-party job board services come with monthly fees. By building your own plugin, you can save money in the long run.
Now, let’s get into the nitty-gritty of building your own WordPress job board plugin.
2. Prerequisites
Before you start coding, make sure you have the following prerequisites in place:
2.1. WordPress Installation
You should have a working WordPress installation up and running. If you haven’t installed WordPress yet, you can follow the official WordPress installation guide.
2.2. Basic Knowledge of PHP and WordPress Development
This tutorial assumes you have some knowledge of PHP and WordPress development. If you’re new to these topics, you might want to check out some beginner resources first.
2.3. Code Editor
A good code editor is essential. Popular choices include Visual Studio Code, Sublime Text, and PhpStorm.
3. Building the Job Board Plugin
Now that you’ve got everything set up let’s start building our job board plugin. We’ll break this process down into several steps.
Step 1: Create a New Plugin Directory
Begin by creating a new directory for your job board plugin within the WordPress plugins directory. You can do this using FTP or directly from your server’s file manager.
bash wp-content/plugins/your-job-board-plugin/
Step 2: Create the Main Plugin File
Inside your plugin directory, create the main PHP file. Let’s name it job-board-plugin.php. This file will contain the plugin’s initialization code.
php <?php /* Plugin Name: Job Board Plugin Description: Create and manage job listings on your WordPress site. Version: 1.0 Author: Your Name */ // Your code goes here
In this code snippet, we define some metadata for our plugin, such as its name, description, version, and author. Replace ‘Your Name’ with your actual name.
Step 3: Define the Custom Post Type
Job listings are essentially a custom post type in WordPress. Let’s define our custom post type within the job-board-plugin.php file.
php // Register the custom post type function create_job_listing_post_type() { $labels = array( 'name' => 'Job Listings', 'singular_name' => 'Job Listing', 'menu_name' => 'Job Listings', 'add_new' => 'Add New', 'add_new_item' => 'Add New Job Listing', 'edit_item' => 'Edit Job Listing', 'new_item' => 'New Job Listing', 'view_item' => 'View Job Listing', 'search_items' => 'Search Job Listings', 'not_found' => 'No job listings found', 'not_found_in_trash' => 'No job listings found in trash', ); $args = array( 'labels' => $labels, 'public' => true, 'publicly_queryable' => true, 'show_ui' => true, 'show_in_menu' => true, 'query_var' => true, 'rewrite' => array( 'slug' => 'job-listing' ), 'capability_type' => 'post', 'has_archive' => true, 'hierarchical' => false, 'menu_position' => null, 'supports' => array( 'title', 'editor', 'author', 'thumbnail', 'excerpt' ), ); register_post_type( 'job_listing', $args ); } add_action( 'init', 'create_job_listing_post_type' );
In the code above, we’re registering a custom post type named ‘job_listing’ with various labels and options. This custom post type will be used to store job listings.
Step 4: Create a Job Listing Form
To allow users to submit job listings, we need to create a job listing submission form. You can do this by creating a WordPress page template or using a plugin like WPForms. Here’s an example of a simple job listing submission form using WPForms:
php [wpforms id="your-form-id"]
Replace “your-form-id” with the actual ID of your WPForms job listing submission form.
Step 5: Displaying Job Listings
Now that users can submit job listings, we need to display them on your WordPress site. Let’s create a simple template to display job listings.
Create a new file named job-listing-template.php within your plugin directory and add the following code:
php <?php /** * Template Name: Job Listing Template */ get_header(); $args = array( 'post_type' => 'job_listing', 'posts_per_page' => -1, ); $job_listings = new WP_Query( $args ); if ( $job_listings->have_posts() ) : while ( $job_listings->have_posts() ) : $job_listings->the_post(); // Your job listing display code goes here endwhile; else : echo 'No job listings found.'; endif; get_footer();
In this code, we’ve created a custom page template that queries and displays job listings. You can customize the job listing display code to match your site’s design and requirements.
Step 6: Adding Job Listing Categories and Tags
Job listings are more useful when categorized and tagged. Let’s add support for categories and tags to our custom post type.
php // Register job listing categories function create_job_listing_categories() { register_taxonomy( 'job_category', 'job_listing', array( 'label' => 'Job Categories', 'rewrite' => array( 'slug' => 'job-category' ), 'hierarchical' => true, ) ); } add_action( 'init', 'create_job_listing_categories' ); // Register job listing tags function create_job_listing_tags() { register_taxonomy( 'job_tag', 'job_listing', array( 'label' => 'Job Tags', 'rewrite' => array( 'slug' => 'job-tag' ), 'hierarchical' => false, ) ); } add_action( 'init', 'create_job_listing_tags' );
Now you can categorize and tag your job listings, making it easier for users to find relevant positions.
Step 7: Add Job Listing Fields
In most job boards, you’ll need custom fields to store additional information about job listings, such as salary, location, and application deadlines. To add custom fields, you can use the Advanced Custom Fields plugin or create them programmatically.
Here’s an example of adding custom fields programmatically to your job listings:
php // Add custom fields to job listings function add_job_listing_custom_fields() { add_post_type_support( 'job_listing', 'custom-fields' ); } add_action( 'init', 'add_job_listing_custom_fields' );
With custom fields in place, you can easily extend your job listing details.
Step 8: Implement Job Listing Search
A crucial feature of any job board is the ability for users to search for job listings based on keywords, location, category, etc. You can create a custom search form and search logic within your plugin.
php // Create a custom job listing search form function job_listing_search_form() { ?> <form role="search" method="get" id="job-search-form" action="<?php echo esc_url( home_url( '/' ) ); ?>"> <input type="text" value="<?php echo get_search_query(); ?>" name="s" id="s" placeholder="Search for job listings" /> <input type="submit" id="searchsubmit" value="Search" /> <input type="hidden" name="post_type" value="job_listing" /> </form> <?php } // Modify the search query for job listings function modify_job_listing_search( $query ) { if ( is_search() && !is_admin() && $query->is_main_query() ) { $query->set( 'post_type', 'job_listing' ); } return $query; } add_action( 'pre_get_posts', 'modify_job_listing_search' );
This code adds a custom job listing search form and modifies the WordPress search query to include job listings.
Step 9: User Management
To allow employers to submit job listings and manage them, you’ll need user management functionality. WordPress provides built-in user roles like “Subscriber,” “Author,” and “Editor.” You can use these roles or create custom roles using a plugin like Members.
Step 10: Styling Your Job Board
Finally, you’ll want to style your job board to match your site’s design. You can do this by creating custom CSS or using a WordPress theme builder like Elementor or Divi. Remember to keep your job board’s design user-friendly and responsive.
4. Testing and Deployment
Before deploying your job board plugin to a live site, thoroughly test it on a staging or development environment. Ensure that all features work as expected, and there are no compatibility issues with your theme or other plugins.
Once you’re confident in your plugin’s stability and functionality, you can deploy it to your live WordPress site. Make sure to keep regular backups and consider using version control to manage your plugin’s code.
5. Additional Features and Enhancements
Creating a basic job board plugin is just the beginning. Depending on your project’s requirements, you can enhance your plugin with features like:
- Job Application Management: Allow applicants to apply directly through your site and enable employers to manage applications.
- Paid Listings: Implement payment gateways to charge employers for posting job listings.
- Email Notifications: Send email notifications to employers and applicants when there are new applications or updates to job listings.
- Advanced Search Filters: Add advanced search options such as location-based searches and filters for job type or salary range.
Conclusion
Building a custom job board plugin for WordPress can be a rewarding project, providing valuable functionality to your website visitors. With the flexibility and control that a custom plugin offers, you can tailor the job board to your exact requirements. Remember to keep your plugin updated, provide excellent user support, and continually improve it to stay competitive in the job board market. Good luck with your job board project!
Table of Contents
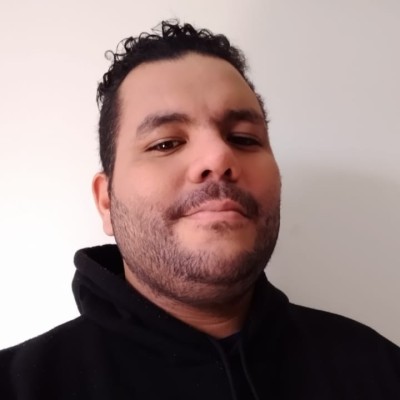
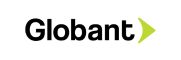