Creating a Q&A Platform with WordPress and Programming
In the digital age, information sharing and knowledge acquisition have evolved immensely. People are constantly seeking answers to their questions, and what better way to facilitate this than by creating a robust Q&A platform? If you’re eager to develop your own question and answer website, combining the versatility of WordPress with programming prowess can result in a feature-rich platform that serves users’ needs effectively. In this guide, we’ll walk you through the process, from setting up WordPress to implementing essential features using programming.
Table of Contents
1. Introduction to Q&A Platform
Q&A platforms have become a staple for knowledge sharing, fostering communities, and enabling users to seek and provide assistance. By combining WordPress, a versatile content management system, with programming techniques, you can create a platform that not only caters to users’ inquiries but also offers a seamless and engaging experience.
2. Setting Up WordPress
2.1. Installing WordPress
Begin by installing WordPress on your preferred web hosting server. Most hosting providers offer one-click installations, simplifying the process. After installation, you’ll have a basic WordPress site ready to be customized.
2.2. Choosing a Theme
Selecting a suitable theme is crucial as it sets the tone for your Q&A platform’s design. Opt for a responsive and customizable theme that aligns with your platform’s goals. You can choose from a variety of free and premium themes available in the WordPress repository.
2.3. Essential Plugins
Enhance your platform’s functionality with plugins. Some essential plugins include:
- bbPress: A forum plugin that integrates seamlessly with WordPress, making it ideal for building the Q&A structure.
- BuddyPress: If you’re aiming for a more community-focused platform, BuddyPress adds social networking features.
- Akismet: Prevent spammy content from flooding your platform’s discussions.
- Yoast SEO: Optimize your content for search engines and increase visibility.
3. Designing the Q&A Interface
3.1. Creating Custom Post Types
WordPress allows you to create custom post types, which are essential for organizing your Q&A content. You can use plugins like “Custom Post Type UI” to define custom post types for questions and answers. This ensures that your Q&A content remains separate from regular blog posts.
php // Register Custom Post Type function custom_qna_post_type() { $args = array( 'public' => true, 'label' => 'Q&A', // Add more arguments as needed ); register_post_type( 'qna', $args ); } add_action( 'init', 'custom_qna_post_type' );
3.2. Designing the User Interface
User experience is paramount in a Q&A platform. Design an intuitive and user-friendly interface. Utilize clean typography, clear navigation, and a responsive layout. Make sure users can easily search for questions, submit their own, and view answers.
4. User Authentication and Profiles
4.1. User Registration and Login
Implement user registration and login functionality to track user interactions accurately. You can use plugins like “User Registration” or “Ultimate Member” to create registration forms and manage user profiles.
php // Display registration form echo do_shortcode( '[user_registration_form id="123"]' );
4.2. User Profiles
Allow users to create profiles where they can manage their questions, answers, and engage with the community. Extend user profiles with custom fields to display relevant information such as expertise areas.
5. Building the Q&A Functionality
5.1. Submitting Questions and Answers
Enable users to submit questions and answers through a user-friendly submission form. Create a custom template for question submission and use conditional logic to differentiate between questions and answers.
php // Check if current user can submit if (current_user_can('publish_qna')) { // Display submission form echo get_template_part('submit', 'qna'); }
5.2. Voting and Best Answers
Implement voting mechanisms to allow users to upvote/downvote questions and answers. Additionally, let the original question asker mark a particular answer as the “best answer.”
php // Handle upvote button click function handle_upvote() { // Update vote count in the database $new_vote_count = get_post_meta( $post_id, 'upvotes', true ) + 1; update_post_meta( $post_id, 'upvotes', $new_vote_count ); } add_action( 'wp_ajax_upvote', 'handle_upvote' ); add_action( 'wp_ajax_nopriv_upvote', 'handle_upvote' );
5.3. Search and Filters
Ensure efficient question discovery with robust search and filter options. Allow users to search by keywords, tags, and categories. Implement AJAX-based filtering for real-time results.
6. Implementing Points and Badges
Reward user engagement by implementing a points and badges system. Users earn points for asking questions, answering, and receiving upvotes. Assign badges based on points earned to gamify the experience.
php // Calculate user points function calculate_user_points( $user_id ) { $points = get_user_meta( $user_id, 'user_points', true ); // Calculate points logic return $points; }
7. Moderation and Security
Implement moderation tools to ensure the quality of content shared on your platform. Allow experienced users to report spam and inappropriate content. Consider implementing user reputation to empower the community to self-moderate.
8. Scaling and Performance Optimization
As your Q&A platform gains traction, ensure it can handle increased traffic. Optimize database queries, implement caching mechanisms, and consider utilizing a Content Delivery Network (CDN) to deliver content faster.
Conclusion
Creating a Q&A platform using WordPress and programming is a rewarding endeavor that combines the power of a versatile CMS with the customization capabilities of coding. By following the steps outlined in this guide, you can create a feature-rich platform that fosters knowledge sharing, community engagement, and meaningful interactions among users. Remember, continuous improvement and adaptation based on user feedback will be key to the long-term success of your Q&A platform. So, roll up your sleeves, start coding, and watch your platform flourish!
Table of Contents
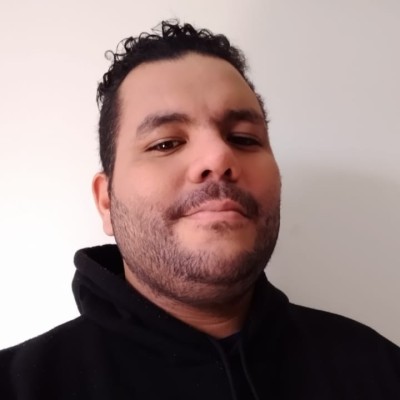
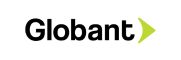