Creating Dynamic Websites with WordPress Programming
WordPress has come a long way since its inception as a simple blogging platform. Today, it powers nearly 40% of all websites on the internet, thanks to its flexibility and ease of use. While WordPress offers a wide range of pre-built themes and plugins, sometimes, you may need to go beyond these out-of-the-box solutions to create truly dynamic websites. In this blog, we will delve into the world of WordPress programming and explore how you can use it to build websites with enhanced interactivity and functionality.
1. Understanding the Basics of WordPress Programming
Before we dive into the technicalities, let’s start with a quick overview of WordPress programming and its essential components.
1.1 What is WordPress Programming?
WordPress programming involves customizing and extending the functionality of WordPress using programming languages such as PHP, JavaScript, and CSS. By writing custom code, developers can create unique themes, plugins, and custom post types to meet specific website requirements.
1.2 The WordPress Architecture
WordPress follows a hierarchical architecture that consists of the following components:
- Core: The core is the foundation of WordPress, providing basic functionality for managing content, user management, and system maintenance.
- Themes: Themes control the design and layout of a WordPress website, and they can be customized using PHP, CSS, and JavaScript.
- Plugins: Plugins add specific features and functionality to a WordPress site, and they can be developed using PHP and JavaScript.
- Database: WordPress uses a MySQL database to store content, settings, and user information.
2. Building Dynamic Themes with WordPress
2.1 Theme Development Basics
To create a dynamic website, you need a solid foundation in WordPress theme development. Start by creating a new folder in the wp-content/themes/ directory for your custom theme. Inside the folder, create a style.css file with the following structure:
css /* Theme Name: Your Theme Name Theme URI: Your Theme URL Author: Your Name Author URI: Your Website URL Description: Your theme's description Version: 1.0 */ /* Theme-specific CSS goes here */
2.2 Utilizing Custom Templates
WordPress allows you to create custom templates for different pages or sections of your website. For example, you might want a unique template for your homepage, blog posts, or custom post types. To create a custom template, add the following code at the top of your PHP file:
php /* Template Name: Custom Template */
You can then select this custom template from the WordPress page editor when editing a specific page.
3. Enhancing Functionality with Custom Plugins
3.1 Introduction to WordPress Plugins
Plugins are essential for extending the functionality of a WordPress website. By creating custom plugins, you can add unique features tailored to your website’s needs.
3.2 Creating a Basic Plugin
To create a custom WordPress plugin, follow these steps:
Step 1: Create a new folder in the wp-content/plugins/ directory for your plugin.
Step 2: Inside the folder, create a PHP file for your plugin and add the following code at the top:
php /* Plugin Name: Your Plugin Name Plugin URI: Your Plugin URL Author: Your Name Author URI: Your Website URL Description: Your plugin's description Version: 1.0 */
Step 3: Define the main functionality of your plugin within the PHP file.
Step 4: Activate your plugin from the WordPress admin panel.
3.3 Adding Shortcodes for Dynamic Content
Shortcodes are a powerful way to add dynamic content to your WordPress website without the need for complex programming. For example, you can create a shortcode to display dynamic data from the database or integrate external content.
php function dynamic_content_shortcode() { // Add your dynamic content generation code here return "Your dynamic content goes here"; } add_shortcode('dynamic_content', 'dynamic_content_shortcode');
With this shortcode, you can add the dynamic content to any page or post by simply inserting [dynamic_content].
4. Improving User Experience with AJAX
4.1 Introduction to AJAX in WordPress
AJAX (Asynchronous JavaScript and XML) allows you to update specific parts of a web page without requiring a full page reload. This can significantly improve user experience and reduce server load.
4.2 Implementing AJAX in Your Theme or Plugin
First, enqueue the JavaScript file containing your AJAX code in your theme’s functions.php file or your plugin’s main PHP file:
php function enqueue_ajax_script() { wp_enqueue_script('your-ajax-script', get_template_directory_uri() . '/js/your-ajax-script.js', array('jquery'), '1.0', true); wp_localize_script('your-ajax-script', 'your_ajax_object', array('ajax_url' => admin_url('admin-ajax.php'))); } add_action('wp_enqueue_scripts', 'enqueue_ajax_script');
Next, create a separate JavaScript file (e.g., your-ajax-script.js) to handle the AJAX request:
javascript jQuery(document).ready(function ($) { // AJAX action handler $(document).on('click', '#your-ajax-button', function () { $.ajax({ url: your_ajax_object.ajax_url, type: 'POST', data: { action: 'your_ajax_function', // Add any additional data you need to pass to the server }, success: function (response) { // Process the response from the server }, error: function (errorThrown) { console.log(errorThrown); } }); }); });
In your plugin or theme’s PHP file, add the PHP function to handle the AJAX request:
php function your_ajax_function() { // Process your AJAX request here wp_send_json_success($your_response_data); } add_action('wp_ajax_your_ajax_function', 'your_ajax_function'); add_action('wp_ajax_nopriv_your_ajax_function', 'your_ajax_function'); // For non-logged-in users
5. Integrating Custom Post Types and Taxonomies
5.1 Creating Custom Post Types
WordPress allows you to define custom post types to organize content in a more structured way. For example, you can create a custom post type for “Portfolio Items” or “Testimonials.”
php function create_custom_post_type() { register_post_type('portfolio', array( 'labels' => array( 'name' => 'Portfolio Items', 'singular_name' => 'Portfolio Item', ), 'public' => true, 'has_archive' => true, 'supports' => array('title', 'editor', 'thumbnail'), )); } add_action('init', 'create_custom_post_type');
5.2 Implementing Custom Taxonomies
Custom taxonomies allow you to group custom post types into categories or tags. For instance, you can create a custom taxonomy called “Project Categories” for your “Portfolio Items” post type.
php function create_custom_taxonomy() { register_taxonomy('project_category', 'portfolio', array( 'labels' => array( 'name' => 'Project Categories', 'singular_name' => 'Project Category', ), 'hierarchical' => true, )); } add_action('init', 'create_custom_taxonomy');
Conclusion
In this blog post, we’ve explored the world of WordPress programming and how it empowers developers to create dynamic and interactive websites. From custom themes and plugins to AJAX integration and custom post types, the possibilities are endless. By leveraging the power of WordPress programming, you can take your website to new heights, providing your users with a rich and engaging experience.
Remember, WordPress programming is an ongoing learning process, and staying updated with the latest WordPress developments and best practices will help you make the most of this powerful platform. So, roll up your sleeves, dive into the code, and unlock the full potential of WordPress to craft websites that stand out from the crowd. Happy coding!
Table of Contents
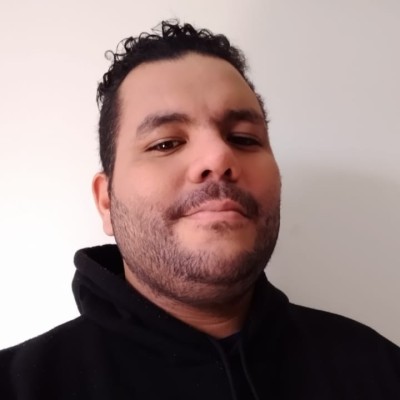
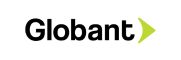