Creating Interactive Forms in WordPress with Programming
Creating forms is an essential aspect of any website, enabling seamless communication between site owners and visitors. While WordPress offers a variety of plugins for form creation, harnessing the power of programming can provide more customization and interactivity. In this guide, we will walk you through the process of creating interactive forms in WordPress using programming. Whether you’re a seasoned developer or just dipping your toes into programming, this tutorial will empower you to take your WordPress forms to the next level.
Table of Contents
1. Why Go Beyond Plugins?
WordPress boasts numerous plugins like WPForms, Contact Form 7, and Gravity Forms that simplify form creation. However, these plugins might fall short when you require highly tailored and interactive forms that perfectly match your website’s design and functionality. This is where programming comes in.
By delving into programming, you can achieve:
1.1. Full Customization:
When you code your forms, you have complete control over their appearance and behavior. You can ensure that the forms seamlessly blend with your website’s design and offer a consistent user experience.
1.2. Enhanced Functionality:
Programming allows you to implement advanced features that might not be readily available in form plugins. You can integrate conditional logic, real-time validation, and dynamic form fields to create engaging interactions.
1.3. Efficient Data Handling:
Custom-coded forms enable you to handle form submissions and data storage in a way that aligns with your specific requirements. This can lead to better data organization and integration with third-party tools.
2. Getting Started: Prerequisites
Before diving into creating interactive forms, ensure you have the following in place:
2.1. Local Development Environment:
Set up a local development environment using tools like XAMPP, WAMP, or MAMP. This allows you to test your forms without affecting your live website.
2.2. Basic HTML, CSS, and PHP Knowledge:
Familiarity with HTML for creating form structures, CSS for styling, and PHP for processing form submissions is essential.
3. Creating Your First Interactive Form
Let’s start by building a simple contact form with interactive elements step by step.
Step 1: HTML Structure
Begin by creating the HTML structure of your form. Open your text editor and create a new file named contact-form.php. Add the following code:
html <form id="contact-form" method="post" action=""> <label for="name">Name:</label> <input type="text" id="name" name="name" required> <label for="email">Email:</label> <input type="email" id="email" name="email" required> <label for="message">Message:</label> <textarea id="message" name="message" required></textarea> <button type="submit">Submit</button> </form>
In this code snippet, we’ve created a basic form structure with fields for name, email, and message, along with a submit button.
Step 2: Adding CSS Styles
Enhance the visual appeal of your form using CSS. Create a new file named style.css and add the following code:
css #contact-form { width: 400px; margin: 0 auto; padding: 20px; border: 1px solid #ccc; border-radius: 5px; background-color: #f5f5f5; } label { display: block; margin-bottom: 5px; font-weight: bold; } input[type="text"], input[type="email"], textarea { width: 100%; padding: 8px; margin-bottom: 10px; border: 1px solid #ccc; border-radius: 3px; } button { background-color: #007bff; color: #fff; border: none; padding: 10px 20px; border-radius: 3px; cursor: pointer; }
This CSS code styles the form elements, giving your form a polished appearance.
Step 3: Processing Form Data
Now it’s time to process the form data using PHP. Create a new file named process-form.php and add the following code:
php <?php if ($_SERVER["REQUEST_METHOD"] == "POST") { $name = $_POST["name"]; $email = $_POST["email"]; $message = $_POST["message"]; // You can add further processing here, such as sending emails or saving data to a database // For now, let's just display the submitted data echo "Name: $name<br>"; echo "Email: $email<br>"; echo "Message: $message<br>"; } ?>
This PHP script captures the form data and can be extended to perform additional actions like sending emails or storing data.
Step 4: Form Validation with JavaScript
Implement client-side form validation using JavaScript. Create a new file named script.js and add the following code:
javascript document.addEventListener("DOMContentLoaded", function() { const form = document.getElementById("contact-form"); form.addEventListener("submit", function(event) { const name = form.querySelector("#name"); const email = form.querySelector("#email"); const message = form.querySelector("#message"); if (name.value.trim() === "") { alert("Please enter your name."); event.preventDefault(); } if (email.value.trim() === "") { alert("Please enter your email."); event.preventDefault(); } if (message.value.trim() === "") { alert("Please enter a message."); event.preventDefault(); } }); });
This JavaScript code adds client-side validation to the form, ensuring that users complete all required fields before submitting.
4. Taking It Further: Advanced Interactions
Now that you have created a basic interactive form, let’s explore some advanced interactions you can add using programming.
4.1. Conditional Logic:
You can use JavaScript to show or hide form fields based on user selections. For instance, if you’re creating a registration form and the user selects “Other” as their gender, you can dynamically show an additional field for specifying their gender.
4.2. Real-time Validation:
Implement real-time validation as users fill out the form. Display instant feedback on the validity of their inputs. For instance, you can show a green checkmark when a valid email address is entered.
4.3. AJAX Submissions:
Enhance user experience by submitting form data via AJAX, which allows the page to update without a full refresh. This is particularly useful for lengthy forms or forms placed in a popup.
4.4. Custom Styling:
Utilize CSS frameworks like Bootstrap to style your forms beautifully and responsively. You can also experiment with CSS animations to make your forms more engaging.
Conclusion
By combining your WordPress expertise with programming skills, you can create interactive forms that elevate user engagement and tailor the form experience to your website’s unique needs. While form plugins have their place, custom-coded forms provide unparalleled control and flexibility. Remember to start with the basics, gradually introducing more advanced features as you become more comfortable with the process. Whether you’re gathering user feedback, registrations, or inquiries, interactive forms will undoubtedly enhance your WordPress website.
Table of Contents
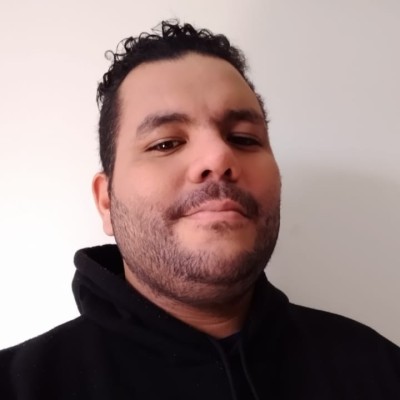
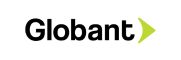