WordPress Multilingual Sites: Translating Content with Programming
If you’re running a WordPress website and aiming to reach a global audience, offering content in multiple languages is essential. Multilingual websites can significantly expand your reach and cater to a diverse audience. While there are many plugins available for translating content in WordPress, sometimes you need more flexibility and control, especially if you’re dealing with custom post types or dynamic content. In this guide, we’ll explore how to create multilingual WordPress sites using programming. We’ll cover translation methods, plugins, and provide you with code samples for a seamless content localization experience.
Table of Contents
1. Why Multilingual Websites Matter
Before diving into the technical aspects of translating content in WordPress, let’s understand why having a multilingual website is crucial:
1.1. Global Reach
English might be a global language, but not everyone prefers it. By offering your content in multiple languages, you can connect with a more diverse audience, potentially increasing your website’s traffic and engagement.
1.2. Better User Experience
Visitors are more likely to stay on your website and explore its content if it’s in their native language. Multilingual sites enhance the user experience and encourage users to spend more time on your pages.
1.3. SEO Benefits
Search engines like Google value content available in multiple languages. A multilingual website can improve your SEO ranking, making it easier for people to find your site through search results.
Now that we understand the importance of multilingual websites, let’s explore how to achieve this using programming.
2. Translation Methods
When it comes to translating content on a WordPress website, you have several methods to consider. Each method has its own pros and cons, and the choice often depends on your specific needs and technical expertise.
2.1. Manual Translation
Manual translation involves creating a separate version of your content for each language you want to support. This method provides complete control over translations but can be time-consuming and challenging to maintain as your site grows.
Here’s an example of manual translation in code:
php <?php // English content $content_en = "Welcome to our website."; // French translation $content_fr = "Bienvenue sur notre site Web."; ?>
2.2. WordPress Core Functions
WordPress offers a set of core functions to assist with content translation. The __() and _e() functions are commonly used for translating strings in your themes and plugins. These functions work well for static content but can be limited when dealing with dynamic content.
Here’s how you can use these functions:
php <?php // Static content translation echo __("Welcome to our website.", "text-domain"); // Dynamic content translation echo sprintf( __("Hello, %s!", "text-domain"), $username ); ?>
2.3. Translation Plugins
There are several translation plugins available for WordPress, such as WPML (WordPress Multilingual) and Polylang. These plugins provide user-friendly interfaces for translating content and managing multiple languages on your site. While they are convenient, they might not offer the level of control you need for custom post types or dynamic content.
3. Translating Content with Programming
To achieve more flexibility and control over content translation, you can use programming. This approach allows you to customize your translation process and handle dynamic content more effectively.
3.1. Creating Multilingual Posts and Pages
One of the most common requirements for multilingual websites is translating posts and pages. You can achieve this by creating custom fields for each language and associating them with your posts or pages. Here’s a code example using custom fields for translations:
php <?php // English content update_post_meta($post_id, 'content_en', "Welcome to our website."); // French translation update_post_meta($post_id, 'content_fr', "Bienvenue sur notre site Web."); ?>
In this example, we’re using the update_post_meta() function to store translations in custom fields. You can then retrieve the appropriate translation based on the user’s language preference.
3.2. Translating Custom Post Types
If your website uses custom post types, you’ll want to ensure they are also available in multiple languages. You can extend the previous approach to handle custom post types by creating custom fields for translations and associating them with the respective post types.
php <?php // English content for custom post type 'portfolio' update_post_meta($post_id, 'portfolio_title_en', "My Portfolio Title"); // French translation for custom post type 'portfolio' update_post_meta($post_id, 'portfolio_title_fr', "Mon Titre de Portefeuille"); ?>
3.3. Handling Dynamic Content
Dealing with dynamic content, such as user-generated content or content pulled from external sources, can be challenging when creating multilingual websites. You can use conditional statements to determine the user’s language preference and display the appropriate content.
Here’s an example of handling dynamic content:
php <?php // Determine the user's language preference $user_language = determine_user_language(); // Display dynamic content based on language if ($user_language === 'en') { echo "Welcome, English-speaking user!"; } elseif ($user_language === 'fr') { echo "Bienvenue, utilisateur francophone!"; } else { echo "Welcome, user!"; } ?>
In this code snippet, we’re using the determine_user_language() function to identify the user’s language preference and display content accordingly.
3.4. Language Switcher
To create a seamless multilingual experience for your users, consider adding a language switcher to your website. This allows users to easily switch between languages and access content in their preferred language. Here’s a simple example of a language switcher in WordPress:
php <ul class="language-switcher"> <li><a href="?lang=en">English</a></li> <li><a href="?lang=fr">Français</a></li> </ul>
You can then use URL parameters like ?lang=en to set the user’s language preference and load the appropriate content.
4. Using Translation Plugins with Programming
While we’ve explored programming-based approaches to content translation in WordPress, it’s worth mentioning that you can also combine these methods with translation plugins for added flexibility.
4.1. WPML Integration
WPML is a popular translation plugin for WordPress. You can use WPML’s API to programmatically interact with translations, making it easier to manage multilingual content. Here’s an example of how to create a translation for a post using WPML’s API:
php <?php // Create a translation for a post $wpml_translation = new WPML_Post_Translation(); $wpml_translation->set_language('fr'); $wpml_translation->set_element_id($post_id); $wpml_translation->set_element_type('post'); $wpml_translation->set_source_language('en'); $wpml_translation->save(); ?>
This code snippet demonstrates how to create a French translation for a post originally written in English using WPML’s API.
4.2. Polylang Integration
Polylang is another translation plugin that can be used in combination with programming. It provides a convenient way to manage translations and switch between languages. To create a translation for a post with Polylang, you can use the following code:
php <?php // Create a translation for a post pll_save_post_translations([ 'fr' => $post_id, // French translation ], true); ?>
This code snippet creates a French translation for a post using Polylang’s functions.
5. Best Practices for Multilingual Programming
When translating content with programming in WordPress, consider the following best practices:
5.1. Use Translation Functions
Whenever you output text in your theme or plugin, use translation functions like __() and _e(). This ensures that your text can be easily translated using plugins or programming methods.
5.2. Keep Content and Code Separated
Separate content from code as much as possible. Store translations in custom fields or database tables rather than hardcoding them into your code. This makes it easier to manage translations and update content without modifying the code.
5.3. Test Extensively
Testing is crucial to ensure that your multilingual website functions as expected. Test different language scenarios, including right-to-left languages, to catch any layout or styling issues.
5.4. Consider SEO
Pay attention to SEO when translating your content. Use proper hreflang tags to indicate language and regional targeting to search engines. This helps improve your website’s visibility in search results.
Conclusion
Creating multilingual WordPress sites with programming provides you with the flexibility and control needed to deliver a seamless multilingual experience to your users. Whether you’re translating posts, pages, custom post types, or handling dynamic content, programming allows you to tailor your approach to your specific needs. Additionally, you can integrate popular translation plugins like WPML or Polylang to enhance your multilingual capabilities further.
By following best practices and testing thoroughly, you can ensure that your multilingual WordPress site reaches a global audience effectively, ultimately expanding your website’s reach and impact. So, start translating your content with programming today and welcome visitors from around the world to your WordPress website.
Table of Contents
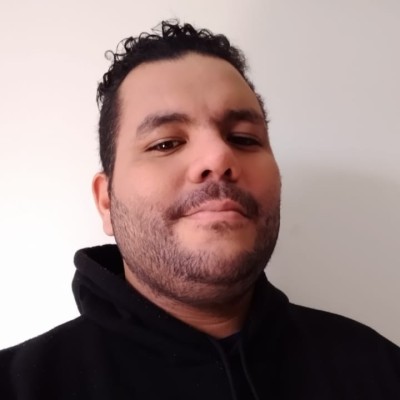
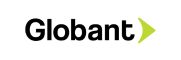