WordPress Custom Fields: Extending Content with Programming
WordPress is a powerhouse when it comes to content management, but sometimes, you need to go beyond the standard editor to create truly dynamic and personalized websites. This is where WordPress Custom Fields come into play. In this blog post, we will explore how you can harness the power of Custom Fields and extend your content with programming. Whether you’re a seasoned developer or a WordPress newbie, this guide will open up a world of possibilities for your website.
Table of Contents
1. Understanding WordPress Custom Fields
1.1. What are Custom Fields?
Custom Fields, also known as post meta or post custom fields, are a way to add extra information to your WordPress posts, pages, or custom post types. They are like hidden gems that can store various types of data, such as text, numbers, dates, or even serialized arrays.
Let’s consider a practical example: You’re running a movie review blog, and you want to display the release year of each movie alongside the review. Instead of cramming this information into the main content, you can use a custom field to store and retrieve the release year for each movie review.
1.2. How to Access Custom Fields
WordPress provides a straightforward way to access Custom Fields:
- Edit or create a post or page in your WordPress dashboard.
- Scroll down to the Custom Fields section, which might be hidden by default. You can usually find it below the main content editor or in the sidebar.
- Here, you can add a new custom field by providing a name (key) and a value (data). In our movie review example, you could name the field “Release Year” and assign it the value “2023.”
- Save or update your post or page.
1.3. Displaying Custom Fields
Displaying Custom Fields on your website requires some programming skills. You’ll need to use template tags or functions to retrieve and output the data. WordPress provides functions like get_post_meta() to fetch custom field values. Here’s a simple code snippet to display the release year from our movie review example:
php <?php $release_year = get_post_meta(get_the_ID(), 'Release Year', true); if (!empty($release_year)) { echo 'Release Year: ' . $release_year; } ?>
In this code:
- get_the_ID() retrieves the current post’s ID.
- ‘Release Year’ is the name (key) of the custom field.
- true ensures that a single value is returned.
2. Extending Content with Custom Fields
Now that you understand the basics of WordPress Custom Fields, let’s explore how you can extend your content and create more dynamic websites using Custom Fields and programming.
2.1. Dynamic Post Templates
Custom Fields allow you to create dynamic post templates. Imagine you have a news website, and you want to display breaking news articles differently from regular news articles. You can use a custom field like “Post Type” to categorize posts and then apply different styles and layouts based on this field.
Here’s a simplified example of how you can achieve this:
php <?php $post_type = get_post_meta(get_the_ID(), 'Post Type', true); if ($post_type === 'Breaking News') { // Apply a different template or styling for breaking news. get_template_part('template-parts/content', 'breaking-news'); } else { // Use the default template for regular news. get_template_part('template-parts/content', 'news'); } ?>
By using Custom Fields to define post types, you can make your website more engaging and user-friendly.
2.2. Personalized Content
Personalization is key to user engagement. You can use Custom Fields to store user preferences or other relevant data and then tailor the content accordingly. For instance, if you run an e-commerce store, you can store a user’s favorite product categories in a custom field and display related products on the homepage.
Here’s a simplified example:
php <?php $user_favorite_categories = get_user_meta(get_current_user_id(), 'Favorite Categories', true); if (!empty($user_favorite_categories)) { // Display personalized content based on user preferences. foreach ($user_favorite_categories as $category) { echo '<h2>' . $category . '</h2>'; // Query and display products from the selected category. } } else { // Display generic content if no preferences are set. echo '<p>Discover our latest products.</p>'; } ?>
This approach makes your website more appealing to users by showing them what they’re most interested in.
2.3. Content Scheduling
You can use Custom Fields to schedule content publication. WordPress already offers a scheduling feature, but with Custom Fields, you can add more granularity and control. For instance, you can create a custom field named “Publication Date” and use it to override the default publication date.
Here’s an example:
php <?php $custom_publication_date = get_post_meta(get_the_ID(), 'Publication Date', true); if (!empty($custom_publication_date)) { // Use the custom publication date if available. $post_date = $custom_publication_date; } else { // Use the default publication date. $post_date = get_the_date(); } echo 'Published on: ' . $post_date; ?>
This approach allows you to plan your content strategy more effectively and ensure that posts go live precisely when you want them to.
2.4. Integrating External Data
Custom Fields can also be used to integrate external data into your WordPress posts. For instance, if you’re running a real estate website, you can use a custom field to store the property’s price and another field to store the property’s location. Then, you can fetch and display this data from an external source or API.
Here’s a simplified example:
php <?php $property_price = get_post_meta(get_the_ID(), 'Property Price', true); $property_location = get_post_meta(get_the_ID(), 'Property Location', true); if (!empty($property_price) && !empty($property_location)) { echo '<p>Price: ' . $property_price . '</p>'; echo '<p>Location: ' . $property_location . '</p>'; } else { echo '<p>Details not available.</p>'; } ?>
This approach enables you to keep your content up to date and synchronized with external data sources automatically.
3. Advanced Techniques with Custom Fields
3.1. Conditional Logic
Custom Fields can be used to implement conditional logic on your website. You can create custom fields that act as flags to control the visibility of certain content elements. For example, you could add a “Featured” custom field to posts and use it to display featured posts prominently on your homepage.
Here’s a simple example:
php <?php $is_featured = get_post_meta(get_the_ID(), 'Featured', true); if ($is_featured === 'yes') { // Display this post as a featured post. echo '<div class="featured-post">...</div>'; } else { // Display regular posts differently. echo '<div class="regular-post">...</div>'; } ?>
This approach empowers you to create dynamic layouts and highlight specific content based on custom criteria.
3.2. Custom Fields in Custom Themes
If you’re developing a custom WordPress theme, you can leverage Custom Fields extensively to provide users with an intuitive interface for customizing their website. Custom Fields can be used to add options for changing colors, fonts, layouts, and more, directly from the WordPress dashboard.
Here’s a simplified example of how you can create a color customization option using Custom Fields:
php <?php $custom_background_color = get_post_meta(get_the_ID(), 'Background Color', true); if (!empty($custom_background_color)) { echo '<style>'; echo 'body { background-color: ' . $custom_background_color . '; }'; echo '</style>'; } ?>
This approach allows non-technical users to personalize their website’s appearance without touching a single line of code.
4. Best Practices for Using Custom Fields
Before you dive into using Custom Fields, it’s essential to follow best practices to ensure your website remains efficient and maintainable:
4.1. Use Descriptive Names
Give your Custom Fields meaningful names that reflect their purpose. This makes it easier for you and other users to understand their function.
4.2. Avoid Overloading
While Custom Fields are powerful, avoid overloading your posts or pages with too many of them. Excessive Custom Fields can clutter the editing interface.
4.3. Sanitize and Validate Data
When working with Custom Fields, validate and sanitize the data to prevent security vulnerabilities or unexpected behavior.
4.4. Backup Your Data
Regularly backup your WordPress site, including Custom Fields data, to prevent data loss.
Conclusion
WordPress Custom Fields are a versatile tool that can take your website to the next level. By combining Custom Fields with programming, you can create dynamic, personalized, and feature-rich websites that cater to your audience’s specific needs. Whether you’re looking to build a news site with custom templates, personalize content, schedule posts, integrate external data, or implement advanced techniques, Custom Fields provide you with the flexibility to achieve your goals. Just remember to follow best practices to ensure a smooth and efficient development process. Start exploring the world of Custom Fields, and unlock the full potential of your WordPress website today.
Table of Contents
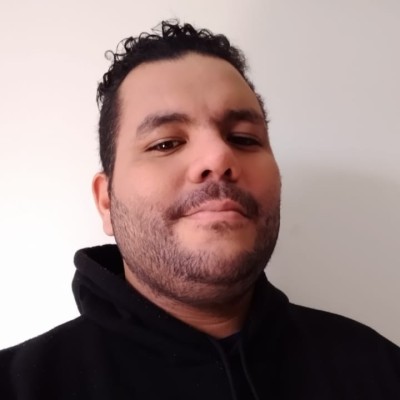
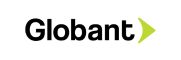