Extending WordPress Functionality with Custom Post Types and Taxonomies
WordPress is one of the most popular content management systems (CMS) globally, powering millions of websites across various industries. Its flexibility and ease of use make it a top choice for bloggers, businesses, and developers alike. While WordPress comes equipped with built-in post types like posts and pages, it also allows developers to create custom post types and taxonomies, enabling them to extend the platform’s functionality and organize content in a more structured manner.
In this blog post, we will delve into the world of custom post types and taxonomies, exploring how they can enhance your WordPress site’s capabilities and help you build more dynamic and customized websites. We’ll cover the basics, provide step-by-step guides, and share code samples to empower you to create your custom content types and taxonomies efficiently.
1. Understanding Custom Post Types
WordPress organizes content using post types, and by default, it provides two primary post types – “posts” for blog posts and “pages” for static pages. However, custom post types allow you to define and create unique content structures tailored to your specific needs.
1.1 Creating a Custom Post Type
To create a custom post type, you’ll need to use the register_post_type() function. Let’s say we want to create a “Portfolio” post type for showcasing our projects. Here’s the code snippet to achieve this:
php // functions.php or a custom plugin file function create_portfolio_post_type() { $labels = array( 'name' => _x('Portfolio', 'post type general name', 'textdomain'), 'singular_name' => _x('Portfolio', 'post type singular name', 'textdomain'), 'menu_name' => _x('Portfolio', 'admin menu', 'textdomain'), 'name_admin_bar' => _x('Portfolio', 'add new on admin bar', 'textdomain'), 'add_new' => _x('Add New', 'portfolio', 'textdomain'), 'add_new_item' => __('Add New Portfolio Item', 'textdomain'), 'new_item' => __('New Portfolio Item', 'textdomain'), 'edit_item' => __('Edit Portfolio Item', 'textdomain'), 'view_item' => __('View Portfolio Item', 'textdomain'), 'all_items' => __('All Portfolio Items', 'textdomain'), 'search_items' => __('Search Portfolio Items', 'textdomain'), 'parent_item_colon' => __('Parent Portfolio Items:', 'textdomain'), 'not_found' => __('No portfolio items found.', 'textdomain'), 'not_found_in_trash' => __('No portfolio items found in Trash.', 'textdomain') ); $args = array( 'labels' => $labels, 'public' => true, 'publicly_queryable' => true, 'show_ui' => true, 'show_in_menu' => true, 'query_var' => true, 'rewrite' => array('slug' => 'portfolio'), 'capability_type' => 'post', 'has_archive' => true, 'hierarchical' => false, 'menu_position' => null, 'supports' => array('title', 'editor', 'thumbnail', 'excerpt', 'custom-fields') ); register_post_type('portfolio', $args); } add_action('init', 'create_portfolio_post_type');
In the above code, we use the register_post_type() function to define various attributes of our custom post type, such as labels, capabilities, and supported features like the title, editor, thumbnail, etc. Adjust these parameters according to your requirements.
2. Organizing Content with Custom Taxonomies
Custom taxonomies allow you to group and categorize content across different custom post types. They are similar to WordPress categories or tags but provide more flexibility in organizing your content effectively.
2.1 Creating a Custom Taxonomy
Let’s create a custom taxonomy called “Project Categories” for our “Portfolio” post type to categorize projects. We’ll use the register_taxonomy() function to achieve this:
php // functions.php or a custom plugin file function create_project_categories_taxonomy() { $labels = array( 'name' => _x('Project Categories', 'taxonomy general name', 'textdomain'), 'singular_name' => _x('Project Category', 'taxonomy singular name', 'textdomain'), 'search_items' => __('Search Project Categories', 'textdomain'), 'all_items' => __('All Project Categories', 'textdomain'), 'parent_item' => __('Parent Project Category', 'textdomain'), 'parent_item_colon' => __('Parent Project Category:', 'textdomain'), 'edit_item' => __('Edit Project Category', 'textdomain'), 'update_item' => __('Update Project Category', 'textdomain'), 'add_new_item' => __('Add New Project Category', 'textdomain'), 'new_item_name' => __('New Project Category Name', 'textdomain'), 'menu_name' => __('Project Categories', 'textdomain'), ); $args = array( 'labels' => $labels, 'hierarchical' => true, 'show_admin_column' => true, 'query_var' => true, 'rewrite' => array('slug' => 'project-category'), ); register_taxonomy('project_category', 'portfolio', $args); } add_action('init', 'create_project_categories_taxonomy');
In this code, we use the register_taxonomy() function to create the “Project Categories” custom taxonomy. Note that we associate this taxonomy with the “Portfolio” post type using the register_taxonomy() function’s third argument.
3. Displaying Custom Post Types and Taxonomies
Now that we have created our custom post type and taxonomy, let’s explore how to display them on the front-end.
3.1 Displaying Custom Post Type Archive
To display the archive page for our “Portfolio” custom post type, we’ll need to create a custom template file named archive-portfolio.php in our theme’s folder or child theme’s folder. In this file, we can use the standard WordPress loop to list the portfolio items:
php <?php /** * Template Name: Portfolio Archive */ get_header(); ?> <main id="primary" class="site-main"> <section class="portfolio-items"> <?php if (have_posts()) : while (have_posts()) : the_post(); ?> <article id="post-<?php the_ID(); ?>" <?php post_class(); ?>> <h2><?php the_title(); ?></h2> <?php the_excerpt(); ?> <?php the_post_thumbnail(); ?> </article> <?php endwhile; else : ?> <p><?php _e('No portfolio items found.', 'textdomain'); ?></p> <?php endif; ?> </section> </main> <?php get_footer();
In the above template file, we use the WordPress loop to display each portfolio item’s title, excerpt, and thumbnail. You can customize the HTML structure and styling to fit your theme’s design.
3.2 Displaying Custom Taxonomy Terms
To display the “Project Categories” custom taxonomy terms, we can use the get_terms() function in our theme’s template file:
php <?php $project_categories = get_terms(array( 'taxonomy' => 'project_category', 'hide_empty' => false, )); ?> <ul class="project-categories"> <?php foreach ($project_categories as $category) : ?> <li> <a href="<?php echo get_term_link($category); ?>"><?php echo $category->name; ?></a> </li> <?php endforeach; ?> </ul>
In this code, we retrieve all the “Project Categories” terms using the get_terms() function and then loop through each term to display its name and link to its archive page.
4. Leveraging Custom Post Types and Taxonomies for Plugins
Custom post types and taxonomies aren’t just for themes; they can be incredibly powerful when used in plugins. Let’s look at an example of how a custom post type and taxonomy could be used in a plugin.
For instance, let’s create a simple plugin called “Testimonials” that allows users to add and manage testimonials as custom post types. Additionally, we’ll create a “Testimonial Categories” custom taxonomy to categorize these testimonials.
php <?php /** * Plugin Name: Testimonials * Description: A simple plugin to manage testimonials. */ // Register Testimonials Post Type function create_testimonials_post_type() { $labels = array( 'name' => _x('Testimonials', 'post type general name', 'textdomain'), 'singular_name' => _x('Testimonial', 'post type singular name', 'textdomain'), 'menu_name' => _x('Testimonials', 'admin menu', 'textdomain'), // ... ); $args = array( 'labels' => $labels, 'public' => true, // ... ); register_post_type('testimonial', $args); } add_action('init', 'create_testimonials_post_type'); // Register Testimonial Categories Taxonomy function create_testimonial_categories_taxonomy() { $labels = array( 'name' => _x('Testimonial Categories', 'taxonomy general name', 'textdomain'), 'singular_name' => _x('Testimonial Category', 'taxonomy singular name', 'textdomain'), // ... ); $args = array( 'labels' => $labels, 'hierarchical' => true, // ... ); register_taxonomy('testimonial_category', 'testimonial', $args); } add_action('init', 'create_testimonial_categories_taxonomy');
In the above code, we’ve created a basic plugin that registers a “Testimonials” custom post type and a “Testimonial Categories” custom taxonomy. Users can now manage testimonials separately from regular posts, making it easier to handle different types of content.
Conclusion
WordPress custom post types and taxonomies are incredibly powerful tools for extending the functionality of your website. Whether you’re building a portfolio site, a membership site, or an e-commerce store, custom post types and taxonomies enable you to organize and display content in a more structured and tailored way.
In this blog post, we’ve covered the fundamentals of creating custom post types and taxonomies, along with code samples to get you started. Now, armed with this knowledge, you have the potential to build more dynamic and customized websites with WordPress.
Remember, the key is to analyze your site’s unique content requirements and determine how custom post types and taxonomies can best serve your purpose. By leveraging these features effectively, you can unlock the full potential of WordPress and take your website to new heights. Happy coding!
Table of Contents
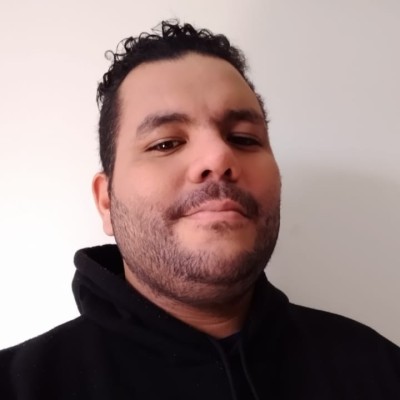
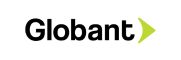