Customizing the WordPress Media Library with Programming
The WordPress Media Library is the heart of your website’s visual content. It’s where you store, manage, and organize images, videos, and other media files. While WordPress provides a user-friendly interface, sometimes you need more control and customization to streamline your workflow. That’s where programming comes into play.
Table of Contents
In this comprehensive guide, we’ll delve into various aspects of customizing the WordPress Media Library using programming. Whether you’re a developer looking to extend functionality or a website owner aiming to enhance media management, we’ve got you covered.
1. Why Customize the Media Library?
Before we dive into the technical details, let’s understand why customizing the WordPress Media Library can be a game-changer for your website:
1.1. Efficient Media Management
As your website grows, so does your media library. Customization allows you to implement features like bulk editing, tagging, and categorization, making it easier to find and organize files.
1.2. Improved User Experience
Tailoring the media library to your specific needs can enhance the user experience. You can create user-friendly interfaces for your content creators, making it simpler for them to add and manage media.
1.3. Optimized Performance
Custom code can help optimize your media library’s performance. You can implement lazy loading, image compression, and other techniques to ensure fast loading times.
1.4. Enhanced SEO
By customizing image attributes and metadata, you can boost your website’s SEO. This can help improve your search engine rankings and drive more organic traffic.
1.5. Integration with Third-Party Services
Programming allows you to integrate your media library with third-party services like cloud storage, CDN providers, and image optimization tools, expanding your website’s capabilities.
Now that you know why customization is essential, let’s explore the various ways to enhance your WordPress Media Library using programming.
2. Customization Methods
There are several methods to customize the WordPress Media Library, each catering to different needs and skill levels:
2.1. Using Plugins
The easiest way to customize the Media Library is by using plugins. There are numerous plugins available that offer various functionalities, from bulk editing to advanced media organization. Some popular options include:
- Media Library Plus: This plugin adds extra features like the ability to create folders, move files, and filter media by date.
- Enhanced Media Library: It allows you to categorize media into folders and subfolders, making it easier to locate files.
- Media Cleaner: This plugin helps you clean up your media library by detecting and deleting unused files.
Plugins are an excellent choice if you don’t have coding skills, but they may not provide the level of customization you desire.
2.2. Custom CSS
If you’re comfortable with CSS, you can make visual changes to the Media Library. For example, you can adjust the layout, fonts, and colors to match your website’s branding. To do this, add custom CSS rules to your theme’s stylesheet or use a plugin like “Simple Custom CSS.”
Here’s an example of custom CSS to change the background color of the Media Library:
css /* Change Media Library background color */ #wp-media-grid { background-color: #f0f0f0; }
While CSS customizations are relatively simple, they are limited to visual changes and won’t provide advanced functionality.
2.3. Custom JavaScript
To add interactive features and functionality to the Media Library, you can use custom JavaScript code. This method requires more technical knowledge but offers greater flexibility.
For instance, you can create a JavaScript script that adds a button to the Media Library to perform a specific action, such as resizing all images to a standard size before insertion.
Here’s a simplified example:
javascript // Add a custom button to the Media Library jQuery(document).ready(function($) { $('.media-frame-content').on('click', '.custom-button', function() { // Your custom code here alert('Custom action executed!'); }); });
Custom JavaScript enables you to tailor the Media Library’s behavior to your exact requirements.
2.4. Custom Plugins and Themes
For the highest level of customization, consider creating custom WordPress plugins or themes. This method is suitable for developers with coding skills and allows you to build tailored solutions that integrate seamlessly with your website.
2.4.1. Custom Plugins
Creating a custom plugin for your media library is a powerful way to add unique features. For instance, you can develop a plugin that automatically tags uploaded images based on their content.
Here’s a simplified example of a custom plugin:
php // Custom plugin to auto-tag images function auto_tag_images($attachment_id) { // Your custom tagging logic here wp_set_post_tags($attachment_id, 'nature', true); } // Hook into the media library upload process add_action('add_attachment', 'auto_tag_images');
2.4.2. Custom Themes
If you want to integrate media library customization with your theme, you can create a custom theme. This allows you to control the library’s appearance and behavior entirely.
For instance, you can design a theme that displays media files in a masonry layout, making your media library more visually appealing.
Custom themes offer complete control but require in-depth coding skills.
3. Essential Customizations with Code Samples
Let’s explore some essential customizations you can achieve with code samples.
3.1. Bulk Image Compression
Large image files can slow down your website. You can use code to automatically compress uploaded images without compromising quality.
Here’s a sample code snippet using the jpegoptim library to compress JPEG images upon upload:
php function compress_uploaded_images($attachment_id) { $file_path = get_attached_file($attachment_id); if (wp_attachment_is_image($attachment_id)) { exec("jpegoptim --max=80 --strip-all $file_path"); } } add_action('add_attachment', 'compress_uploaded_images');
This code hooks into the attachment upload process and compresses JPEG images using jpegoptim with a maximum quality of 80.
3.2. Media Taxonomies
WordPress doesn’t natively support taxonomies for media files, but you can add this feature with code.
Here’s an example of registering a custom taxonomy called “Media Categories” for media attachments:
php function register_media_taxonomy() { $args = array( 'hierarchical' => true, 'labels' => array( 'name' => 'Media Categories', 'singular_name' => 'Media Category', ), 'show_ui' => true, 'show_admin_column' => true, ); register_taxonomy('media_category', 'attachment', $args); } add_action('init', 'register_media_taxonomy');
With this code, you can categorize media files just like you would with posts or pages.
3.3. Custom Columns in Media Library
You can add custom columns to the Media Library to display additional information about your media files. For instance, you can display the image dimensions or the date it was uploaded.
Here’s a code snippet to add a “Dimensions” column:
php function add_dimensions_column($columns) { $columns['dimensions'] = 'Dimensions'; return $columns; } function populate_dimensions_column($column_name, $attachment_id) { if ($column_name == 'dimensions') { $image_data = wp_get_attachment_image_src($attachment_id, 'full'); echo $image_data[1] . 'x' . $image_data[2]; } } add_filter('manage_media_columns', 'add_dimensions_column'); add_action('manage_media_custom_column', 'populate_dimensions_column', 10, 2);
This code adds a “Dimensions” column to the Media Library, displaying the width and height of each image.
3.4. Media Library Shortcodes
Create custom shortcodes to embed media files anywhere on your site. This is useful when you want to showcase specific media items in posts or pages.
Here’s an example shortcode to embed a specific image by its attachment ID:
php function custom_media_shortcode($atts) { $atts = shortcode_atts(array( 'id' => '', ), $atts); if (empty($atts['id'])) { return; } $image_url = wp_get_attachment_image_url($atts['id'], 'full'); if (!$image_url) { return; } return '<img src="' . esc_url($image_url) . '" alt="Custom Image" />'; } add_shortcode('custom_media', 'custom_media_shortcode');
You can use this shortcode in your posts or pages like this: [custom_media id=”123″].
4. Going Beyond: Advanced Customization
The customizations we’ve discussed so far are just the tip of the iceberg. Depending on your requirements, you can dive deeper into customization by exploring these advanced topics:
4.1. Media Library API
WordPress provides a robust Media Library API that allows you to interact with media files programmatically. You can use it to create custom interfaces for media management, automate tasks, and integrate external services seamlessly.
4.2. Custom Media Uploaders
Develop custom media uploaders tailored to your specific needs. For example, you can create an uploader that directly connects to your cloud storage or incorporates advanced image editing features.
4.3. Advanced Image Processing
Implement advanced image processing techniques such as face detection, image recognition, and watermarking using libraries like OpenCV or custom solutions.
Conclusion
Customizing the WordPress Media Library with programming opens up a world of possibilities for improving your website’s media management, user experience, and performance. Whether you’re a developer or a website owner, you can leverage code to take control of your media assets and enhance your WordPress-powered site.
Remember that with great power comes great responsibility. Always backup your website before implementing customizations and thoroughly test them in a safe environment to ensure they work as expected. Happy customizing!
Table of Contents
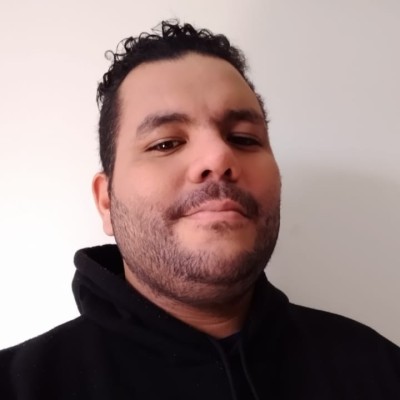
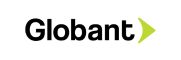