Customizing the WordPress Comment System with Programming
When it comes to running a successful blog or website, fostering user engagement is crucial. One of the most effective ways to achieve this is through an interactive comment system. WordPress, the popular content management system, provides a built-in comment feature, but often, site owners want to take it a step further by customizing it with programming. In this comprehensive guide, we will explore how you can tailor the WordPress comment system to meet your unique requirements, from enhancing user experience to implementing advanced moderation techniques.
Table of Contents
1. Why Customize the Comment System?
Before diving into the technical details of customizing the WordPress comment system, it’s essential to understand why you might want to undertake this endeavor.
1.1. Improved User Experience
A vanilla comment system might not always align with your website’s design and functionality. By customizing it, you can provide users with a more seamless and engaging commenting experience. Tailoring the comment system to match your site’s aesthetics and user interface can make users feel more at home, increasing their likelihood to engage with your content.
1.2. Enhanced Moderation
Moderation is essential to maintain a healthy online community. Customization allows you to implement advanced moderation techniques, such as automated content filtering and user role-based comment permissions. This way, you can ensure that your comment section remains a safe and constructive space for discussions.
1.3. Unique Branding
Your website is an extension of your brand. Customizing the comment system enables you to incorporate your brand’s visual elements and style into the comment section, making it a more integral part of your site’s identity.
2. Customization Basics
Before we dive into specific customization techniques, let’s establish some fundamental concepts.
2.1. Understanding the WordPress Comment Template
WordPress uses a template system to display comments. This template is typically located in your theme’s folder and is named comments.php. This file controls how comments are displayed and styled on your site. To start customizing your comment system, you’ll need to modify this template.
2.2. Child Themes: Your Customization Playground
When customizing WordPress, it’s advisable to create a child theme. A child theme inherits the functionality and styling of the parent theme, allowing you to make modifications without altering the original theme files. This ensures that your customizations won’t be lost when the parent theme is updated.
php // Example of creating a child theme in WordPress /* Theme Name: My Custom Theme Template: parent-theme-folder */
3. Enhancing User Experience
Let’s explore some ways to enhance the user experience within the WordPress comment system.
3.1. AJAX-Powered Comment Loading
AJAX (Asynchronous JavaScript and XML) can be used to load comments without requiring a page refresh. This provides a smoother and more dynamic user experience, similar to popular social media platforms.
Here’s a simplified example of how to load comments via AJAX:
javascript // JavaScript to load comments via AJAX jQuery(function($) { $('.load-comments-button').click(function() { $.ajax({ url: ajaxurl, // WordPress AJAX handler type: 'GET', data: { action: 'load_comments', post_id: 123 // Replace with your post ID }, success: function(response) { $('.comments-container').html(response); } }); }); });
In your theme’s functions.php, you would need to create the corresponding AJAX action:
php // PHP to handle the AJAX request function load_comments() { $post_id = $_GET['post_id']; // Fetch and display comments for the given post ID // Return the HTML content die(); } add_action('wp_ajax_load_comments', 'load_comments'); add_action('wp_ajax_nopriv_load_comments', 'load_comments');
This code enables you to load comments dynamically without reloading the entire page, providing a more engaging user experience.
3.2. Nested Comments for Improved Threads
WordPress supports nested (threaded) comments by default, but you can enhance their styling and functionality. Improving the visual hierarchy and adding features like reply buttons can encourage more in-depth discussions.
css /* CSS to style nested comments */ .comment.depth-1 { margin-left: 20px; } .comment-reply-link { color: #0073e6; text-decoration: underline; }
This CSS code adjusts the margin for nested comments and styles the reply link. You can customize it to match your site’s design.
3.3. Comment Voting System
Implementing a comment voting system can boost user engagement. Users can upvote or downvote comments, promoting quality content and discouraging spam or low-value comments.
php // Example code for a comment voting system function add_comment_votes() { // Register a custom field to store vote counts register_comment_meta('comment', 'vote_count', [ 'type' => 'integer', 'show_in_rest' => true, 'single' => true, 'default' => 0, ]); } add_action('init', 'add_comment_votes');
With this custom field, you can create voting buttons and update the vote count for each comment accordingly.
javascript // JavaScript for comment voting jQuery(function($) { $('.upvote-button').click(function() { var commentID = $(this).data('comment-id'); $.post(ajaxurl, { action: 'upvote_comment', comment_id: commentID, }, function(response) { // Update the vote count display }); }); // Similar code for downvoting });
In your functions.php, handle the vote actions:
php // PHP to handle comment upvotes function upvote_comment() { $comment_id = $_POST['comment_id']; // Update the vote count for the comment // Return the updated count die(); } add_action('wp_ajax_upvote_comment', 'upvote_comment'); add_action('wp_ajax_nopriv_upvote_comment', 'upvote_comment');
This example demonstrates the basics of implementing a comment voting system. You can further enhance it with features like vote limits and user reputation.
4. Advanced Moderation Techniques
Moderation is a crucial aspect of managing comments on your website. Customization allows you to implement advanced moderation techniques to maintain a healthy comment section.
4.1. Auto-Moderation with AI
Automated content filtering using artificial intelligence can help identify and flag potentially inappropriate or spammy comments. Services like Perspective API or Akismet can be integrated into your comment system to achieve this.
php // Example code for integrating Akismet function custom_comment_moderation($approved, $comment_data) { $comment_content = $comment_data['comment_content']; // Use Akismet to check the comment $is_spam = custom_akismet_check($comment_content); if ($is_spam) { return 'spam'; } return $approved; } add_filter('pre_comment_approved', 'custom_comment_moderation', 10, 2);
4.2. Custom Comment Reporting
Empower your users to report inappropriate comments with a custom reporting system. Create a “Report” button for each comment and implement a mechanism for handling reports.
php // Example code for custom comment reporting function report_comment() { $comment_id = $_POST['comment_id']; $reporter_email = $_POST['reporter_email']; $reason = $_POST['reason']; // Log the report and send an email to site administrators // Implement your reporting logic here die(); } add_action('wp_ajax_report_comment', 'report_comment'); add_action('wp_ajax_nopriv_report_comment', 'report_comment');
4.3. Role-Based Comment Permissions
WordPress allows you to define user roles with different capabilities. Leverage this feature to assign specific comment moderation duties to different user roles. For example, you can give moderators the ability to approve or delete comments, while regular users can only comment.
php // Example code for role-based comment permissions function custom_comment_permissions($caps, $cap, $user_id, $args) { // Check the user's role and adjust capabilities accordingly if (is_moderator($user_id)) { $caps['moderate_comments'] = true; } return $caps; } add_filter('user_has_cap', 'custom_comment_permissions', 10, 4);
This code snippet modifies comment-related capabilities based on user roles.
5. Unique Branding
Make your comment section an integral part of your site’s branding by customizing its appearance and functionality.
5.1. Styling Comments to Match Your Brand
Use CSS to style comments, avatars, and user details in a way that aligns with your site’s branding. You can customize fonts, colors, and spacing to create a cohesive look.
css /* CSS for custom comment styling */ .comment { background-color: #f9f9f9; border: 1px solid #ddd; padding: 10px; margin-bottom: 20px; } .comment .avatar { border-radius: 50%; margin-right: 10px; } .comment .comment-author { font-weight: bold; color: #0073e6; }
5.2. Custom Avatars and User Profiles
Give users the option to upload custom avatars or profile pictures. You can use the WordPress Media Library or third-party services like Gravatar.
php // Example code for custom avatars function custom_avatar($avatar, $id_or_email, $size, $default, $alt) { // Implement your custom avatar logic here } add_filter('get_avatar', 'custom_avatar', 10, 5);
5.3. Implementing Rich Media Comments
Enhance the interactivity of your comment section by allowing users to embed rich media, such as images, videos, and GIFs. Plugins like “Enable Media Replace” can help with this customization.
6. Security Considerations
When customizing the comment system, it’s crucial to consider security to protect your site and users.
6.1. Protecting Against Comment Spam
Comment spam can harm your site’s reputation and user experience. Implement CAPTCHA or reCAPTCHA to deter automated spam submissions.
php // Example code for adding reCAPTCHA to comments function add_recaptcha_to_comments() { // Implement reCAPTCHA integration here } add_action('comment_form_after_fields', 'add_recaptcha_to_comments');
6.2. Securing Comment Data
Ensure that sensitive comment data is stored securely. Implement measures to prevent SQL injection and data breaches, such as using prepared statements when interacting with the database.
7. Testing and Maintenance
Before deploying your custom comment system, thoroughly test it on a staging site to ensure it functions as intended. Regularly update your customizations to remain compatible with WordPress updates and security best practices.
Conclusion
Customizing the WordPress comment system with programming can greatly enhance user engagement, improve moderation, and align the comment section with your site’s branding. By following the techniques and best practices outlined in this guide, you can create a unique and interactive commenting experience for your website visitors. Experiment, iterate, and watch your online community thrive with a tailored comment system.
Table of Contents
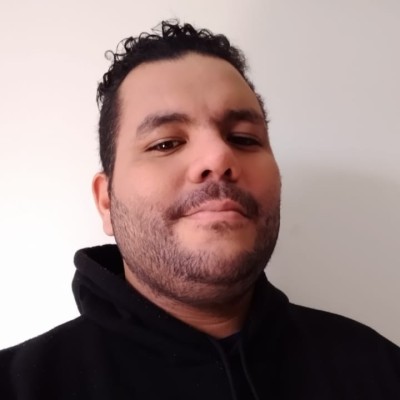
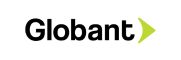