Customizing the WordPress Dashboard with Programming
The WordPress dashboard serves as the nerve center of your website, providing you with a comprehensive overview of your site’s performance, content, and settings. While the default dashboard is functional, you might find that customizing it can greatly enhance your workflow and provide a more tailored experience. In this article, we’ll delve into the world of dashboard customization using programming techniques, exploring various ways to make the dashboard truly your own.
Table of Contents
1. Why Customize the Dashboard?
The default WordPress dashboard layout is designed to cater to a broad range of users, which might not always align perfectly with your specific needs. By customizing the dashboard, you can declutter the interface, bring frequently used tools to the forefront, and create a more intuitive and productive environment for managing your website.
2. Getting Started: Creating a Child Theme
Before we dive into the customization process, it’s crucial to establish a solid foundation. This involves creating a child theme, which is a safer way to make changes to your WordPress site without affecting the core files. To create a child theme, follow these steps:
- Create a New Folder: In your WordPress themes directory, create a new folder for your child theme.
- Create Stylesheet: Within the child theme folder, create a stylesheet named style.css. In this file, define the necessary information, such as the theme name and the parent theme.
css /* Theme Name: Your Child Theme Name Template: parent-theme-folder-name */
- Activate the Child Theme: Go to your WordPress admin panel and navigate to “Appearance” > “Themes.” You’ll see your newly created child theme listed – activate it.
3. Customizing the Dashboard Layout
One of the most noticeable aspects of the WordPress dashboard is its layout. By reorganizing widgets and adding new ones, you can create a dashboard that caters to your specific tasks and preferences. Here’s how:
3.1. Removing Unnecessary Widgets:
To clean up your dashboard, remove widgets that you rarely use. Add the following code to your child theme’s functions.php file to disable specific dashboard widgets:
php function custom_remove_dashboard_widgets() { // Remove 'Quick Draft' widget remove_meta_box('dashboard_quick_press', 'dashboard', 'side'); // Remove 'Welcome' widget remove_action('welcome_panel', 'wp_welcome_panel'); } add_action('wp_dashboard_setup', 'custom_remove_dashboard_widgets');
3.2. Adding Custom Widgets:
You can also add custom widgets to your dashboard to display relevant information. For example, if you’re managing an e-commerce site, you might want to display recent orders or sales statistics. Use the following code as a starting point:
php function custom_dashboard_widget() { echo '<div class="custom-dashboard-widget">'; echo '<h2>Recent Orders</h2>'; // Display your custom content here echo '</div>'; } function add_custom_dashboard_widget() { wp_add_dashboard_widget( 'custom_dashboard_widget', 'Custom Dashboard Widget', 'custom_dashboard_widget' ); } add_action('wp_dashboard_setup', 'add_custom_dashboard_widget');
4. Customizing Admin Color Scheme
Aesthetic preferences vary, and the default WordPress admin color scheme might not resonate with your brand or personal taste. Luckily, you can easily customize the admin color scheme using code:
4.1. Enqueue Custom Stylesheet:
Create a new CSS file for your admin color scheme and enqueue it in your child theme’s functions.php:
php function custom_admin_style() { wp_enqueue_style('admin-styles', get_stylesheet_directory_uri() . '/admin-style.css'); } add_action('admin_enqueue_scripts', 'custom_admin_style');
4.2. Define Custom Styles:
In your admin-style.css file, define your preferred color scheme. You can customize various dashboard elements, including headers, buttons, and backgrounds:
css /* Example admin-style.css */ /* Customize the admin header */ #wpadminbar { background-color: #333; color: #fff; } /* Customize button styles */ .button-primary { background-color: #ff9900; border-color: #ff9900; }
5. Adding Dashboard Widgets with External Data
To take your dashboard customization to the next level, consider adding widgets that display external data. For instance, you might want to show your latest social media posts or analytics. Here’s how to do it:
5.1. Retrieve External Data:
Using the relevant APIs, retrieve the data you want to display. For example, to display recent tweets, you would need to interact with the Twitter API.
5.2. Display Data in a Widget:
Create a custom widget to display the external data. This involves integrating the API calls within your widget’s code. Here’s a simplified example:
php function custom_external_data_widget() { // Make API calls and retrieve data $tweets = fetch_recent_tweets(); // Display the data in the widget echo '<div class="custom-external-data-widget">'; echo '<h2>Recent Tweets</h2>'; foreach ($tweets as $tweet) { echo '<p>' . $tweet->text . '</p>'; } echo '</div>'; } function add_custom_external_data_widget() { wp_add_dashboard_widget( 'custom_external_data_widget', 'External Data Widget', 'custom_external_data_widget' ); } add_action('wp_dashboard_setup', 'add_custom_external_data_widget');
Conclusion
Customizing the WordPress dashboard with programming offers a world of possibilities for tailoring your website management experience. By creating a child theme, reorganizing and adding widgets, adjusting the admin color scheme, and incorporating external data, you can transform the default dashboard into a powerful and personalized hub that aligns perfectly with your needs. Remember that each customization should be approached thoughtfully, prioritizing usability and efficiency to create a dashboard that empowers you to manage your website seamlessly. So, dive in, experiment, and elevate your WordPress experience to new heights.
Table of Contents
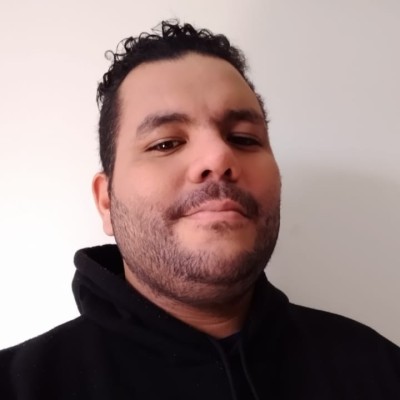
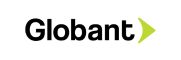