Managing Database Operations with WordPress Programming
WordPress is renowned for its versatility as a content management system (CMS) that empowers users to create and manage websites with ease. Behind the scenes, WordPress relies heavily on a database to store and retrieve content, configurations, and user data. Understanding how to manage database operations is crucial for maintaining the performance, security, and overall functionality of your WordPress website. In this article, we will delve into the intricacies of managing database operations using WordPress programming. We will explore various techniques, provide code samples, and discuss best practices to ensure your WordPress site runs smoothly.
Table of Contents
1. Understanding WordPress Database Structure:
Before diving into managing database operations, it’s essential to grasp the structure of the WordPress database. WordPress employs a MySQL database to store information in various tables. Key tables include:
- wp_posts: This table stores posts, pages, and custom post types, containing data such as titles, content, and timestamps.
- wp_users: User-related information, including usernames, passwords, and email addresses, is stored in this table.
- wp_comments: Comments on posts are stored here, along with relevant metadata.
- wp_terms: Taxonomies, such as categories and tags, are managed within this table.
- wp_options: Configuration settings and various options are stored in this table.
2. CRUD Operations in WordPress:
CRUD operations (Create, Read, Update, Delete) are fundamental for managing database data. WordPress provides APIs and functions to interact with the database seamlessly.
2.1. Creating Data:
To add new content to your WordPress site, you can use functions like wp_insert_post() to create posts programmatically. For instance:
php $new_post = array( 'post_title' => 'New Post Title', 'post_content' => 'This is the content of the new post.', 'post_status' => 'publish', 'post_author' => 1, 'post_category'=> array(2, 4) // Categories IDs ); // Insert the post into the database $new_post_id = wp_insert_post($new_post);
2.2. Reading Data:
Retrieving data is a common operation in WordPress. Functions like get_posts() and WP_Query enable you to fetch posts based on specific criteria. For instance:
php $args = array( 'post_type' => 'post', 'post_status' => 'publish', 'posts_per_page' => 10, 'orderby' => 'date', 'order' => 'DESC' ); $posts = get_posts($args); foreach ($posts as $post) { // Process each post }
2.3. Updating Data:
WordPress provides functions like wp_update_post() to modify existing posts. For example:
php $updated_post = array( 'ID' => 42, // Post ID to update 'post_title' => 'Updated Title', 'post_content' => 'Updated content.', ); wp_update_post($updated_post);
2.4. Deleting Data:
Deleting data is straightforward with functions like wp_delete_post(). Here’s how you could delete a post:
php $post_id_to_delete = 25; wp_delete_post($post_id_to_delete, true); // Set the second parameter to true to force delete
3. Database Queries and wpdb Class:
For more complex database operations, WordPress provides the wpdb class, which offers a safe and efficient way to interact with the database using SQL queries.
3.1. Basic Query:
The wpdb class allows you to perform custom queries. Here’s an example of fetching all post titles:
php global $wpdb; $query = "SELECT ID, post_title FROM {$wpdb->posts} WHERE post_type = 'post' AND post_status = 'publish'"; $results = $wpdb->get_results($query); foreach ($results as $result) { echo $result->post_title . '<br>'; }
3.2. Prepared Statements:
Prepared statements enhance security by preventing SQL injection attacks. The prepare() method is used to create a safe query:
php $title = 'Sample Title'; $content = 'Sample Content'; $query = $wpdb->prepare("INSERT INTO {$wpdb->posts} (post_title, post_content) VALUES (%s, %s)", $title, $content); $wpdb->query($query);
4. Database Optimization:
Efficient database management leads to better website performance. Consider the following practices:
- Indexing: Properly indexing database tables improves query performance. WordPress core tables are usually well-indexed, but if you create custom tables, ensure proper indexing for frequently used columns.
- Limiting Revisions: WordPress saves post revisions by default. You can limit revisions using the WP_POST_REVISIONS constant in your wp-config.php file.
php define('WP_POST_REVISIONS', 5); // Limit to 5 revisions per post
- Using Object Caching: Utilize object caching mechanisms like Memcached or Redis to store frequently used data in memory, reducing database load.
5. Plugins for Database Management:
Several plugins can assist in managing database operations effectively:
- WP-Optimize: This plugin optimizes the database by cleaning up unnecessary data, optimizing tables, and reducing database bloat.
- Advanced Database Cleaner: It helps you clean up the database by removing spam comments, revisions, and other clutter.
- WP Migrate DB: For moving your WordPress site, this plugin ensures a smooth migration by handling database search and replace operations.
Conclusion
Managing database operations is an integral aspect of maintaining a healthy and high-performing WordPress website. Understanding the structure of the WordPress database, performing CRUD operations, utilizing the wpdb class, and implementing optimization techniques are essential skills for any WordPress developer. By mastering these techniques and best practices, you can ensure the longevity and success of your WordPress site, offering users a seamless experience while navigating your content.
Table of Contents
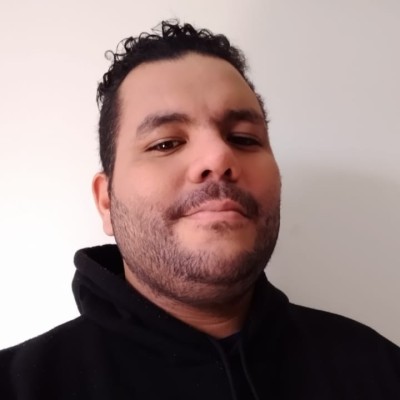
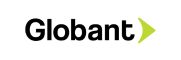