WordPress Blogging Platform: Extending Functionality with Programming
WordPress is undoubtedly one of the most popular platforms for blogging and website creation. Its user-friendly interface and vast library of themes and plugins make it accessible to beginners and professionals alike. However, if you’re looking to take your WordPress blog to the next level and unlock its full potential, it’s time to delve into the world of programming.
Table of Contents
In this comprehensive guide, we will explore various ways to extend the functionality of your WordPress blog using programming. From creating custom themes to developing plugins and automating tasks, you’ll discover how coding can transform your WordPress experience.
1. Why Extend WordPress Functionality with Programming?
Before we dive into the technical details, let’s understand why extending WordPress functionality with programming is essential.
- Tailored Solutions: Programming allows you to create custom solutions that perfectly match your blog’s unique needs. Whether it’s a specific feature, design element, or functionality, you have complete control.
- Efficiency: Automated processes save time and reduce manual work. With programming, you can streamline tasks such as content publishing, SEO optimization, and data analysis.
- Scalability: As your blog grows, so do your requirements. Programming enables you to scale your WordPress site efficiently, ensuring it can handle increased traffic and new features.
- Competitive Edge: Customization sets you apart from the crowd. A well-coded, unique blog attracts more visitors and keeps them engaged.
Now that you understand the advantages, let’s explore how to extend WordPress functionality with programming.
2. Creating Custom Themes
Your WordPress blog’s theme is its visual identity. Customizing it with programming allows you to design a unique and appealing site. Here’s how to get started:
2.1. Understand the Basics of WordPress Themes
Before diving into coding, familiarize yourself with the structure of WordPress themes. Themes consist of template files, style sheets, and functions.php, which control different aspects of your blog’s appearance and functionality.
2.2. Develop a Child Theme
To ensure that your customizations are not lost during theme updates, create a child theme. A child theme inherits the parent theme’s functionality but allows you to make modifications without altering the original theme files.
php // Example of a child theme's style.css file /* Theme Name: My Custom Theme Template: parent-theme-directory */
2.3. Customize Templates
Identify the templates you want to customize, such as single.php (for single posts) or page.php (for static pages). Copy the relevant template file from the parent theme to your child theme’s directory and start making changes.
2.4. Use WordPress Functions
WordPress provides a rich set of functions (such as the_title() and the_content()) that you can use in your templates to retrieve and display content dynamically.
php // Example: Displaying the post title <h1><?php the_title(); ?></h1>
2.5. Style with CSS
Enhance the visual aspect of your theme using Cascading Style Sheets (CSS). You can add custom CSS rules to your child theme’s style.css file.
css /* Example: Changing the font color */ body { color: #333; }
By creating a custom theme, you can give your WordPress blog a distinctive look and feel that aligns with your brand or personal style.
3. Developing Custom Plugins
Plugins are a cornerstone of WordPress functionality extension. They allow you to add new features and capabilities to your blog. Creating custom plugins with programming gives you complete control over your blog’s behavior.
3.1. Understand WordPress Plugin Structure
A WordPress plugin typically consists of a main PHP file and additional files or directories for assets and libraries. Understanding the basic structure is essential for developing your own.
3.2. Create a New Plugin
Start by creating a new directory for your plugin within the wp-content/plugins/ directory. Inside this directory, you’ll place your main PHP file.
php // Example: Plugin header /* Plugin Name: My Custom Plugin Description: Adds custom functionality to your WordPress blog. Version: 1.0 Author: Your Name */
3.3. Define Plugin Functionality
In your main PHP file, define the functionality you want to add to your blog. This might include custom post types, widgets, shortcodes, or even API integrations.
php // Example: Adding a custom shortcode function custom_shortcode() { return 'This is my custom shortcode content.'; } add_shortcode('my_shortcode', 'custom_shortcode');
3.4. Test and Debug
Testing is a crucial step in plugin development. Activate your plugin and verify that it works as intended. Debug any issues using tools like WP_Debug.
Creating custom plugins empowers you to add features that are not available through existing plugins, giving your blog a competitive edge.
4. Automating Tasks with WordPress Programming
Automation is a game-changer in blogging. It allows you to save time and ensure consistent performance. Here’s how to automate tasks with WordPress programming:
4.1. Use Cron Jobs
WordPress has a built-in Cron system that enables you to schedule tasks. By creating custom Cron jobs, you can automate tasks like publishing posts, sending emails, or performing backups.
php // Example: Scheduling a custom task function custom_task() { // Your task code here } add_action('my_custom_schedule', 'custom_task'); // Schedule the task to run daily if (!wp_next_scheduled('my_custom_schedule')) { wp_schedule_event(time(), 'daily', 'my_custom_schedule'); }
4.2. Implement Custom Scripts
Custom scripts can automate various tasks, such as data imports, content generation, or data analysis. You can create these scripts using PHP and WordPress functions.
php // Example: Importing data from an external source function import_data() { // Your import code here } import_data();
4.3. Utilize Plugins
Several plugins, such as WP-CLI, allow you to manage your WordPress blog through the command line. This is particularly useful for automating complex tasks and maintenance.
sh # Example: Running a WP-CLI command wp plugin activate my-custom-plugin
Automation reduces the risk of human error and ensures that repetitive tasks are executed consistently, freeing up your time for more creative aspects of blogging.
5. Optimizing SEO with Programming
Search Engine Optimization (SEO) is critical for driving organic traffic to your blog. While there are many SEO plugins available for WordPress, you can take customization to the next level with programming.
5.1. Custom SEO Functions
Develop custom SEO functions to control how your content appears in search engine results. You can set custom title tags, meta descriptions, and canonical URLs for each post or page.
php // Example: Custom meta description function custom_meta_description() { global $post; // Your meta description logic here $meta_description = 'Custom meta description for ' . get_the_title(); echo '<meta name="description" content="' . esc_attr($meta_description) . '">'; } add_action('wp_head', 'custom_meta_description', 5);
5.2. Structured Data Markup
Use structured data markup (Schema.org) to enhance your content’s visibility in search results. By coding structured data directly into your posts, you can provide search engines with detailed information about your content.
html <!-- Example: Adding structured data to a recipe post --> <script type="application/ld+json"> { "@context": "http://schema.org", "@type": "Recipe", "name": "Delicious Chocolate Cake", "author": { "@type": "Person", "name": "Your Name" }, "datePublished": "2023-08-30", "description": "A mouthwatering chocolate cake recipe.", "image": "link-to-image.jpg", "recipeYield": "8 servings", "recipeIngredient": [ "2 cups of flour", "1 cup of sugar", "..." ], "recipeInstructions": "..." } </script>
Customizing your blog’s SEO with programming helps you fine-tune your content for search engines, ultimately increasing your chances of ranking higher in search results.
6. Enhancing Security
Security is a top priority for any WordPress blog. Programming can help you strengthen your blog’s defenses against potential threats.
6.1. Implement Security Plugins
Utilize security plugins or write custom security scripts to protect your blog from common vulnerabilities like SQL injection and cross-site scripting (XSS) attacks.
php // Example: Protecting against SQL injection function sanitize_input($input) { global $wpdb; return $wpdb->prepare($input); }
6.2. Regularly Update Plugins and Themes
Keep your themes, plugins, and WordPress core up to date to patch known security vulnerabilities. You can automate this process or set up notifications for updates.
6.3. Monitor User Activity
Implement user activity monitoring to detect suspicious behavior. Plugins like Wordfence or custom scripts can help you track login attempts and changes to sensitive settings.
php // Example: Logging login attempts function log_login_attempt($username) { // Your logging code here } add_action('wp_login_failed', 'log_login_attempt');
By proactively addressing security concerns with programming, you can safeguard your blog and the data of your visitors.
Conclusion
Extending the functionality of your WordPress blog with programming is a powerful way to unlock its full potential. Whether you’re customizing themes, developing plugins, automating tasks, optimizing SEO, or enhancing security, coding skills open up a world of possibilities.
Remember that while programming offers immense customization and control, it also requires continuous learning and maintenance. Stay updated with the latest WordPress and programming trends to keep your blog at its best.
Start exploring the exciting realm of WordPress programming, and watch your blog thrive like never before!
Table of Contents
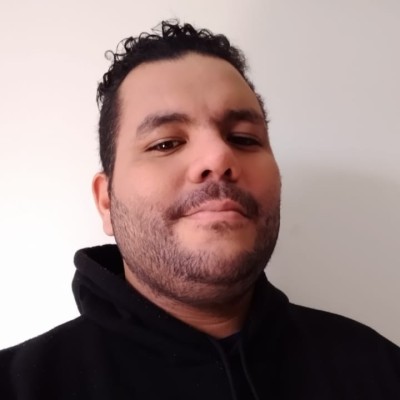
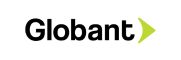