WordPress Gutenberg: Custom Block Development with Programming
WordPress Gutenberg has revolutionized the way we create content within WordPress. Its block-based editor offers a dynamic and intuitive way to design web pages. However, the true power of Gutenberg lies in its extensibility through custom block development. In this guide, we’ll explore how you can leverage programming to build your own custom blocks, adding unique functionality and interactivity to your WordPress website.
Table of Contents
1. Introduction to Custom Blocks in Gutenberg
When Gutenberg was introduced, it brought a new level of flexibility and creativity to WordPress content creation. The concept of blocks allowed users to break down their content into modular components, such as paragraphs, headings, images, and more. This block-based approach made it easier to manipulate content and layouts without needing to rely on complex HTML and CSS.
However, the built-in blocks only scratch the surface of what Gutenberg can offer. Custom blocks empower developers to create unique content elements that suit their specific needs. Whether it’s a complex pricing table, an interactive quiz, or a dynamic portfolio showcase, custom blocks open the door to endless possibilities.
2. Getting Started with Custom Block Development
2.1. Prerequisites
Before diving into custom block development, you should have a solid understanding of:
- WordPress core concepts
- HTML, CSS, and JavaScript
- WordPress theme and plugin development
2.2. Setting Up Your Environment
To get started, create a new WordPress plugin or add the custom block to your existing theme’s functions.php file. This ensures that your custom block remains separate from the core WordPress files, making updates and maintenance smoother.
In your plugin or theme, create a new folder for your custom block. Inside this folder, you’ll need to set up the necessary files and structure, including:
- index.js: This is the entry point for your custom block. It will contain your block’s JavaScript code.
- style.css: The styles for your custom block.
- editor.css: Styles specifically for the block editor.
- block.json: Block configuration and metadata.
3. Building Your First Custom Block
Let’s walk through building a simple custom block – a testimonial block that allows users to add client testimonials to their pages.
3.1. Setting Up the Block Structure
In your index.js file, start by importing the necessary components and functions:
javascript import { registerBlockType } from '@wordpress/blocks'; import { TextControl, RichText, InspectorControls } from '@wordpress/block-editor'; import { PanelBody } from '@wordpress/components';
3.2. Registering the Block
Next, use the registerBlockType function to define your custom block:
javascript registerBlockType('your-namespace/testimonial', { title: 'Testimonial', icon: 'format-quote', category: 'common', attributes: { author: { type: 'string', default: 'Anonymous' }, content: { type: 'string', default: '' } }, edit: function(props) { const { attributes, setAttributes } = props; return ( <div> <InspectorControls> <PanelBody title="Testimonial Settings"> <TextControl label="Author" value={attributes.author} onChange={(value) => setAttributes({ author: value })} /> </PanelBody> </InspectorControls> <div className={props.className}> <RichText tagName="p" placeholder="Enter testimonial content..." value={attributes.content} onChange={(value) => setAttributes({ content: value })} /> </div> </div> ); }, save: function() { // Save functionality here } });
3.3. Styling Your Block
In your style.css file, add styling to make your testimonial block visually appealing:
css /* Add styles for the block container */ .your-namespace-testimonial { background-color: #f9f9f9; padding: 20px; border: 1px solid #ccc; border-radius: 5px; } /* Style the testimonial author */ .your-namespace-testimonial .testimonial-author { font-size: 16px; font-weight: bold; } /* Style the testimonial content */ .your-namespace-testimonial .testimonial-content { margin-top: 10px; font-size: 14px; }
4. Adding Functionality to Your Custom Block
Now that your testimonial block is set up, let’s add some interactivity. We’ll make it possible for users to toggle the visibility of the author’s name.
4.1. Modifying the Edit Function
Update the edit function in your index.js file to include a checkbox to toggle the author’s visibility:
javascript // ... (previous code) edit: function(props) { const { attributes, setAttributes } = props; return ( <div> <InspectorControls> {/* ... (previous code) */} </InspectorControls> <div className={props.className}> <RichText tagName="p" placeholder="Enter testimonial content..." value={attributes.content} onChange={(value) => setAttributes({ content: value })} /> <label> <input type="checkbox" checked={attributes.showAuthor} onChange={() => setAttributes({ showAuthor: !attributes.showAuthor })} /> Show Author </label> {attributes.showAuthor && ( <div className="testimonial-author"> <RichText tagName="p" placeholder="Enter author name..." value={attributes.author} onChange={(value) => setAttributes({ author: value })} /> </div> )} </div> </div> ); }, // ... (remaining code)
4.2. Update the Block Styles
To enhance the visual experience, modify your style.css file to handle the new changes:
css /* ... (previous styles) */ /* Style the toggle label */ .your-namespace-testimonial .toggle-label { font-size: 12px; margin-left: 5px; } /* Hide the author by default */ .your-namespace-testimonial .testimonial-author { display: none; }
Conclusion
Custom block development in WordPress Gutenberg opens up a world of possibilities for enhancing your website’s functionality and design. With just a little bit of programming, you can create unique content elements that cater to your specific needs. This guide provided a step-by-step introduction to building your own custom block, from setting up the development environment to adding interactivity.
As you continue your custom block development journey, remember to explore the vast library of Gutenberg components and features to create even more advanced blocks. Whether you’re a developer looking to extend your website’s capabilities or a business owner aiming to create a distinct online presence, Gutenberg’s custom block development has you covered. So go ahead, unleash your creativity and start crafting stunning, interactive content blocks that will captivate your audience like never before.
Table of Contents
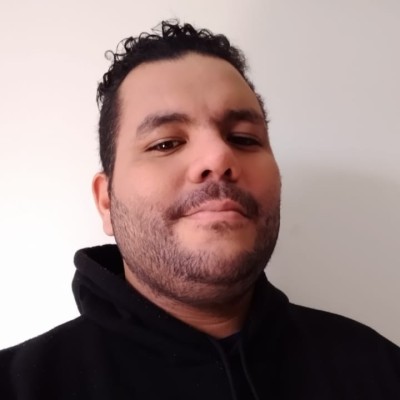
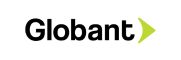