WordPress Headless CMS: Decoupling Frontend and Backend with Programming
In the world of web development, the decoupling of frontend and backend systems has become a transformative trend, enhancing flexibility, scalability, and overall performance. One technology that has gained significant attention in this regard is the WordPress Headless CMS. In this article, we’ll delve into what a Headless CMS is, why it matters, and how you can harness its power to decouple the frontend and backend of your WordPress site through programming.
Table of Contents
1. Understanding the Headless CMS Architecture
Traditional content management systems, like WordPress, combine both the frontend and backend into a monolithic structure. This means that the presentation layer (frontend) and the content management logic (backend) are tightly integrated. While this approach simplifies initial setup, it can hinder flexibility and scalability as the project grows.
A Headless CMS takes a different approach. It separates the content management system from the presentation layer. In this architecture, the backend is responsible solely for content creation, storage, and management. The frontend, on the other hand, becomes an independent entity that can be developed using different technologies.
2. Advantages of Going Headless
Decoupling the frontend and backend offers several key benefits:
- Flexibility: With a Headless CMS, you’re not bound by the limitations of a monolithic system. You can choose the best tools and technologies for both the frontend and backend, enabling you to create a more tailored and efficient solution.
- Scalability: As your website or application grows, you can scale the frontend and backend separately. This allows for optimized resource allocation and improved performance.
- Improved Performance: Since the frontend is disconnected from the backend, there’s less server load, resulting in faster loading times and better overall user experiences.
- Enhanced Security: Separating the frontend and backend mitigates security risks. Vulnerabilities in the frontend won’t directly expose sensitive backend data.
- Future-Proofing: Technology evolves rapidly. A Headless CMS future-proofs your project by enabling you to adapt to new tools and trends without overhauling the entire system.
3. Implementing WordPress as a Headless CMS
WordPress, initially known for its blogging capabilities, has evolved into a versatile content management system. Its plugin ecosystem, themes, and user-friendly interface make it a popular choice. However, to utilize it as a Headless CMS, a shift in approach is required.
Step 1: Set Up the Backend
Begin by setting up your WordPress backend as usual. Install the necessary plugins for content management and create your desired content structure. However, keep in mind that the frontend won’t be using WordPress’s default theme rendering.
Step 2: Develop the Frontend
This is where the decoupling happens. You’ll develop the frontend independently using your preferred programming language or framework. Common choices include React, Angular, or Vue.js.
Below is a simplified example of how you might fetch and display WordPress content using React:
jsx import React, { useState, useEffect } from 'react'; function BlogPost() { const [post, setPost] = useState({}); useEffect(() => { async function fetchPost() { const response = await fetch('your-wordpress-api-endpoint'); const data = await response.json(); setPost(data); } fetchPost(); }, []); return ( <div> <h2>{post.title}</h2> <div dangerouslySetInnerHTML={{ __html: post.content }} /> </div> ); } export default BlogPost;
In this example, the fetchPost function retrieves content from the WordPress API, and the fetched data is then used to populate the React component.
Step 3: Connect with the WordPress API
WordPress provides a robust REST API that allows you to retrieve content and interact with your site’s backend. You’ll need to understand the API’s endpoints and authentication mechanisms to fetch and display the data on your frontend.
4. Overcoming Challenges
While the benefits of a Headless CMS are significant, there are challenges to overcome:
- Steeper Learning Curve: Developing the frontend and backend separately requires a deeper understanding of both areas, which might pose a challenge for some developers.
- Complexity: The decoupled architecture adds complexity to the project. Managing multiple technologies and ensuring smooth communication between frontend and backend components can be intricate.
- SEO Considerations: Traditional WordPress themes often include SEO functionalities. In a Headless setup, you’ll need to implement these functionalities manually on the frontend.
Conclusion
WordPress Headless CMS offers a powerful solution for decoupling the frontend and backend of your website or application. By leveraging this approach, you gain the flexibility to choose the best technologies for each layer, enhancing performance, scalability, and security. Despite the challenges, the benefits of a Headless CMS are driving a paradigm shift in web development that empowers developers to create more dynamic and future-proof digital experiences. So, why not take the plunge and explore the possibilities of WordPress as a Headless CMS?
Decoupling frontend and backend has never been more accessible, and with the right approach, you can revolutionize how you build digital experiences. Embrace the change, and unlock a new realm of possibilities in the world of web development.
Table of Contents
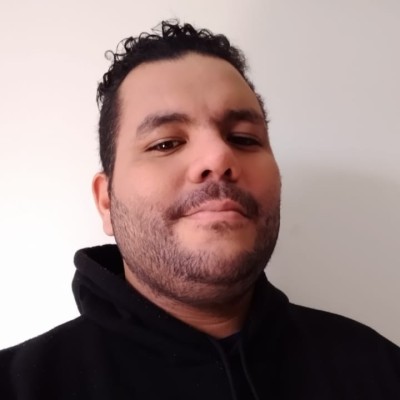
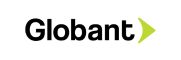