Harnessing the Power of WordPress Hooks and Actions
If you’re a WordPress developer or enthusiast, you’re probably familiar with the vast ecosystem of themes and plugins that can enhance and customize your website. But have you ever wondered how all these features and functionalities are seamlessly integrated into WordPress? The answer lies in the magic of hooks and actions.
1. What are Hooks and Actions?
Hooks and actions are essential components of the WordPress core that allow developers to modify, extend, or customize the platform’s behavior without modifying the core files. These mechanisms provide a structured and reliable way to add, remove, or modify code at specific execution points throughout the WordPress runtime.
Hooks come in two flavors: actions and filters. Actions are points where specific functions or code can be executed. Filters, on the other hand, allow developers to modify data or content before it is displayed. By using hooks, developers can seamlessly integrate their code into WordPress, ensuring compatibility with other themes and plugins.
2. The Power of Actions
Actions are the building blocks that enable developers to trigger custom code at predefined moments during the WordPress lifecycle. When a specific event occurs, an action is fired, and any associated functions or code snippets are executed. This enables developers to inject their custom code into various parts of WordPress without modifying the core files.
To hook into an action, you use the add_action function. The basic syntax is as follows:
php add_action( $action_name, $callback_function, $priority, $accepted_args );
- $action_name: The name of the action you want to hook into.
- $callback_function: The function or method to be executed when the action occurs.
- $priority: Optional. Defines the order in which the functions are executed when multiple actions are hooked into the same action name.
- $accepted_args: Optional. The number of arguments the callback function can accept.
Example:
Let’s say you want to display a welcome message on your website’s homepage. You can hook into the wp_head action, which is fired in the <head></head> section of the page.
php function display_welcome_message() { echo '<p>Welcome to our website!</p>'; } add_action( 'wp_head', 'display_welcome_message' );
In this example, the display_welcome_message function will be called whenever the wp_head action is triggered, and the welcome message will be displayed at the top of the website.
3. Leveraging Filters
Filters, as mentioned earlier, allow you to modify data before it is rendered or saved in the database. They are similar to actions but focus on manipulating values rather than triggering functions. Filters accept a variable, modify it, and then return the updated version.
To hook into a filter, you use the add_filter function. The basic syntax is as follows:
php add_filter( $filter_name, $callback_function, $priority, $accepted_args );
- $filter_name: The name of the filter you want to hook into.
- $callback_function: The function or method responsible for modifying the data.
- $priority: Optional. Specifies the order in which the filters are executed when multiple filters are hooked into the same filter name.
- $accepted_args: Optional. The number of arguments the callback function can accept.
Example:
Let’s consider a scenario where you want to add a custom prefix to all post titles displayed on your website. You can achieve this by hooking into the the_title filter.
php function add_custom_prefix_to_titles( $title ) { return 'Custom Prefix: ' . $title; } add_filter( 'the_title', 'add_custom_prefix_to_titles' );
Now, every time the the_title filter is applied (e.g., when displaying post titles), the add_custom_prefix_to_titles function will modify the title and prepend it with “Custom Prefix: “.
4. Using Hooks from Themes and Plugins
One of the most powerful aspects of hooks and actions is their ability to enable seamless integration of custom functionality from themes and plugins. Developers can use hooks to allow users to customize their products without modifying the core theme or plugin files.
For theme developers, it’s common to provide action hooks at strategic points in the template files. This way, users can add custom content or functionality without altering the original theme files. Similarly, filters can be used to modify theme elements such as titles, excerpts, and more.
Plugin developers can also harness the power of hooks to let users customize their plugin’s behavior. By offering well-documented hooks, plugin authors empower users to tailor the plugin to their specific needs while still being able to receive updates and maintain compatibility.
5. Do’s and Don’ts with Hooks and Actions
Using hooks and actions can significantly enhance your WordPress development experience, but it’s essential to understand some best practices to avoid potential pitfalls.
Do:
Check for Function Existence: Before adding an action or filter, verify that the function or method you are hooking into exists. You can use the function_exists function to prevent errors when removing or modifying hooks.
php if ( function_exists( 'display_welcome_message' ) ) { remove_action( 'wp_head', 'display_welcome_message' ); }
- Use Proper Priority: When adding actions or filters, consider the priority parameter. Other plugins or themes might have hooked into the same action or filter, and the order can be crucial for desired functionality.
- Document Your Hooks: If you’re developing themes or plugins, make sure to document the available hooks and their usage. Clear documentation encourages users to customize your products.
Don’t:
- Modify Core Files: Avoid directly modifying the core WordPress files to add custom functionality. Not only is this bad practice, but it can also lead to issues during updates.
- Overuse Hooks: While hooks are powerful, excessive use can make code difficult to manage and maintain. Use hooks strategically and consider the best approach for your specific use case.
6. Advanced Hook Techniques
Conditional Hooks
Conditional hooks allow you to execute actions or filters based on specific conditions. For example, you may want to enqueue a script or style only on certain pages or posts. WordPress provides conditional functions that you can use within your hooks to achieve this.
Example:
php function enqueue_custom_script() { if ( is_single() ) { wp_enqueue_script( 'custom-script', 'path/to/custom.js', array(), '1.0', true ); } } add_action( 'wp_enqueue_scripts', 'enqueue_custom_script' );
In this example, the enqueue_custom_script function will be called only on single post pages due to the is_single() conditional check.
Removing Hooks
Sometimes, you may need to remove an existing action or filter that’s added by another theme or plugin. The remove_action and remove_filter functions can be used to detach hooks.
Example:
php // Let's assume a theme or plugin added a filter like this: add_filter( 'the_content', 'some_function_to_modify_content' ); // To remove the filter: remove_filter( 'the_content', 'some_function_to_modify_content' );
Anonymous Functions
In some cases, you might want to use anonymous functions or closures for hooks. Anonymous functions are handy when you need to define a one-time callback without creating a separate function.
Example:
php add_action( 'wp_footer', function() { echo '<p>This is a custom footer text.</p>'; });
Conclusion
WordPress hooks and actions provide an elegant and powerful way to extend and customize WordPress without directly modifying core files. By leveraging hooks, developers can create themes and plugins that are flexible and user-friendly, while users can tailor their websites to meet their exact requirements.
Whether you’re a theme or plugin developer or just someone looking to customize their WordPress website, understanding hooks and actions will empower you to take full control of your WordPress journey.
Remember, hooks are not just a feature; they are a philosophy that encourages the WordPress community to collaborate and build upon each other’s work, making WordPress the incredible platform it is today.
Table of Contents
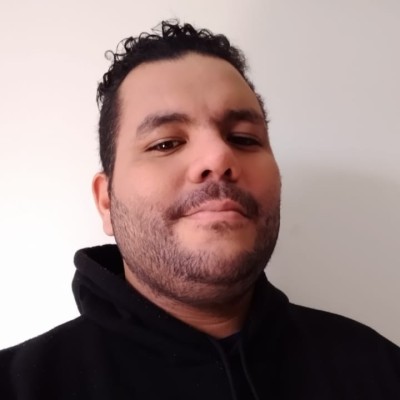
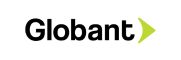