Implementing User Registration and Login in WordPress with Programming
WordPress is a versatile platform used for everything from blogs and e-commerce websites to corporate portals and more. One essential feature for many websites is user registration and login functionality. Whether you want to create a membership site or just give your visitors a more personalized experience, implementing user registration and login can be crucial. In this guide, we’ll walk you through the process of adding user registration and login functionality to your WordPress website using programming.
Table of Contents
1. Why Implement User Registration and Login?
Before we dive into the technical details, let’s understand why adding user registration and login is beneficial for your WordPress site:
1.1. Personalized User Experience
User registration allows you to collect user information, helping you provide a personalized experience.
Registered users can have profiles, avatars, and even customize their preferences.
1.2. Content Restriction
You can restrict certain content, making it accessible only to registered users.
Monetize your content by offering premium access to registered members.
1.3. Community Building
Enable discussions and community interaction through user-generated content.
Encourage user engagement by allowing comments and contributions.
1.4. Data Collection
Collect valuable user data for marketing purposes.
Use data analytics to improve your website’s performance.
Now that you understand the importance of user registration and login, let’s get into the technical aspects.
2. Prerequisites
Before we start coding, ensure you have the following prerequisites:
- WordPress Website: You should have a WordPress website up and running.
- Administrator Access: Ensure you have administrator access to your WordPress dashboard.
- Basic Knowledge of PHP: Familiarity with PHP will be helpful, as we’ll be writing PHP code to extend WordPress’s functionality.
3. Creating a User Registration Form
The first step in implementing user registration is creating a registration form. We’ll do this by creating a custom WordPress plugin. Here’s how:
Step 1: Create a New Directory for Your Plugin
In your WordPress installation directory, navigate to wp-content/plugins. Create a new directory for your plugin, e.g., custom-registration.
Step 2: Create the Main Plugin File
Inside your plugin directory, create a PHP file for your plugin, e.g., custom-registration.php. This file will contain the plugin’s main code.
Step 3: Define the Plugin Header
In custom-registration.php, start by defining the plugin header:
php <?php /* Plugin Name: Custom Registration Description: Adds custom registration functionality to your WordPress site. Version: 1.0 Author: Your Name */ // Plugin code goes here
Replace the placeholders with your plugin’s details.
Step 4: Create the Registration Form
Now, let’s create a simple user registration form. We’ll use WordPress hooks and functions to display the form on a page.
php // Function to display the registration form function custom_registration_form() { ob_start(); ?> <form id="registration-form" action="<?php echo $_SERVER['REQUEST_URI']; ?>" method="post"> <label for="username">Username:</label> <input type="text" name="username" id="username" required /><br /> <label for="email">Email:</label> <input type="email" name="email" id="email" required /><br /> <label for="password">Password:</label> <input type="password" name="password" id="password" required /><br /> <input type="submit" name="submit" value="Register" /> </form> <?php return ob_get_clean(); } // Function to handle form submissions function custom_registration() { if (isset($_POST['submit'])) { // Handle form submission and user creation here } } // Add the registration form to a WordPress page function add_registration_form() { add_shortcode('registration_form', 'custom_registration_form'); } add_action('init', 'add_registration_form');
In the above code:
- custom_registration_form() displays the registration form.
- custom_registration() will handle form submissions (we’ll implement this shortly).
- add_registration_form() adds a shortcode [registration_form] that you can use to display the form on any WordPress page.
Step 5: Handle Form Submissions
Now, let’s complete the custom_registration() function to handle form submissions and user registration. We’ll use the wp_insert_user() function to create a new user.
php // Function to handle form submissions function custom_registration() { if (isset($_POST['submit'])) { $username = sanitize_user($_POST['username']); $email = sanitize_email($_POST['email']); $password = $_POST['password']; // Check if the username already exists if (username_exists($username)) { // Username already in use echo "Username already exists. Please choose another."; return; } // Create a new user $user_id = wp_create_user($username, $password, $email); if (is_wp_error($user_id)) { // Error creating user echo "Error creating user. Please try again."; } else { // Registration successful echo "Registration successful. <a href='" . wp_login_url() . "'>Login here</a>."; } } }
In this code:
- We sanitize and validate the user input.
- Check if the username already exists.
- Create a new user using wp_create_user().
- Handle any errors that may occur during user creation.
Step 6: Adding the Registration Form to a Page
To display the registration form on a page, create a new page or edit an existing one. Add the [registration_form] shortcode to the page content. Users can now visit this page to register on your website.
Step 7: Test the Registration Form
Test the registration form to ensure everything is working as expected. Register a test user and make sure they can log in after registration.
4. Implementing User Login
Now that we’ve implemented user registration, let’s move on to user login functionality.
Step 1: Create a Login Form
Similar to the registration form, we’ll create a login form. Add this code to your custom-registration.php file:
php // Function to display the login form function custom_login_form() { if (!is_user_logged_in()) { ob_start(); ?> <form id="login-form" action="<?php echo wp_login_url(); ?>" method="post"> <label for="username">Username:</label> <input type="text" name="username" id="username" required /><br /> <label for="password">Password:</label> <input type="password" name="password" id="password" required /><br /> <input type="submit" name="submit" value="Log In" /> </form> <?php return ob_get_clean(); } else { // User is already logged in, display a logout link return '<a href="' . wp_logout_url() . '">Logout</a>'; } } // Add the login form to a WordPress page function add_login_form() { add_shortcode('login_form', 'custom_login_form'); } add_action('init', 'add_login_form');
In this code:
- custom_login_form() displays the login form if the user is not logged in.
- If the user is already logged in, it displays a logout link.
Step 2: Add the Login Form to a Page
Just like the registration form, create a new page or edit an existing one. Add the [login_form] shortcode to the page content. Users can now visit this page to log in.
Step 3: Test User Login
Test the login form to ensure users can log in successfully. Make sure to test with both registered and non-registered user accounts.
5. Customizing the User Experience
You’ve now successfully implemented user registration and login functionality in WordPress. However, you can take it a step further by customizing the user experience. Here are a few ways to do that:
5.1. Redirect Users After Login
You can redirect users to a specific page after they log in. To do this, add the following code to your functions.php file in your theme directory:
php function custom_login_redirect($redirect_to, $request, $user) { // Change 'your-page-slug' to the slug of the page you want to redirect to return home_url('/your-page-slug/'); } add_filter('login_redirect', 'custom_login_redirect', 10, 3);
5.2. Customize the User Profile
You can add custom fields to the user profile to collect more information from users. Use plugins like Advanced Custom Fields (ACF) to create custom user fields.
5.3. Implement User Roles
WordPress has built-in user roles like Administrator, Editor, and Subscriber. You can create custom user roles with specific permissions using plugins like Members.
5.4. Implement Two-Factor Authentication (2FA)
Enhance security by adding two-factor authentication to your login process using plugins like Google Authenticator – Two-Factor Authentication.
Conclusion
Implementing user registration and login functionality in WordPress can significantly enhance the user experience and open up new possibilities for your website. By following the steps outlined in this guide, you can create a custom registration and login system tailored to your specific needs. Don’t forget to keep security in mind and regularly update your plugins and WordPress installation to protect user data. With these features in place, you’ll be well on your way to building a dynamic and engaging WordPress website.
Table of Contents
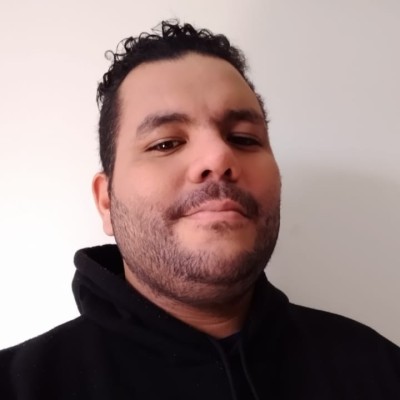
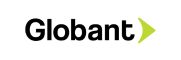