WordPress Multilingual Sites: Language Redirect with Programming
Multilingual websites have become essential in our globalized world. They cater to diverse audiences who speak different languages. WordPress is one of the most popular platforms for building websites, and luckily, it has robust support for creating multilingual sites. However, ensuring that users land on the right language version of your site can be challenging. In this blog, we’ll explore how to implement language redirection on WordPress multilingual sites using programming. We’ll cover various methods and provide code samples to help you enhance user experience and reach a wider audience.
Table of Contents
1. Understanding the Importance of Language Redirection
When visitors land on your website, it’s crucial to provide them with content in their preferred language. If they have to search for the right language version, it can lead to frustration and potentially deter them from exploring your site further. Language redirection ensures that users are directed to the appropriate language version of your site based on their browser settings, location, or language preference. This enhances user experience and can significantly impact your site’s effectiveness.
2. Choosing the Right Multilingual Plugin
Before we dive into the programming aspect of language redirection, you need to choose a reliable multilingual plugin for your WordPress site. There are several excellent options available, but two of the most popular ones are WPML (WordPress Multilingual Plugin) and Polylang. For the purpose of this blog, we’ll use WPML as our multilingual plugin.
Step 1: Install and Activate WPML
- Go to your WordPress dashboard.
- Navigate to the Plugins section.
- Click Add New and search for “WPML.”
- Install and activate the plugin.
3. Setting Up Languages in WPML
Once you have WPML installed and activated, you’ll need to set up the languages you want to support on your site. This is a fundamental step in creating a multilingual website.
Step 2: Configure Languages
In your WordPress dashboard, go to WPML > Languages.
Add the languages you want to support on your website.
Set the default language.
4. Programming Language Redirection
Now that you have WPML set up with your desired languages, it’s time to implement language redirection programmatically. We’ll cover several methods, each catering to different scenarios.
Method 1: Browser Language Redirection
One of the most user-friendly ways to redirect visitors to the right language version of your site is by detecting their browser language settings. Here’s how you can achieve this with programming.
Step 3: Add Code to Your Theme’s functions.php
php function redirect_based_on_browser_language() { $user_language = substr($_SERVER['HTTP_ACCEPT_LANGUAGE'], 0, 2); $supported_languages = array('en', 'es', 'fr'); // Add your supported languages here if (in_array($user_language, $supported_languages)) { $language_code = $user_language; } else { $language_code = 'en'; // Default to English if no match is found } $redirect_url = home_url('/' . $language_code . '/'); wp_redirect($redirect_url); exit; } add_action('template_redirect', 'redirect_based_on_browser_language');
This code snippet checks the user’s browser language and redirects them to the appropriate language version of your site based on the supported languages you define.
Step 4: Test the Redirection
Open your website in different browsers with different language settings to ensure the redirection works correctly.
Method 2: Geolocation-Based Redirection
Another effective way to determine a user’s preferred language is by their geographical location. This method is particularly useful if your target audience spans multiple countries where the same language may have regional variations.
Step 5: Install and Configure the GeoIP Detection Plugin
To implement geolocation-based redirection, you’ll need a GeoIP detection plugin. MaxMind’s GeoIP2 is a popular choice. Install and configure it on your WordPress site.
Step 6: Add Code for Geolocation-Based Redirection
php function redirect_based_on_geolocation() { $user_location = geoip_detect2_get_info_from_current_ip(); if ($user_location && isset($user_location->country->isoCode)) { $country_code = strtolower($user_location->country->isoCode); $supported_countries = array('us', 'ca', 'fr'); // Add ISO country codes for supported countries if (in_array($country_code, $supported_countries)) { $language_code = ($country_code === 'ca') ? 'fr' : 'en'; // Define language codes for each country } else { $language_code = 'en'; // Default to English for unsupported countries } $redirect_url = home_url('/' . $language_code . '/'); wp_redirect($redirect_url); exit; } } add_action('template_redirect', 'redirect_based_on_geolocation');
This code snippet uses the GeoIP information to redirect users based on their country of origin.
Step 7: Test the Geolocation-Based Redirection
Test the redirection by accessing your site from different locations or using a VPN to simulate different locations.
Method 3: Language Selector with Cookie Storage
While automatic redirection is convenient, some users may prefer to choose their language manually. Implementing a language selector with cookie storage is a user-centric approach.
Step 8: Create a Language Selector
In your WordPress theme, create a language selector in your header or footer. Here’s an example HTML structure:
html <div class="language-selector"> <a href="#" data-lang="en">English</a> <a href="#" data-lang="es">Español</a> <a href="#" data-lang="fr">Français</a> </div>
Step 9: Add JavaScript for Cookie Storage
javascript jQuery(document).ready(function($) { $('.language-selector a').click(function(e) { e.preventDefault(); var selectedLang = $(this).data('lang'); setLanguageCookie(selectedLang); window.location.reload(); }); function setLanguageCookie(lang) { var expiryDate = new Date(); expiryDate.setFullYear(expiryDate.getFullYear() + 1); // Cookie expiration in 1 year document.cookie = 'site_language=' + lang + '; expires=' + expiryDate.toUTCString() + '; path=/'; } });
This JavaScript code stores the selected language in a cookie and reloads the page with the chosen language.
Step 10: Implement Cookie-Based Redirection
Now, modify your previous redirection code to consider the language cookie.
php function redirect_based_on_cookie() { if (isset($_COOKIE['site_language'])) { $language_code = $_COOKIE['site_language']; } else { $language_code = 'en'; // Default to English if no language cookie is found } $redirect_url = home_url('/' . $language_code . '/'); wp_redirect($redirect_url); exit; } add_action('template_redirect', 'redirect_based_on_cookie');
This code checks for the language cookie and redirects users accordingly.
Step 11: Test the Cookie-Based Redirection
Test the language selector and cookie-based redirection by selecting different languages and checking if the site loads in the chosen language upon refresh.
Conclusion
Implementing language redirection on your WordPress multilingual site is a crucial step to enhance user experience and reach a wider audience. In this blog, we’ve explored three different methods for language redirection: browser language detection, geolocation-based redirection, and a language selector with cookie storage. Each method caters to different user preferences and scenarios.
By following the steps and using the provided code samples, you can ensure that visitors to your site are directed to the appropriate language version, making their experience seamless and enjoyable. This, in turn, can help you expand your global reach and effectively communicate with a diverse audience. So, go ahead and implement language redirection on your WordPress multilingual site to unlock its full potential. Your users will thank you for it.
Table of Contents
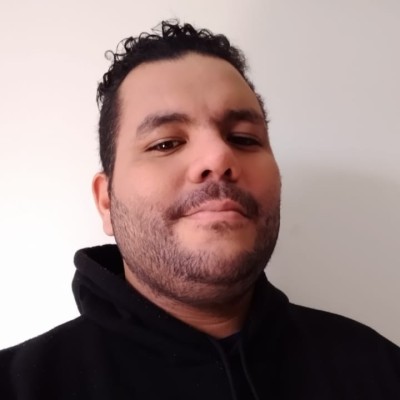
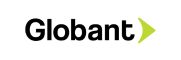