WordPress Multisite: Managing User Permissions with Programming
WordPress Multisite is a powerful feature that allows you to manage multiple WordPress websites from a single dashboard. It’s a fantastic solution for organizations, agencies, or individuals who need to run multiple websites. However, with great power comes the need for precise control over user permissions. In this blog post, we’ll explore how you can manage user permissions in WordPress Multisite using programming, providing you with the flexibility and control you need to ensure your network operates smoothly.
Table of Contents
1. Understanding WordPress Multisite User Roles
Before diving into the programming aspect, it’s crucial to understand the default user roles in WordPress Multisite. Here are the primary roles:
- Super Admin: The top-level administrator with control over the entire network, including site creation and user management.
- Administrator: Administrators have control over a specific site within the network, but they can’t manage the network itself.
- Editor: Editors can publish and manage posts and pages, but they can’t change the site’s settings.
- Author: Authors can write and publish their own posts but can’t manage other users or settings.
- Contributor: Contributors can write and edit their own posts but can’t publish them.
- Subscriber: Subscribers can only view content and manage their profiles.
These default roles provide a good starting point, but often, you’ll need more customized roles and permissions to meet your network’s specific requirements. This is where programming comes in handy.
2. Customizing User Roles and Permissions
To customize user roles and permissions in WordPress Multisite, you can use various techniques, including code snippets, plugins, and custom themes. Here, we’ll focus on using code snippets to add, modify, or remove user roles and define their capabilities.
2.1. Adding a Custom User Role
Let’s say you want to add a new user role called “Content Manager” with some specific capabilities. You can achieve this by adding the following code to your theme’s functions.php file or in a custom plugin:
php function add_content_manager_role() { // Add a new user role add_role( 'content_manager', 'Content Manager', array( 'read' => true, 'edit_posts' => true, 'publish_posts' => true, 'delete_posts' => true, // Add more capabilities as needed ) ); } // Hook the function to the 'init' action add_action('init', 'add_content_manager_role');
In this code, we use the add_role function to create a new role named “Content Manager” with specific capabilities like reading, editing, publishing, and deleting posts. You can customize these capabilities to match your requirements.
2.2. Modifying Existing User Roles
If you want to modify an existing user role’s capabilities, you can use the add_cap and remove_cap functions. For example, let’s say you want to allow Editors to manage comments:
php function modify_editor_role() { $editor_role = get_role('editor'); // Add the capability to manage comments $editor_role->add_cap('moderate_comments'); } // Hook the function to the 'init' action add_action('init', 'modify_editor_role');
In this code, we retrieve the Editor role using get_role and then use add_cap to grant the “moderate_comments” capability to Editors.
2.3. Removing a User Role
To remove a user role, you can use the remove_role function. For example, if you want to remove the “Contributor” role:
php function remove_contributor_role() { remove_role('contributor'); } // Hook the function to the 'init' action add_action('init', 'remove_contributor_role');
Be cautious when removing roles, as this action is irreversible, and any users with the removed role will lose their associated capabilities.
3. Assigning Custom Roles to Users
Once you’ve created or modified user roles, you’ll need to assign them to specific users. You can do this programmatically as well.
3.1. Assigning Roles When Creating Users
When creating a new user, you can specify their role by using the wp_insert_user function. Here’s an example:
php function create_new_content_manager() { $user_data = array( 'user_login' => 'new_content_manager', 'user_pass' => 'password123', 'user_email' => 'newcontentmanager@example.com', ); $user_id = wp_insert_user($user_data); // Assign the 'content_manager' role $user = new WP_User($user_id); $user->add_role('content_manager'); } // Hook the function to the 'init' action add_action('init', 'create_new_content_manager');
In this code, we create a new user and assign them the “Content Manager” role right after creation.
3.2. Changing User Roles
You can also change a user’s role at any time. For example, if you want to promote an Author to an Editor:
php function promote_author_to_editor($user_id) { $user = new WP_User($user_id); $user->remove_role('author'); $user->add_role('editor'); } // Hook the function to an appropriate action or event add_action('some_custom_event', 'promote_author_to_editor');
In this code, we remove the “Author” role and add the “Editor” role to the specified user.
4. Advanced User Permissions
Beyond creating and modifying user roles, you can implement more advanced user permission systems using custom code. Here are a few scenarios where this might be necessary:
4.1. Restricting Access to Specific Sites
In a WordPress Multisite network, you might want to restrict certain users to specific sites within the network. You can achieve this by checking the user’s role and site context when they try to access a page. Here’s an example:
php function restrict_site_access() { if (is_user_logged_in()) { $current_user = wp_get_current_user(); // Check if the user is a Content Manager and on Site ID 2 if ($current_user->has_cap('content_manager') && get_current_blog_id() == 2) { // Allow access return; } // Redirect to a specific page or show an error message wp_redirect(home_url('/access-denied/')); exit; } } // Hook the function to the 'template_redirect' action add_action('template_redirect', 'restrict_site_access');
In this example, we check if the user is logged in, has the “Content Manager” role, and is trying to access Site ID 2. If these conditions are met, we allow access; otherwise, we redirect them to an “Access Denied” page.
4.2. Custom Capabilities for Specific Plugins or Themes
Sometimes, you may need to define custom capabilities for specific plugins or themes. For instance, if you have a custom plugin that allows users to manage events, you can create a custom capability like “manage_events” and assign it to your custom user roles. Here’s how you can do it:
php function add_custom_capabilities() { // Define a custom capability $capability = 'manage_events'; // Get all custom user roles that should have this capability $custom_roles = array('content_manager', 'event_manager'); foreach ($custom_roles as $role) { $role_obj = get_role($role); if ($role_obj) { $role_obj->add_cap($capability); } } } // Hook the function to an appropriate action or event add_action('some_custom_event', 'add_custom_capabilities');
In this code, we define a custom capability called “manage_events” and assign it to the “Content Manager” and “Event Manager” roles.
Conclusion
Managing user permissions in WordPress Multisite through programming provides you with the flexibility to tailor your network to your exact needs. Whether you need to create custom user roles, modify existing ones, or implement advanced permission systems, coding allows you to have precise control over your WordPress Multisite installation.
Remember that while coding offers immense power and customization, it also comes with responsibility. Always back up your site before making significant changes, and thoroughly test your code to ensure it works as expected.
With the knowledge shared in this blog post, you’re well-equipped to start managing user permissions in WordPress Multisite like a pro. So go ahead, customize those roles, grant the right permissions, and build a network that perfectly suits your requirements. Happy coding!
Table of Contents
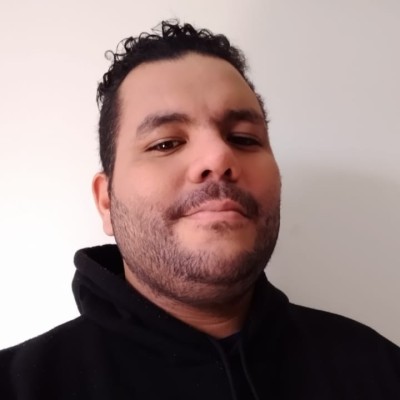
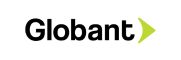