WordPress Multilingual Sites: Language Switching with Programming
In today’s globalized world, reaching a diverse audience is crucial for the success of any website. WordPress, being one of the most popular content management systems, offers powerful tools for creating multilingual websites. These websites cater to users who speak different languages, providing them with content that resonates in their native tongue. To truly enhance the user experience on multilingual sites, seamless language switching is paramount. In this article, we’ll delve into the world of programming techniques that enable a smooth language switching experience on WordPress multilingual sites.
Table of Contents
1. Understanding the Importance of Language Switching
Imagine stumbling upon a website that presents its content only in a language you don’t understand. Frustrating, right? This is where multilingual sites come to the rescue. They allow users to access content in their preferred language, making them more likely to stay, engage, and convert. However, it’s not just about offering content in different languages; it’s also about facilitating a seamless transition between these languages. This is where a well-implemented language switching feature comes into play.
2. Setting Up a Multilingual WordPress Site
Before we dive into the programming aspects of language switching, let’s quickly cover the basics of setting up a multilingual WordPress site. There are several plugins available, such as WPML (WordPress Multilingual Plugin) and Polylang, that assist in creating multilingual websites. These plugins allow you to translate posts, pages, custom post types, and even theme elements.
- Choose a Multilingual Plugin: Install and activate a multilingual plugin of your choice. For this article, we’ll use WPML.
- Configure Languages: Configure the languages you want to support on your website. Each language will have a unique identifier, such as ‘en’ for English, ‘es’ for Spanish, and so on.
- Translate Content: Translate your posts, pages, and other content elements into the desired languages. The plugin provides an interface for translating content efficiently.
3. Implementing Language Switching Programmatically
Now, let’s take our WordPress multilingual site to the next level by implementing a language switching feature using programming techniques. This involves adding a language switcher to your site’s front end, allowing users to easily toggle between languages.
3.1. Displaying Language Switcher on the Front End
To achieve this, you’ll need to modify your theme’s template files. Locate the file where you want to display the language switcher. Typically, this would be the header, footer, or a dedicated widget area. Add the following code to display a basic language switcher:
php <div class="language-switcher"> <?php $languages = apply_filters('wpml_active_languages', NULL, 'orderby=id&order=desc'); if (!empty($languages)) { foreach ($languages as $language) { echo '<a href="' . $language['url'] . '" class="language-link ' . $language['language_code'] . '">' . $language['native_name'] . '</a>'; } } ?> </div>
In this code, we’re utilizing the WPML function wpml_active_languages to retrieve the active languages along with their URLs and native names. This data is then used to generate language switcher links.
3.2. Styling the Language Switcher
Of course, the above code will likely need some styling to fit seamlessly into your website’s design. You can use CSS to style the language switcher according to your brand’s aesthetics.
css .language-switcher { display: flex; gap: 10px; } .language-link { text-decoration: none; color: #333; padding: 5px; border: 1px solid #333; border-radius: 4px; } .language-link:hover { background-color: #333; color: #fff; }
3.3. URL Structure and Language Switching
By default, WPML uses a query parameter to differentiate between languages in URLs. For instance, example.com/en represents the English version of a page, while example.com/es represents the Spanish version. When implementing a language switcher, you’ll need to ensure that the appropriate URLs are generated.
php $url = apply_filters('wpml_permalink', get_permalink($post_id), $language_code);
3.4. Language Switching in Menus
In addition to the language switcher, you might want to include language options within your site’s navigation menus. This can be achieved using the following steps:
- Go to the WordPress admin dashboard.
- Navigate to “Appearance” > “Menus.”
- Create a new menu or edit an existing one.
- Add custom links for each language using the appropriate language URLs.
- Arrange the menu items to match your desired structure.
- Assign the menu to the appropriate location on your site.
3.5. Language Switching with Custom JavaScript
To further enhance the user experience, you can implement custom JavaScript to dynamically update the language switcher without requiring a page reload. Here’s a simplified example:
javascript document.addEventListener('DOMContentLoaded', function () { const languageLinks = document.querySelectorAll('.language-link'); languageLinks.forEach(link => { link.addEventListener('click', function (event) { event.preventDefault(); const targetUrl = this.getAttribute('href'); fetch(targetUrl, { method: 'GET' }) .then(response => response.text()) .then(data => { const parser = new DOMParser(); const newDocument = parser.parseFromString(data, 'text/html'); const newLanguageLinks = newDocument.querySelectorAll('.language-link'); languageLinks.forEach((oldLink, index) => { oldLink.textContent = newLanguageLinks[index].textContent; oldLink.setAttribute('href', newLanguageLinks[index].getAttribute('href')); }); }) .catch(error => { console.error('Error fetching new content:', error); }); }); }); });
This JavaScript code fetches the content of the new page when a language link is clicked and updates the language switcher links without a full page reload.
Conclusion
A well-executed language switching feature significantly enhances the user experience on multilingual WordPress sites. By implementing programming techniques to create a seamless language switcher, you can cater to a broader audience and keep them engaged with your content. Whether you’re running a business website or a personal blog, embracing a multilingual approach can open up new horizons and foster a deeper connection with users around the world. With the right tools and a bit of coding, you can transform your WordPress site into a global platform that speaks the language of your audience.
Table of Contents
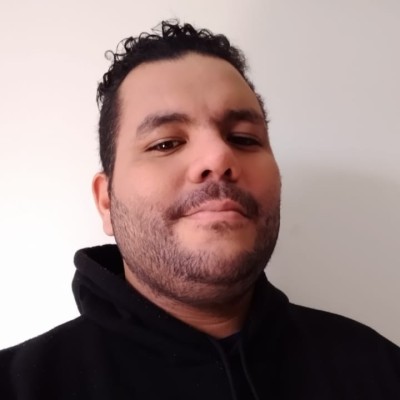
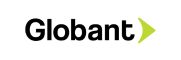