WordPress Multisite: Managing Network-wide Settings with Programming
WordPress Multisite is a powerful feature that allows you to create a network of interconnected websites using a single WordPress installation. Whether you’re managing a collection of blogs, corporate websites, or e-commerce stores, Multisite can significantly simplify your administrative tasks. However, as your network grows, managing network-wide settings and configurations can become a daunting task. In this blog post, we’ll delve into how programming can be leveraged to efficiently manage network-wide settings within a WordPress Multisite setup.
Table of Contents
1. Understanding the Power of WordPress Multisite
Before we dive into programming techniques, let’s briefly recap what WordPress Multisite is and why it’s so useful. Multisite enables you to create a network of individual websites, all sharing a single WordPress installation. Each website within the network is a separate entity, with its own content, themes, and plugins. However, they also share resources like user accounts, plugins, and themes from the main installation.
1.1. This architecture is incredibly beneficial for various scenarios:
- Efficient Management: With a single installation, you can manage multiple websites. This streamlines updates, reduces maintenance overhead, and provides centralized control.
- Cost-effectiveness: Hosting multiple websites becomes more cost-effective as they share the same resources, such as server space and bandwidth.
- Consistent Branding: Multisite is perfect for businesses or organizations that want consistent branding across various websites.
2. The Challenge of Network-wide Settings
While Multisite offers numerous advantages, efficiently managing network-wide settings becomes crucial as the network grows. Network administrators often face challenges such as:
- Consistent Configuration: Ensuring uniform settings across all sites in the network is essential to maintain a cohesive user experience.
- Time Efficiency: Manually configuring settings for each site can be time-consuming and error-prone.
- Customization: Balancing network-wide settings with individual site customization can be complex.
This is where programming comes into play. By utilizing programming techniques, you can automate processes, enforce consistent settings, and enhance the overall efficiency of managing a WordPress Multisite network.
3. Programming Techniques for Managing Network-wide Settings
3.1. Custom Functions and Hooks
WordPress Multisite provides a plethora of hooks and filters that allow you to modify default behavior. By creating custom functions and hooking them into the appropriate actions, you can easily enforce network-wide settings.
php // functions.php in your theme or a custom plugin // Disable comments network-wide function disable_comments_network_wide() { return false; } add_filter('comments_open', 'disable_comments_network_wide', 10, 2); add_filter('pings_open', 'disable_comments_network_wide', 10, 2);
In this example, the disable_comments_network_wide function hooks into the comments_open and pings_open filters, effectively disabling comments across the entire network.
3.2. Centralized Customization with Network Options
WordPress Multisite also provides a mechanism to store network-wide settings in the database using network options. These options are accessible from any site within the network, allowing for centralized customization.
php // In a network-activated plugin // Add a network-wide setting function add_network_wide_setting() { add_network_option(null, 'custom_option', 'default_value'); } add_action('network_admin_menu', 'add_network_wide_setting'); // Retrieve the network-wide setting $custom_option = get_network_option(null, 'custom_option');
This code demonstrates how to add and retrieve a network-wide setting using add_network_option and get_network_option.
3.3. Global Styles and Scripts Management
Efficiently managing styles and scripts across all sites can be challenging. Utilizing global styles and scripts management can simplify this process.
php // In your theme's functions.php or a custom plugin // Enqueue styles globally function enqueue_global_styles() { wp_enqueue_style('global-style', get_stylesheet_directory_uri() . '/global.css'); } add_action('wp_enqueue_scripts', 'enqueue_global_styles');
By enqueuing styles and scripts in this manner, you can ensure that they are loaded on all sites within the network.
3.4. Default Content and Settings for New Sites
When new sites are added to your Multisite network, ensuring consistent default content and settings is crucial. Programming can automate this process.
php // In a network-activated plugin // Set default content for new sites function set_default_content_for_new_sites($blog_id) { switch_to_blog($blog_id); // Set default posts, pages, etc. restore_current_blog(); } add_action('wpmu_new_blog', 'set_default_content_for_new_sites');
This code utilizes the wpmu_new_blog action to automatically set default content for new sites added to the network.
4. Leveraging Multisite APIs for Enhanced Control
WordPress Multisite offers powerful APIs that allow you to interact with the network and its components programmatically. Some notable APIs include:
- wp_get_sites(): Retrieve a list of sites in the network.
- switch_to_blog() and restore_current_blog(): Temporarily switch the current site within a network-wide context.
- get_blog_option() and update_blog_option(): Retrieve and update site-specific options.
- add_user_to_blog() and remove_user_from_blog(): Manage user roles across sites.
Conclusion
Managing network-wide settings in a WordPress Multisite setup can be a complex task, especially as the network expands. However, by harnessing the power of programming, you can streamline customization, enforce consistent settings, and save valuable time. Custom functions, centralized options, global styles, and automated defaults are just a few of the programming techniques that can enhance your Multisite experience.
As your Multisite network evolves, keep exploring the APIs and programming possibilities that WordPress provides. By doing so, you’ll be well-equipped to efficiently manage your network-wide settings and ensure a seamless experience for all sites within your network.
Table of Contents
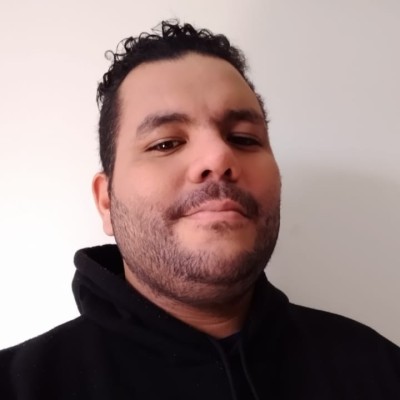
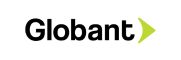