Optimizing WordPress Database with Programming Techniques
WordPress is one of the most popular content management systems (CMS) in the world, powering over 40% of all websites on the internet. Its popularity stems from its flexibility, ease of use, and a vast ecosystem of plugins and themes. However, as your WordPress site grows, its database can become a bottleneck, slowing down your website’s performance. In this blog post, we will explore various programming techniques to optimize your WordPress database and keep your site running smoothly.
Table of Contents
1. Why Optimize Your WordPress Database?
Before we dive into the programming techniques, let’s understand why optimizing your WordPress database is crucial.
- Improved Page Load Speed: A well-optimized database ensures that your web pages load quickly. Faster loading times enhance the user experience and can improve your site’s search engine rankings.
- Reduced Server Load: An efficient database requires fewer server resources to handle queries. This can lead to cost savings and improved site reliability, especially during traffic spikes.
- Enhanced Security: A tidy database is less vulnerable to SQL injection attacks and other security threats. Optimizing your database can help safeguard your site’s data.
Now, let’s explore some programming techniques to optimize your WordPress database.
2. Use a Caching Plugin
One of the most effective ways to optimize your WordPress database is by using a caching plugin. Caching plugins generate static HTML files from your dynamic WordPress pages. These static files are then served to users, reducing the need to query the database on every page load.
Example: Installing and Configuring a Caching Plugin
php // Install a caching plugin like WP Super Cache // Activate the plugin in your WordPress dashboard // Configure the plugin settings to enable caching
Popular caching plugins include WP Super Cache, W3 Total Cache, and WP Rocket. Install and configure one of these plugins to start reaping the benefits of caching.
3. Optimize Database Tables
WordPress stores a vast amount of data in its database, including posts, pages, comments, and plugin data. Over time, this can lead to database bloat, slowing down your site. You can optimize your database tables to reclaim storage space and improve performance.
Example: Optimizing Database Tables with SQL
sql -- Optimize all tables in the database OPTIMIZE TABLE wp_options, wp_posts, wp_comments;
Running the OPTIMIZE TABLE SQL command on your WordPress database tables can remove overhead and defragment the data, resulting in a more efficient database.
4. Limit Post Revisions
WordPress automatically saves revisions of your posts and pages, which can quickly accumulate and bloat your database. You can limit the number of post revisions to keep your database lean.
Example: Limiting Post Revisions in wp-config.php
php // Limit post revisions to 5 define('WP_POST_REVISIONS', 5);
Add this code to your wp-config.php file to restrict the number of post revisions. This helps control database growth and keeps your site running smoothly.
5. Clean Up Unused Plugins and Themes
Unused plugins and themes can also contribute to database bloat. Deactivate and delete any plugins or themes that you no longer use.
Example: Deleting Unused Plugins and Themes
php // Deactivate and delete a plugin // Navigate to the Plugins section in your WordPress dashboard // Locate the plugin and click "Deactivate," then "Delete"
By removing unused plugins and themes, you reduce the amount of data stored in your database, which can lead to improved performance.
6. Use Excerpts Instead of Full Posts in Archives
WordPress generates archive pages for categories, tags, and author listings. By default, these pages display full posts, which can put a strain on your database. You can modify your theme to display excerpts instead.
Example: Displaying Excerpts in Archive Pages
php // Modify the archive template file (archive.php) in your theme // Replace the_content() with the_excerpt() to display excerpts
Switching to excerpts in archive pages can reduce the amount of database querying required, especially on pages with multiple posts.
7. Implement Lazy Loading for Images
Loading all images on a page at once can increase database queries and slow down page load times. Implementing lazy loading for images ensures that images are loaded only when they become visible in the user’s viewport.
Example: Adding Lazy Loading to Images
html <img src="image.jpg" alt="Description" loading="lazy">
By adding the loading=”lazy” attribute to your <img> tags, you instruct browsers to load images lazily, reducing the initial database load.
8. Use Content Delivery Networks (CDNs)
Content Delivery Networks are services that distribute your website’s assets, such as images and scripts, across multiple servers worldwide. This reduces the load on your WordPress server and speeds up content delivery.
Example: Configuring a CDN
php // Sign up for a CDN service like Cloudflare or StackPath // Follow the service's setup instructions and configure your CDN in WordPress
CDNs can significantly improve your site’s performance by serving assets from servers geographically closer to your users.
9. Optimize Database Queries
WordPress plugins and themes often execute database queries inefficiently. You can optimize these queries by reviewing your code and using caching techniques.
Example: Optimizing Database Queries
php // Bad query $result = $wpdb->get_results("SELECT * FROM wp_posts WHERE post_type = 'post'"); // Optimized query $result = $wpdb->get_results("SELECT post_title, post_date FROM wp_posts WHERE post_type = 'post'");
Review your theme and plugin code to ensure that database queries are as efficient as possible. Minimize the data retrieved from the database to reduce the server load.
10. Enable GZIP Compression
Enabling GZIP compression on your server can reduce the size of files transferred to users’ browsers. Smaller files load faster, improving site performance.
Example: Enabling GZIP Compression in Apache
apache <IfModule mod_deflate.c> # Compress HTML, CSS, JavaScript, Text, XML, and fonts AddOutputFilterByType DEFLATE application/javascript AddOutputFilterByType DEFLATE application/rss+xml AddOutputFilterByType DEFLATE application/vnd.ms-fontobject AddOutputFilterByType DEFLATE application/x-font AddOutputFilterByType DEFLATE application/x-font-opentype AddOutputFilterByType DEFLATE application/x-font-otf AddOutputFilterByType DEFLATE application/x-font-truetype AddOutputFilterByType DEFLATE application/x-font-ttf AddOutputFilterByType DEFLATE application/x-javascript AddOutputFilterByType DEFLATE application/xhtml+xml AddOutputFilterByType DEFLATE application/xml AddOutputFilterByType DEFLATE font/opentype AddOutputFilterByType DEFLATE font/otf AddOutputFilterByType DEFLATE font/ttf AddOutputFilterByType DEFLATE image/svg+xml AddOutputFilterByType DEFLATE image/x-icon AddOutputFilterByType DEFLATE text/css AddOutputFilterByType DEFLATE text/html AddOutputFilterByType DEFLATE text/javascript AddOutputFilterByType DEFLATE text/plain AddOutputFilterByType DEFLATE text/xml # Remove browser bugs (only needed for really old browsers) BrowserMatch ^Mozilla/4 gzip-only-text/html BrowserMatch ^Mozilla/4\.0[678] no-gzip BrowserMatch \bMSIE !no-gzip !gzip-only-text/html Header append Vary User-Agent </IfModule>
Implementing GZIP compression is a server-level optimization that can greatly reduce the amount of data transferred to users, improving load times.
Conclusion
Optimizing your WordPress database is essential for maintaining a fast and reliable website. By implementing the programming techniques discussed in this blog post, you can enhance user experience, reduce server load, and ensure the security and efficiency of your WordPress site. Keep in mind that database optimization is an ongoing process, so regularly monitor and maintain your database to ensure optimal performance.
Remember that every WordPress site is unique, so the specific optimizations needed may vary. Use the techniques mentioned here as a starting point and tailor them to your site’s requirements. With a well-optimized database, your WordPress site will be better equipped to handle increased traffic and deliver an exceptional user experience.
Optimize your WordPress database today, and watch your website thrive!
Table of Contents
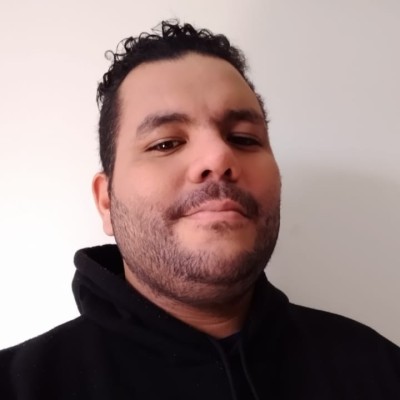
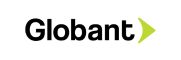